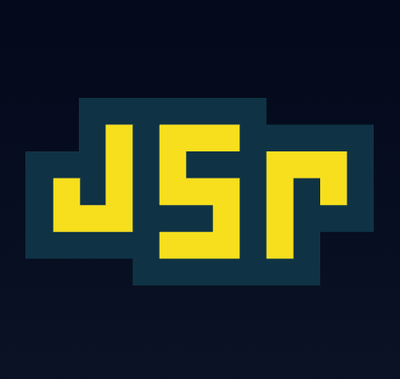
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
A completely customizable framework for building rich text editors.
Slate is a completely customizable framework for building rich text editors. It provides a set of tools and components to create complex text editing experiences with ease.
Creating a Basic Editor
This code initializes a basic Slate editor instance. The `Editor` class is the core of Slate, and it provides the foundational methods and properties needed to build a rich text editor.
const { Editor } = require('slate');
const editor = new Editor();
console.log(editor);
Handling User Input
This code demonstrates how to handle user input by inserting text into the editor. The `Transforms` module provides various methods to manipulate the editor's content.
const { Editor, Transforms } = require('slate');
const editor = new Editor();
Transforms.insertText(editor, 'Hello, Slate!');
console.log(editor.children);
Custom Elements and Marks
This code shows how to define custom elements and marks. In this example, a custom text node with a bold mark is created and added to the editor's content.
const { Editor, Text } = require('slate');
const editor = new Editor();
const customText = { text: 'Custom Text', bold: true };
editor.children = [{ type: 'paragraph', children: [customText] }];
console.log(editor.children);
Plugins
This code demonstrates how to extend the editor's functionality using plugins. The `withCustomPlugin` function wraps the editor and overrides the `insertText` method to add custom behavior.
const { Editor } = require('slate');
const withCustomPlugin = editor => {
const { insertText } = editor;
editor.insertText = text => {
console.log('Inserting text:', text);
insertText(text);
};
return editor;
};
const editor = withCustomPlugin(new Editor());
editor.insertText('Hello, Plugins!');
Draft.js is a framework for building rich text editors in React. It provides a set of immutable models and React components to create complex text editing experiences. Compared to Slate, Draft.js is more opinionated and less flexible but offers a more straightforward API for common use cases.
ProseMirror is a toolkit for building rich text editors with a focus on extensibility and performance. It provides a highly customizable and modular architecture, similar to Slate, but with a steeper learning curve. ProseMirror is known for its fine-grained control over document structure and editing behavior.
Quill is a modern WYSIWYG editor built for compatibility and extensibility. It offers a simple API and a rich set of features out of the box, making it easy to get started. Compared to Slate, Quill is less customizable but provides a more polished and user-friendly experience for basic text editing needs.
This package contains the core logic of Slate. Feel free to poke around to learn more!
Note: A number of source files contain extracted types for Interfaces
or Transforms
. This is done currently to enable custom type extensions as found in packages/src/interfaces/custom-types.ts
.
FAQs
A completely customizable framework for building rich text editors.
The npm package slate receives a total of 781,112 weekly downloads. As such, slate popularity was classified as popular.
We found that slate demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.