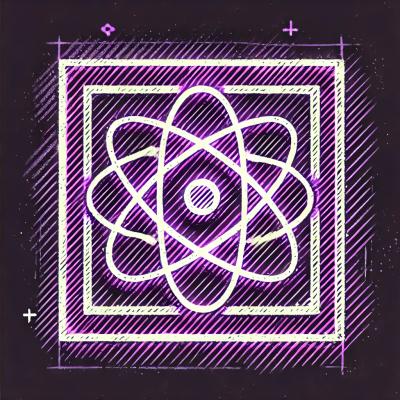
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
sjs-base-model
Advanced tools
BaseModel helps translate data to models
With Node.js:
$ npm install sjs-base-model
const apiData = {
make: 'Tesla',
model: 'Model S',
YeAr: 2014,
feature: {
abs: true,
airbags: true
},
colors: [{id: 'red', name: 'Red'}, {id: 'white', name: 'White'}]
}
const carModel = new CarModel(apiData);
This is how you should extend sjs-base-model
import {BaseModel} from 'sjs-base-model';
export class CarModel extends BaseModel {
make = '';
model = '';
year = null;
feature = FeatureModel;
colors = [ColorModel];
constructor(data = {}) {
super();
this.update(data);
}
update(data) {
super.update(data);
this.year = data.YeAr;
}
}
Model Explained
import {BaseModel} from 'sjs-base-model';
export class CarModel extends BaseModel {
// The class properties must match the data properties being passed in. Otherwise they will be ignored
make = '';
model = '';
year = null;
// If data passed in is an object then a FeatureModel will be created
// else the property will be set to null
feature = FeatureModel;
// If the data passed is an array then it will create a ColorModel for each item
// else the property will be set to an empty array
colors = [ColorModel];
constructor(data = {}) {
super();
this.update(data);
}
update(data) {
super.update(data);
// If the data doesn't match the property name
// You can set the value(s) manually after the update super method has been called.
this.year = data.YeAr;
}
}
Example how to use the update
method which will only change the property value(s) that were passed in
carModel.update({year: 2015, feature: {abs: true}});
Converts the BaseModel data into a JSON object and deletes the sjsId property.
const json = carModel.toJSON();
Converts a BaseModel to a JSON string,
const jsonStr = carModel.toJSONString();
Converts the string json data into an Object and calls the update method with the converted Object.
const str = '{"make":"Tesla","model":"Model S","year":2014}'
const carModel = new CarModel();
carModel.fromJSON(str);
Creates a clone/copy of the BaseModel.
const clone = carModel.clone();
You will need to do as any
when assigning the function model to the type of model so the compiler doesn't complain. Notice FeatureModel as any;
and [ColorModel as any];
import {BaseModel} from 'sjs-base-model';
export class CarModel extends BaseModel {
make: string = '';
model: string = '';
year: number = null;
feature: FeatureModel = FeatureModel as any;
colors: ColorModel[] = [ColorModel as any];
constructor(data: any = {}) {
super();
this.update(data);
}
update(data: any): void {
super.update(data);
this.year = data.YeAr;
}
}
I like to keep my data consistent in my applications. So I like everything to be camelCase
. It's hard when dealing with different data api's. Each one can return a differnt case type (kebab-case
, snake_case
, PascalCase
, camelCase
, UPPER_CASE
and this one @propertyName
).
What you can do is create a class called PropertyNormalizerModel that extends sjs-base-mode
that normalizes the data coming in. Then all your other models extends PropertyNormalizerModel.
See example code for ideas.
2018-04-20 v1.4.0 Add the ability to convert property values to ConversionTypeEnum.Float, ConversionTypeEnum.Number or ConversionTypeEnum.Boolean with IConvertOption.
2018-04-15 v1.3.2 Make the clone method a Generic TypeScript type. model.clone<SomeModel>();
2018-04-10 v1.3.1 Update to previous version (v1.3.0) to allow other types not just objects. Now will add number, string, etc.
2018-03-29 v1.3.0 If the default property value is an array and a object is passed for that property. It will put that object into an array.
2018-03-20 v1.2.0 Handle null being passed in and console.error message. Remove null check condition from constructor in code examples.
2018-03-05 v1.1.0 Fixed issue: If an array of data passed in with no BaseModel assigned. It would set it as an empty array. Now it will assign the raw array data correctly.
2018-02-24 v1.0.0
FAQs
BaseModel helps translate data to models
The npm package sjs-base-model receives a total of 189 weekly downloads. As such, sjs-base-model popularity was classified as not popular.
We found that sjs-base-model demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.