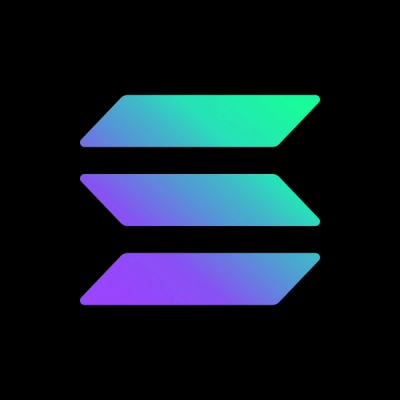
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
simplemattable
Advanced tools
A simplified, declarative table-library using @angular/material's MatTable
SimpleMatTable is an abstraction of MatTable from the @angular/material dependency. It allows you to quickly and in a typesafe way define simple tables. This is perfect if you just want to display some data in a table and do not need full control over the table HTML.
Instead of copy/pasting the HTML for each column, you can describe the columns in a declarative way via Typescript code.
This Dependency is for use with Angular Material Design only. As of the first version, it requires Angular Material 6.0 or above (see Version section for more information).
Start with a normal Angular App with Material Design installed.
Then install Simplemattable using
npm install simplemattable
Then import the SimplemattableModule into your AppModule. The following snippet shows the minimum AppModule you need to use Simplemattable:
import {BrowserModule} from '@angular/platform-browser';
import {NgModule} from '@angular/core';
import {SimplemattableModule} from 'simplemattable';
import {AppComponent} from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
SimplemattableModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Lets say you have a model that you want to display in a table, for example:
class TestData {
constructor(public key: string, public value: string) {
}
}
In your component (e.g. AppComponent) you can then define your columns like this:
columns = [
new TableColumn<TestData, 'key'>('Key', 'key'),
new TableColumn<TestData, 'value'>('Value', 'value')
];
The TableColumn class requires two generics. The first is the class of your model, the second is the property this column describes. The property-generic uses "keyof T", so your IDE will show an error at compile time if you enter a name that does not belong to a property of your model.
The Constructor of TableColumn requires you to specify the string that will be displayed in the table header and (again) the name of the property on the model. You already specified the property name in the generics, but since information about generics is not available at runtime, you need to specify it here again. Fortunately, the property in the constructor is also typesafe.
If you are looking for more customization options (e.g. align, width, different display values) for your columns, have a look at section TableColumn options.
After you defined your table columns, you can bind them to the html element using:
<smc-simplemattable [data]="testData" [columns]="columns" [paginator]="true" [filter]="true" [sorting]="true"></smc-simplemattable>
where testData is an array of your model and columns are the TableColumns you defined earlier.
Additionally, you can turn on a paginator, a filter and sorting. These are the standard MatTable Features that are also well described at https://material.angular.io/components/table/overview. The paginator, filter and sorting are optional. If omitted, the flags will default to false.
If you have a more complex model, for example
class ComplexTestData {
constructor(public id: number, public description: string, public data: TestData) {
}
}
and you want to display the description property as well as key and value of the data property, you can specify the colums like this:
columns = [
new TableColumn<ComplexTestData, 'description'>('Description', 'description'),
new TableColumn<ComplexTestData, 'data'>('Key', 'data', (data) => data.key),
new TableColumn<ComplexTestData, 'data'>('Value', 'data', (data) => data.value)
];
You can also use .withTransform instead of the 3rd constructor parameter (see next section TableColumn options).
Due to the data binding, the data as well as the table column definitions can be updated dynamically.
To update the table columns, simply change/add/remove the table columns in your table column array. For example, if you want to toggle the visiblility of the first column:
this.columns[0].visible = !this.columns[0].visible;
Simplemattable will recognize any changes to the properties of any of the supplied columns or to the column array.
Changing the data is a bit different. Performing the checks to recognize any changes on the fly would take up too much performance on large tables with complex models. Therefore, the default Angular check is used, which compares the array references via ===. This means that you have to change the array reference. If you have a table with the complex model described earlier, you could add a new entry using this:
this.testData.push(new ComplexTestData(42, 'New Entry', new TestData('key', 'value')));
this.testData = this.testData.slice(0);
Note that .slice(0) is one of the fastest, if not the fastest way to clone arrays. For more information, see this StackOverflow question.
If sorting is enabled, updating the columns will clear the current sorting selection. Changes in Data will not clear the sorting selection.
TableColumn has several optional parameters, allowing you to further customize the table. Some of these properties are functions. These functions always take the property of your model (e.g. the key property of TestData) as first parameter and the model object itself (e.g the instance of TestData) as second parameter. Often times, the first parameter will suffice, so you can leave out the second one. Use the second one only if you need to reference another property of your model object in the function.
checkedIn
on your model, you could do (checkedIn) => checkedIn ? 'check' : 'close'
for its column, which will either display a tick or a cross icon.ButtonType.BASIC
(mat-button), ButtonType.RAISED
(mat-raised-button) or ButtonType.ICON
(mat-icon-button).
The button will use the text and icon (or only the icon in case of ButtonType.ICON
) that would normally be displayed directly in the cell.
If specified, the onClick function will be executed on a click event.'primary'
, 'warn'
or 'accent'
.
If you leave the button color empty, the standard white/transparent background (depending on button type) will be used.The Sourcecode is in a private repository for now. If anyone is interested in contributing, email me and I will transfer this library to a public github or gitlab repository. For my email address, see the authors section.
There will be new versions when new features are added or a new Angular version releases.
History (Version in parenthesis is required Angular Version):
Henning Wobken (henning.wobken@simplex24.de)
This project is licensed under the MIT License
FAQs
A simplified, declarative table-library using @angular/material's MatTable with form capabilities for adding/editing/deleting data
The npm package simplemattable receives a total of 16 weekly downloads. As such, simplemattable popularity was classified as not popular.
We found that simplemattable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.