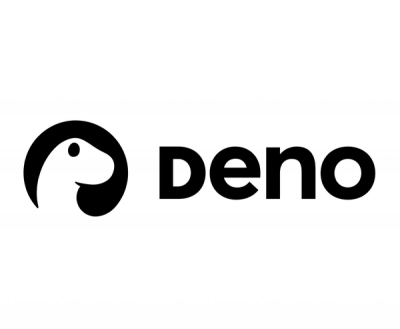
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
skyflow-nodejs-sdk
Advanced tools
Node.js API Client for the [Skyflow Platform API].
Requires Node.js version 6.9.0 or higher.
npm install skyflow-nodejs-sdk
You can view the entire JsDocs for this project here: https://dev.skyflow.com/skyflow-sdk-nodejs/jsdocs/
All usage of this SDK begins with the creation of a client, the client handles the authentication and communication with the Skyflow API. To create a client, you need to provide it with your Skyflow Domain and an API token. To obtain those, see Getting Started With the Skyflow APIs.
To get started create a skyflow client in one of the following ways
import {connect} from 'skyflow-nodejs-sdk';
const client = connect(orgid, <skyflow username>, <skyflow password>, <app id>, <app secret> , options)
//options are optional parameters
Options object can include
{
accessToken : 'your access token', // your access jwt. Defaults to generating a new token from given credentials
browser : true, // if you are using this client from front end, Defaults to false
prodApp : false //if this is a production application. Default to false
}
All interactions with the [Skyflow Platform API] is done through client methods. Some examples are below, but for a full list of methods please refer to the JsDoc page for the [Client].
client.getAccessToken()
.then(res => {
//returns access token if credentials are valid
})
.catch(err => {
})
client.insertRecord('<your vault id',
[
{
"name": "<field name>",
"value": "<field value>"
}
]
)
.then(res => {
console.log(res) // returns the token id and tokens of each column values
})
.catch(err => console.log(err.data.error))
To get the record values back pass in the id token of respective rows,
client.getRecord('<vault id>', '<token>')
.then(res => {
console.log(res)
})
.catch(err => console.log(err));
This api deletes the record permanently from the vault.
client.deleteRecord('<vault id>', '<token>')
.then(res => {
console.log(res) //returns back the id if operation is successful
})
.catch(err => console.log(err));
This api can be used to update some or all the ros of the record.
let recordFields = [
{
name : 'field name',
value : 'field new value'
}
]
client.updateRecord('<vault id>', '<token>', recordFields)
.then(res => {
console.log(res) //updated token values
})
.catch(err => console.log(err));
This can be used to add multiple records at once.
let records = {
records : [
{
fields : [
{
name : 'field name',
value : 'field value'
}
]
}
]
}
client.insertBulkRecord('<vault id>', records)
.then(res => {
console.log(res)
})
.catch(err => console.log(err));
}, 3000);
FAQs
Node js client for skyflow API's
The npm package skyflow-nodejs-sdk receives a total of 0 weekly downloads. As such, skyflow-nodejs-sdk popularity was classified as not popular.
We found that skyflow-nodejs-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.