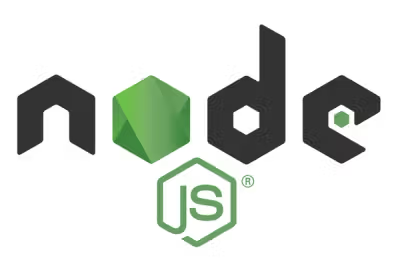
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
sjs-base-model
Advanced tools
BaseModel helps translate data to models
const apiData = {
make: 'Tesla',
model: 'Model S',
YeAr: 2014,
feature: {
abs: true,
airbags: true
},
colors: [{id: 'red', name: 'Red'}, {id: 'white', name: 'White'}]
}
const carModel = new CarModel(data);
This is how you should extend sjs-base-model
import {BaseModel} from 'sjs-base-model';
export class CarModel extends BaseModel {
make = '';
model = '';
year = null;
feature = FeatureModel;
colors = [ColorModel];
constructor(data = {}) {
super();
if (data) {
this.update(data);
}
}
update(data) {
super.update(data);
this.year = data.YeAr;
}
}
Model Explained
import {BaseModel} from 'sjs-base-model';
export class CarModel extends BaseModel {
// The class properties must match the data properties being passed in. Otherwise they will be ignored
make = '';
model = '';
year = null;
// If data passed in is an object then a FeatureModel will be created
// else the property will be set to null
feature = FeatureModel;
// If the data passed is an array then it will create a ColorModel for each item
// else the property will be set to an empty array
colors = [ColorModel];
constructor(data = {}) {
super();
if (data) {
this.update(data);
}
}
update(data) {
super.update(data);
// If the data doesn't match the property name
// You can set the value(s) manually after the update super method has been called.
this.year = data.YeAr;
}
}
Example how to use the update
method which will only change the property value(s) that were passed in
carModel.update({year: 2015, feature: {abs: true}});
You will need to do as any
when assigning the function model to the type of model so the compiler doesn't complain. Notice FeatureModel as any;
and [ColorModel as any];
import {BaseModel} from 'sjs-base-model';
export class CarModel extends BaseModel {
make: string = '';
model: string = '';
year: number = null;
feature: FeatureModel = FeatureModel as any;
colors: ColorModel[] = [ColorModel as any];
constructor(data: any = {}) {
super();
if (data) {
this.update(data);
}
}
update(data: any): void {
super.update(data);
this.year = data.YeAr;
}
}
I like to keep my data consistent in my applications. So I like everything to be camelCase
. It's hard when dealing with different data api's. Each one can return a differnt case type (kebab-case
, snake_case
, PascalCase
, camelCase
, UPPER_CASE
and this one @propertyName
).
What you can do is create a class called PropertyNormalizerModel that extends sjs-base-mode
that normalizes the data coming in. Then all your other models extends PropertyNormalizerModel.
See example code for ideas.
FAQs
BaseModel helps translate data to models
The npm package sjs-base-model receives a total of 2,053 weekly downloads. As such, sjs-base-model popularity was classified as popular.
We found that sjs-base-model demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.