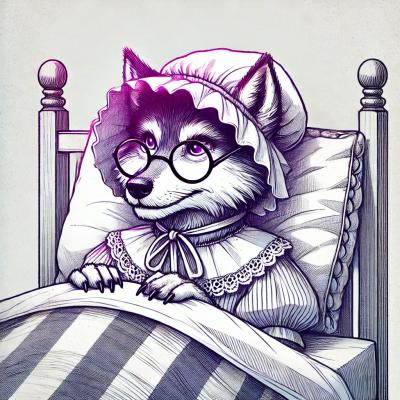
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Super fast simple k-means and k-means++ clustering for unidimiensional and multidimensional data. Works in node and browser
The skmeans npm package is a simple and efficient implementation of the k-means clustering algorithm. It is used for partitioning a dataset into k distinct, non-overlapping subsets (clusters). The package is designed to be lightweight and easy to use, making it suitable for quick clustering tasks in JavaScript applications.
Basic k-means clustering
This feature allows you to perform basic k-means clustering on a dataset. The code sample demonstrates how to cluster a simple 2D dataset into 2 clusters.
const skmeans = require('skmeans');
const data = [[1, 2], [2, 3], [3, 4], [8, 9], [9, 10], [10, 11]];
const k = 2;
const result = skmeans(data, k);
console.log(result);
Custom initialization
This feature allows you to specify custom initial centroids for the k-means algorithm. The code sample demonstrates how to initialize the centroids manually.
const skmeans = require('skmeans');
const data = [[1, 2], [2, 3], [3, 4], [8, 9], [9, 10], [10, 11]];
const k = 2;
const initialCentroids = [[1, 2], [8, 9]];
const result = skmeans(data, k, 'kmpp', initialCentroids);
console.log(result);
Weighted k-means clustering
This feature allows you to perform weighted k-means clustering, where each data point can have a different weight. The code sample demonstrates how to apply weights to the data points.
const skmeans = require('skmeans');
const data = [[1, 2], [2, 3], [3, 4], [8, 9], [9, 10], [10, 11]];
const weights = [1, 1, 1, 2, 2, 2];
const k = 2;
const result = skmeans(data, k, 'kmpp', null, weights);
console.log(result);
kmeans-js is another JavaScript implementation of the k-means clustering algorithm. It offers similar functionality to skmeans but includes additional features like support for different distance metrics and more advanced initialization methods. It is slightly more complex but provides more flexibility for advanced users.
ml-kmeans is part of the machine learning library 'ml' and provides a robust implementation of the k-means algorithm. It is designed to work seamlessly with other machine learning tools in the 'ml' ecosystem, making it a good choice for more comprehensive machine learning projects. It offers more advanced options and better integration with other machine learning algorithms compared to skmeans.
simple-statistics is a library that provides a wide range of statistical tools, including k-means clustering. While it is not solely focused on k-means, it offers a comprehensive set of statistical functions that can be useful for data analysis. It is a good choice if you need a broader set of statistical tools in addition to k-means clustering.
Super fast simple k-means and k-means++ implementation for unidimiensional and multidimensional data. Works on nodejs and browser.
npm install skmeans
const skmeans = require("skmeans");
var data = [1,12,13,4,25,21,22,3,14,5,11,2,23,24,15];
var res = skmeans(data,3);
<!doctype html>
<html>
<head>
<script src="skmeans.js"></script>
</head>
<body>
<script>
var data = [1,12,13,4,25,21,22,3,14,5,11,2,23,24,15];
var res = skmeans(data,3);
console.log(res);
</script>
</body>
</html>
{
it: 2,
k: 3,
idxs: [ 2, 0, 0, 2, 1, 1, 1, 2, 0, 2, 0, 2, 1, 1, 0 ],
centroids: [ 13, 23, 3 ]
}
Calculates unidimiensional and multidimensional k-means clustering on data. Parameters are:
The function will return an object with the following data:
// k-means with 3 clusters. Random initialization
var res = skmeans(data,3);
// k-means with 3 clusters. Initial centroids provided
var res = skmeans(data,3,[1,5,9]);
// k-means with 3 clusters. k-means++ cluster initialization
var res = skmeans(data,3,"kmpp");
// k-means with 3 clusters. Random initialization. 10 max iterations
var res = skmeans(data,3,null,10);
// k-means with 3 clusters. Custom distance function
var res = skmeans(data,3,null,null,(x1,x2)=>Math.abs(x1-x2));
// Test new point
var res = skmeans(data,3,null,10);
res.test(6);
// Test new point with custom distance
var res = skmeans(data,3,null,10);
res.test(6,(x1,x2)=>Math.abs(x1-x2));
FAQs
Super fast simple k-means and k-means++ clustering for unidimiensional and multidimensional data. Works in node and browser
The npm package skmeans receives a total of 253,723 weekly downloads. As such, skmeans popularity was classified as popular.
We found that skmeans demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.