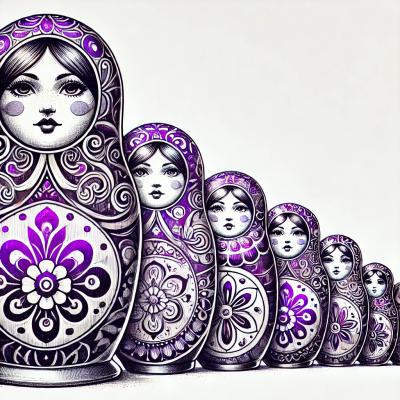
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
smartlook-client
Advanced tools
Imports and initializes Smartlook recorder into a page.
Installation
npm install smartlook-client --save
or
yarn add smartlook-client
Import
import smartlookClient from 'smartlook-client'
or
var smartlookClient = require('smartlook-client')
API
init(string key)
init(string key, params?: { region?: 'eu' | 'us', version?: 'legacy' | 'nextgen' })
track(string eventName, object<key:value> props)
identify(integer | string userId, object<key:value> props)
anonymize()
disable()
consentForms(string | false consent)
consentIP(string | false consent)
consentAPI(string | false consent)
getData(function callback)
restart()
pause()
resume()
error(string | Error error)
navigation(string locationOrPath)
properties(object<key:value> properties)
initialized()
playUrl
sessionId
visitorId
recordId
key
version
Example usage in React
Usage in other libraries is similar.
import React, { Component } from 'react'
import smartlookClient from 'smartlook-client'
class App extends Component {
handleIdentify = () => {
smartlookClient.identify(12345, {
name: 'John Doe',
email: 'email@domain.com',
// other custom properties
})
}
handleTrack = () => {
smartlookClient.track('transaction', {
value: 150,
currency: 'usd',
product: 'Product Description',
// other custom properties
})
}
handlePause = () => {
smartlookClient.pause()
}
render() {
return (
<div>
<button onClick={this.handleIdentify}>Identify visitor</button>
<button onClick={this.handleTrack}>Track event</button>
<button onClick={this.handlePause}>Pause recording</button>
</div>
)
}
componentDidMount() {
smartlookClient.init('43bc84d9a8406exxxxxxxxxb5601f5bbf8d2ed')
}
}
export default App
For more info visit https://www.smartlook.com/docs/api.html
FAQs
Official Smartlook client for easy frontend integration.
The npm package smartlook-client receives a total of 26,020 weekly downloads. As such, smartlook-client popularity was classified as popular.
We found that smartlook-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.