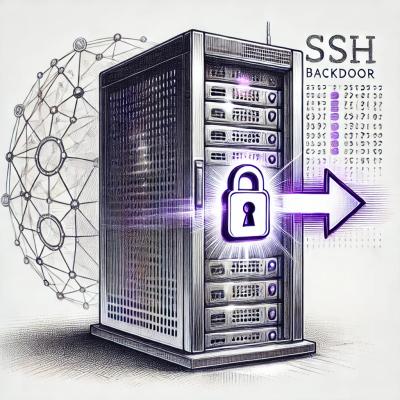
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
sleep-promise
Advanced tools
The 'sleep-promise' npm package provides a simple way to pause the execution of asynchronous code for a specified duration. It returns a promise that resolves after the given time, making it useful for delaying operations in a non-blocking manner.
Basic Sleep
This feature allows you to pause the execution of your code for a specified duration. In this example, the code pauses for 2 seconds between logging 'Start' and 'End'.
const sleep = require('sleep-promise');
async function example() {
console.log('Start');
await sleep(2000); // Sleep for 2 seconds
console.log('End');
}
example();
Sleep with Error Handling
This feature demonstrates how to handle errors that might occur during the sleep operation. Although 'sleep-promise' itself does not throw errors, this pattern is useful for more complex scenarios where other asynchronous operations might fail.
const sleep = require('sleep-promise');
async function example() {
try {
console.log('Start');
await sleep(2000); // Sleep for 2 seconds
console.log('End');
} catch (error) {
console.error('An error occurred:', error);
}
}
example();
The 'delay' package provides similar functionality to 'sleep-promise' by allowing you to delay the execution of asynchronous code. It also offers additional features like creating cancellable delays and delay loops. Compared to 'sleep-promise', 'delay' is more feature-rich and versatile.
The 'await-sleep' package is another alternative that provides a simple way to pause execution in async functions. It is very similar to 'sleep-promise' in terms of functionality but has a smaller footprint and fewer additional features.
sleep-promise resolves a promise after a specified delay.
npm install sleep-promise
import sleep from 'sleep-promise';
(async () => {
await sleep(2000);
console.log('2 seconds later …');
})();
var sleep = require('sleep-promise');
sleep(2000).then(function() {
console.log('2 seconds later …');
});
import sleep from 'sleep-promise';
let trace = value => {
console.log(value);
return value;
};
sleep(2000)
.then(() => 'hello')
.then(trace)
.then(sleep(1000))
.then(value => value + ' world')
.then(trace)
.then(sleep(500))
.then(value => value + '!')
.then(trace);
// [2 seconds sleep]
// hello
// [1 second sleep]
// hello world
// [500 ms sleep]
// hello world!
import sinon from 'sinon';
import sleep from 'sleep-promise';
const clock = sinon.useFakeTimers();
(async () => {
// 2 seconds faked by sinon
const sleepPromise = sleep(2000);
clock.tick(2000);
await sleepPromise;
console.log('instant');
// Caches global setTimeout before sinon replaced it
const sleepPromise2 = sleep(2000, { useCachedSetTimeout: true });
clock.tick(2000);
await sleepPromise2;
console.log('2 seconds later');
})();
FAQs
Resolves a promise after a specified delay
The npm package sleep-promise receives a total of 180,862 weekly downloads. As such, sleep-promise popularity was classified as popular.
We found that sleep-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.