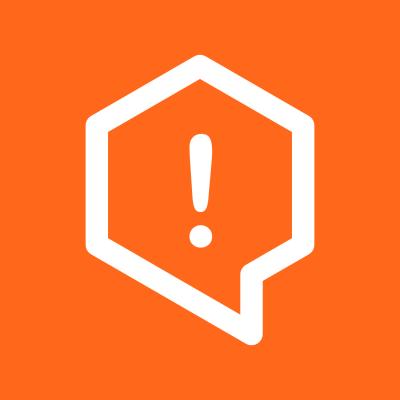
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
simplytyped
Advanced tools
Yet another typing library. This differs by aiming to be less experimental than others, driven by industry use cases.
Many of the exposed types are a very thin layer above built in functionality. The goal is to provide all of the building blocks necessary to make concise, yet complex types.
npm install --save-dev simplytyped
If - And - Or - Not - Xor - Nand
IsAny - IsArray - IsBoolean - IsFunction - IsNever - IsNumber - IsObject - IsType
Keys - ObjectType - CombineObjects - Intersect - SharedKeys - DiffKeys - AllKeys - Omit - Merge - Overwrite - DeepPartial - DeepReadonly - Optional - GetKey - AllRequired - Required - TaggedObject
Tuple - UnionizeTuple - IntersectTuple - Length
Diff - StringEqual - DropString
IsZero - IsOne - NumberToString - Next - Prev - Add - Sub - NumberEqual
Predicate - ConstructorFunction - AnyFunc - Readonly - isKeyOf - objectKeys
Nullable - NoInfer - Unknown - Nominal
Some (somewhat common) implementations of conditional type logic
type x = If<True, string, number> // => string
type y = If<False, string, number> // => number
type x = If<And<True, True>, string, number> // => string
type y = If<And<True, False>, string, number> // => number
...
type x = If<Or<True, False>, string, number> // => string
type y = If<Or<False, False>, string, number> // => number
...
type x = Not<True> // => False
type y = Not<False> // => True
type x = Xor<True, False> // => True
type y = Xor<True, True> // => False
...
type x = Nand<True, True> // => False
type y = Nand<False, True> // => True
type x = IsAny<any> // => True
type y = IsAny<'hey'> // => False
type x = IsArray<any[]> // => True
type y = IsArray<number> // => False
type x = IsBoolean<false> // => True
type y = IsBoolean<3> // => False
type x = IsFunction<(() => string)> // => True
type y = IsFunction<'not a function'> // => False
Returns true if type is never
, otherwise returns false.
type x = IsNever<'hi'> // => False
type y = IsNever<never> // => True
type x = IsNumber<3> // => True
type y = IsNumber<false> // => False
type x = IsObject<{a: number, b: string}> // => True
type y = IsObject<string> // => False
Given a base type and a value, check to see if value matches the base type. Useful for checking if something is an instance of a class.
class Thing { x: string };
type x = IsType<Thing, { x: string }> // => True
type y = IsType<Thing, { y: number }> // => False
type obj1 = { w: string, x: string, y: number }
type obj2 = { y: string, z: number }
No different than keyof
, but can look a bit nicer when nesting many types deep
type x = keyof obj1 // => 'w' | 'x' | 'y'
type y = Keys<obj1> // => 'w' | 'x' | 'y'
On its own, not that interesting.
Takes an object and makes it an object type.
Is useful when combined with &
intersection types (as seen next).
type x = ObjectType<obj1> // => { w: string, x: string, y: number }
Takes the intersection between two objects, and flattens them. This can make extremely complex types look much nicer.
type x = obj1 & obj2 // => { w: string, x: string, y: number } & { y: string, z: number }
type y = CombineObjects<obj1, obj2> // => { w: string, x: string, y: string & number, z: number }
Returns only the shared properties between two objects. All shared properties must be the same type.
type x = Intersect<obj1, { x: string }> // => { x: string }
Gets all of the keys that are shared between two objects (as in keys in common).
type x = SharedKeys<obj1, obj2> // => 'y'
Gets all of the keys that are different from obj1 to obj2.
type x = DiffKeys<obj1, obj2> // => 'w' | 'x'
type y = DiffKeys<obj2, obj1> // => 'z'
Gets all keys between two objects.
type x = AllKeys<obj1, obj2> // => 'w' | 'x' | 'y' | 'z'
Gives back an object with listed keys removed.
type x = Omit<obj1, 'w' | 'x'> // => { y: number }
Much like _.merge
in javascript, this returns an object with all keys present between both objects, but conflicts resolved by rightmost object.
type x = Merge<obj1, obj2> // => { w: string, x: string, y: string, z: number }
Can change the types of properties on an object.
This is similar to Merge
, except that it will not add previously non-existent properties to the object.
type a = Overwrite<obj1, obj2> // => { w: string, x: string, y: string }
type b = Overwrite<obj2, obj1> // => { y: number, z: number }
Uses Partial
to make every parameter of an object optional (| undefined
).
type x = DeepPartial<obj1> // => { w?: string, x?: string, y?: number }
Uses Readonly
to make every parameter of an object readonly
type x = DeepReadonly<obj1> // => { w: readonly string, x: readonly string, y: readonly number }
Makes certain properties on an object optional.
type x = Optional<obj1, 'w' | 'x'> // => { w?: string, x?: string, y: number }
Gets the value of specified property on any object without compile time error (Property 'b' does not exist on type '{ a: string; }'.
) and the like.
Returns never
if the key is not on the object.
I suggest using If<HasKey...
first to handle validity of the object first.
type x = GetKey<{ a: string }, 'a'> // => string
type y = GetKey<{ a: string }, 'b'> // => never
Builds the type of a constructor function for a particular object.
type c = ConstructorFor<obj1> // => new (...args: any[]) => { w: string, x: string, z: number }
Makes all fields of an object "required". This means not Nullable and not optional.
type x = { a?: string, b: number | undefined };
type o = AllRequired<x>; // => { a: string, b: number }
Makes certain fields of an object "required"
type x = { a?: string, b: number | undefined };
type o = Required<x, 'a'>; // => { a: string, b: number | undefined }
Creates an object with each entry being tagged by the key defining that entry.
const obj = {
a: { merp: 'hi' },
b: { merp: 'there' },
c: { merp: 'friend' },
};
const got = taggedObject(obj, 'name');
/*
got = {
a: { name: 'a', merp: 'hi' },
b: { name: 'b', merp: 'there' },
c: { name: 'c', merp: 'friend' },
};
*/
A few utility functions that generically work in any context, with any type.
Makes a type nullable (null | undefined).
type x = Nullable<string>; // => string | null | undefined
Prevents typescript from being able to infer a generic type. Useful when trying to get a function to infer based on one argument of a function, but not another.
function doStuff<T>(x: T, y: NoInfer<T>): T { return x; }
function inferAll<T>(x: T, y: T): T { return x; }
doStuff('hi', 'there') // => compile error
inferAll('hi', 'there') // => typeof T === 'string'
A type that has no properties and cannot be passed into functions.
This is particularly useful on the boundaries of an app where you may not know the type of a variable.
For instance JSON.parse
could return an Unknown
and would require validation and / or a type assertion to make it a useful type.
declare let x: Unknown;
x = 'hi'; // valid operation
function doStuff(a: number) {}
doStuff(x); // invalid operation
x.thing // invalid operation
x() // invalid operation
This creates a type that, while it shares all of the properties with its parent type, cannot be set to another type without containing the same tag. This is useful in the case where you have a string that is an id, but you don't want to be able to set just any string to this id; only a string tagged as being an id.
type Id = Nominal<string, 'id'>; // => string
declare let x: Id;
x = 'hi'; // invalid operation;
x = 'hi' as Nominal<string, 'id'>; // valid operation
x = 'hi' as Id; // valid operation
A tuple can be defined in two ways: [number, string]
which as of Typescript 2.7 has an enforced length type parameter: [number, string]['length'] === 2
or using this library's Tuple<any>
which can be extended with any length of tuple: function doStuff<T extends Tuple<any>>(x: T) {}
.
function doStuff<T extends Tuple<any>>(x: T) {}
doStuff(['hi', 'there']); // => doStuff(x: ['hi', 'there']): void
Returns elements within a tuple as a union.
type x = UnionizeTuple<[number, string]> // => number | string
Returns elements of a tuple intersected with each other. Note: only works for tuples up to length 10
type x = IntersectTuple<[{a: 'hi'}, {b: 'there'}]>; // => {a: 'hi'} & {b: 'there'}
Gets the length of either a built-in tuple, or a Vector. This will only work after Typescript 2.7 is released.
type x = Length<['hey', 'there']> // => 2
Get the differences between two unions of strings.
type x = Diff<'hi' | 'there', 'hi' | 'friend'> // => 'there'
Returns true if all elements in two unions of strings are equal.
type x = StringEqual<'hi' | 'there', 'hi'> // => False
type y = StringEqual<'hi' | 'there', 'there' | 'hi'> // => True
Can remove a string from a union of strings
type x = DropString<'hi' | 'there', 'hi'> // => 'there'
Supports numbers from [0, 63]. More slows down the compiler to a crawl right now.
Returns true if the number is equal to zero.
type x = IsZero<1> // => False
type y = IsZero<0> // => True
Returns true if the number is equal to one.
type x = IsOne<0> // => False
type y = IsOne<1> // => True
Returns the string type for a given number
type x = NumberToString<0> // => '0'
type y = NumberToString<1> // => '1'
Returns the number + 1.
type x = Next<0> // => 1
type y = Next<22> // => 23
Returns the number - 1.
type x = Prev<0> // => -1
type y = Prev<23> // => 22
Adds two numbers together.
type x = Add<22, 8> // => 30
Subtracts the second from the first.
type x = Sub<22, 8> // => 14
Returns True
if the numbers are equivalent
type x = NumberEqual<0, 0> // => True
type y = NumberEqual<22, 21> // => False
This is a function that takes some args and returns a boolean
type x = Predicate // => (...args: any[]) => boolean
const isThing: Predicate = (x: Thing) => x instanceof Thing;
This represents the constructor for a particular object.
class Thing { constructor(public x: string) {}}
type x = ConstructorFunction<Thing>
declare const construct: x;
const y = new construct(); // => y instanceof Thing
Concisely and cleanly define an arbitrary function. Useful when designing many api's that don't care what function they take in, they just need to know what it returns.
type got = AnyFunc; // => (...args: any[]) => any;
type got2 = AnyFunc<number>; // => (...args: any[]) => number;
This takes a runtime object and makes its properties readonly. Useful for declare object literals, but using inferred types.
const config = Readonly({
url: 'https://example.com',
password: 'immasecurepassword',
}); // => { url: readonly string, password: readonly string }
Type guard returning true if k
is a key in obj
.
const obj = { a: '' };
const k = 'a';
if (isKeyOf(obj, k)) {
obj[k] = 'hi'; // typeof k === 'a'
} else {
throw new Error('oops'); // typeof k === string
}
Same as Object.keys
except that the returned type is an array of keys of the object.
Note that for the same reason that Object.keys
does not do this natively, this method is not safe for objects on the perimeter of your code (user input, read in files, network requests etc.).
const obj = { a: '', b: 22 };
const keys = objectKeys(obj); // Array<'a' | 'b'>
FAQs
yet another Typescript type library for advanced types
The npm package simplytyped receives a total of 7,724 weekly downloads. As such, simplytyped popularity was classified as popular.
We found that simplytyped demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.