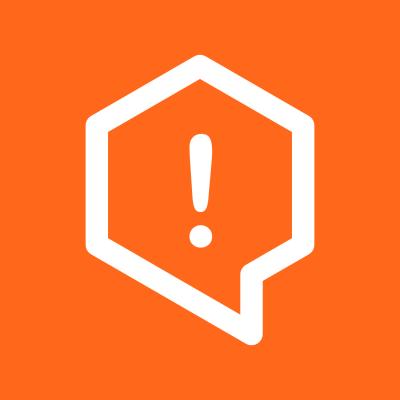
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
smartsheet
Advanced tools
This is an SDK to simplify connecting to the Smartsheet API from Node.js applications.
Please note that this SDK is beta and may change significantly in the future.
To install this SDK, simply run the following command in a terminal window:
npm install smartsheet
The Smartsheet API documentation can be found here.
See below for code examples that show how to call the various methods in this SDK.
If you would like to contribute a change to the SDK, please fork a branch and then submit a pull request. More info here.
##Support If you have any questions or issues with this SDK please post on StackOverflow using the tag "smartsheet-api" or contact us directly at api@smartsheet.com.
All APIs are exposed inside the root module that is created using the following:
var client = require('smartsheet');
var smartsheet = client.createClient({accessToken:'ACCESSTOKEN'});
The smartsheet variable now contains access to all of the APIs documented here. The following code examples show how to call various methods using this SDK.
###Favorite API methods
####listFavorites back to TOC #####Example using promises
smartsheet.favorites.listFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.listFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addItemsToFavorites back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addItemsToFavorites(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addItemsToFavorites(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addSheetToFavorites back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addSheetToFavorites(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addSheetToFavorites(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addFolderToFavorites back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addFolderToFavorites(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addFolderToFavorites(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addReportToFavorites back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addReportToFavorites(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addReportToFavorites(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addTemplateToFavorites back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addTemplateToFavorites(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addTemplateToFavorites(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addWorkspaceToFavorites back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addWorkspaceToFavorites(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.favorites.addWorkspaceToFavorites(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeSheetFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeSheetFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeSheetFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeFolderFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeFolderFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeFolderFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeReportFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeReportFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeReportFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeTemplateFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeTemplateFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeTemplateFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeWorkspaceFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeWorkspaceFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeWorkspaceFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeSheetsFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeSheetsFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeSheetsFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeFoldersFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeFoldersFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeFoldersFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeReportsFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeReportsFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeReportsFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeTemplatesFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeTemplatesFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeTemplatesFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeWorkspacesFromFavorites back to TOC #####Example using promises
smartsheet.favorites.removeWorkspacesFromFavorites({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.favorites.removeWorkspacesFromFavorites({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Folder API methods
####getFolder back to TOC #####Example using promises
smartsheet.folders.getFolder({id: foldersId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.folders.getFolder({id: foldersId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listChildFolders back to TOC #####Example using promises
smartsheet.folders.listChildFolders({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.folders.listChildFolders({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createChildFolder back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.folders.createChildFolder(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.folders.createChildFolder(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateFolder back to TOC #####Example using promises
var options = {
id: foldersId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.folders.updateFolder(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: foldersId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.folders.updateFolder(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteFolder back to TOC #####Example using promises
var options = {
id: foldersId
}
smartsheet.folders.deleteFolder(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: foldersId
}
smartsheet.folders.deleteFolder(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Group API methods
####listGroups back to TOC #####Example using promises
smartsheet.groups.listGroups({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.groups.listGroups({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getGroup back to TOC #####Example using promises
smartsheet.groups.getGroup({id: groupsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.groups.getGroup({id: groupsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createGroup back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.groups.createGroup(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.groups.createGroup(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addGroupMembers back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.groups.addGroupMembers(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.groups.addGroupMembers(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateGroup back to TOC #####Example using promises
var options = {
id: groupsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.groups.updateGroup(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: groupsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.groups.updateGroup(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteGroup back to TOC #####Example using promises
var options = {
id: groupsId
}
smartsheet.groups.deleteGroup(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: groupsId
}
smartsheet.groups.deleteGroup(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeGroupMember back to TOC #####Example using promises
smartsheet.groups.removeGroupMember({id: groupsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.groups.removeGroupMember({id: groupsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Home API methods
####listContents back to TOC #####Example using promises
smartsheet.home.listContents({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.home.listContents({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listFolders back to TOC #####Example using promises
smartsheet.home.listFolders({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.home.listFolders({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createFolder back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.home.createFolder(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.home.createFolder(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Report API methods
####listReports back to TOC #####Example using promises
smartsheet.reports.listReports({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.listReports({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getReport back to TOC #####Example using promises
smartsheet.reports.getReport({id: reportsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.getReport({id: reportsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####sendReportViaEmail back to TOC #####Example using promises
smartsheet.reports.sendReportViaEmail({id: reportsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.sendReportViaEmail({id: reportsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getReportAsExcel back to TOC #####Example using promises
smartsheet.reports.getReportAsExcel({id: reportsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.getReportAsExcel({id: reportsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getReportAsCSV back to TOC #####Example using promises
smartsheet.reports.getReportAsCSV({id: reportsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.getReportAsCSV({id: reportsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getShare back to TOC #####Example using promises
smartsheet.reports.getShare({id: reportsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.getShare({id: reportsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listShares back to TOC #####Example using promises
smartsheet.reports.listShares({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.listShares({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####share back to TOC #####Example using promises
smartsheet.reports.share({id: reportsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.reports.share({id: reportsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteShare back to TOC #####Example using promises
var options = {
id: reportsId
}
smartsheet.reports.deleteShare(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: reportsId
}
smartsheet.reports.deleteShare(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateShare back to TOC #####Example using promises
var options = {
id: reportsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.reports.updateShare(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: reportsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.reports.updateShare(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Search API methods
####searchAll back to TOC #####Example using promises
smartsheet.search.searchAll({id: searchId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.search.searchAll({id: searchId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####searchSheet back to TOC #####Example using promises
smartsheet.search.searchSheet({id: searchId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.search.searchSheet({id: searchId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Server API methods
####getInfo back to TOC #####Example using promises
smartsheet.server.getInfo({id: serverId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.server.getInfo({id: serverId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Sheet API methods
####sendSheetViaEmail back to TOC #####Example using promises
smartsheet.sheets.sendSheetViaEmail({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.sendSheetViaEmail({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getPublishStatus back to TOC #####Example using promises
smartsheet.sheets.getPublishStatus({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getPublishStatus({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####setPublishStatus back to TOC #####Example using promises
smartsheet.sheets.setPublishStatus({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.setPublishStatus({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateSheet back to TOC #####Example using promises
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateSheet(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateSheet(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteSheet back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteSheet(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteSheet(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getAttachment back to TOC #####Example using promises
smartsheet.sheets.getAttachment({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getAttachment({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listAttachments back to TOC #####Example using promises
smartsheet.sheets.listAttachments({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.listAttachments({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listAttachmentVersions back to TOC #####Example using promises
smartsheet.sheets.listAttachmentVersions({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.listAttachmentVersions({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addAttachment back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addAttachment(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addAttachment(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####attachNewVersion back to TOC #####Example using promises
smartsheet.sheets.attachNewVersion({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.attachNewVersion({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteAttachment back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteAttachment(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteAttachment(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteAllAttachmentVersions back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteAllAttachmentVersions(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteAllAttachmentVersions(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getColumns back to TOC #####Example using promises
smartsheet.sheets.getColumns({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getColumns({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getColumn back to TOC #####Example using promises
smartsheet.sheets.getColumn({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getColumn({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addColumn back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addColumn(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addColumn(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteColumn back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteColumn(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteColumn(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateColumn back to TOC #####Example using promises
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateColumn(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateColumn(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getComment back to TOC #####Example using promises
smartsheet.sheets.getComment({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getComment({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteComment back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteComment(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteComment(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addCommentAttachment back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addCommentAttachment(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addCommentAttachment(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createSheet back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheet(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheet(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createSheetFromExisting back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheetFromExisting(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheetFromExisting(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createSheetInFolder back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheetInFolder(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheetInFolder(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createSheetInWorkspace back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheetInWorkspace(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createSheetInWorkspace(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getDiscussions back to TOC #####Example using promises
smartsheet.sheets.getDiscussions({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getDiscussions({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getDiscussion back to TOC #####Example using promises
smartsheet.sheets.getDiscussion({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getDiscussion({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listDiscussionAttachments back to TOC #####Example using promises
smartsheet.sheets.listDiscussionAttachments({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.listDiscussionAttachments({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createDiscussion back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createDiscussion(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createDiscussion(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addDiscussionComment back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addDiscussionComment(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addDiscussionComment(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteDiscussion back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteDiscussion(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteDiscussion(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getSheet back to TOC #####Example using promises
smartsheet.sheets.getSheet({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getSheet({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listSheets back to TOC #####Example using promises
smartsheet.sheets.listSheets({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.listSheets({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getSheetAsCSV back to TOC #####Example using promises
smartsheet.sheets.getSheetAsCSV({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getSheetAsCSV({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getSheetAsExcel back to TOC #####Example using promises
smartsheet.sheets.getSheetAsExcel({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getSheetAsExcel({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getSheetAsPDF back to TOC #####Example using promises
smartsheet.sheets.getSheetAsPDF({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getSheetAsPDF({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getSheetVersion back to TOC #####Example using promises
smartsheet.sheets.getSheetVersion({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getSheetVersion({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listOrganizationSheets back to TOC #####Example using promises
smartsheet.sheets.listOrganizationSheets({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.listOrganizationSheets({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getRow back to TOC #####Example using promises
smartsheet.sheets.getRow({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getRow({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getRowAttachments back to TOC #####Example using promises
smartsheet.sheets.getRowAttachments({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getRowAttachments({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getRowDiscussions back to TOC #####Example using promises
smartsheet.sheets.getRowDiscussions({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getRowDiscussions({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getCellHistory back to TOC #####Example using promises
smartsheet.sheets.getCellHistory({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getCellHistory({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####copyRowToAnotherSheet back to TOC #####Example using promises
smartsheet.sheets.copyRowToAnotherSheet({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.copyRowToAnotherSheet({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####moveRowToAnotherSheet back to TOC #####Example using promises
smartsheet.sheets.moveRowToAnotherSheet({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.moveRowToAnotherSheet({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addRow back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addRow(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addRow(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addRows back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addRows(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addRows(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addRowAttachment back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addRowAttachment(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.addRowAttachment(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createRowDiscussion back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createRowDiscussion(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.createRowDiscussion(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####sendRow back to TOC #####Example using promises
smartsheet.sheets.sendRow({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.sendRow({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteRow back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteRow(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteRow(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateRow back to TOC #####Example using promises
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateRow(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateRow(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getShare back to TOC #####Example using promises
smartsheet.sheets.getShare({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.getShare({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listShares back to TOC #####Example using promises
smartsheet.sheets.listShares({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.listShares({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####share back to TOC #####Example using promises
smartsheet.sheets.share({id: sheetsId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.sheets.share({id: sheetsId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteShare back to TOC #####Example using promises
var options = {
id: sheetsId
}
smartsheet.sheets.deleteShare(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId
}
smartsheet.sheets.deleteShare(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateShare back to TOC #####Example using promises
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateShare(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: sheetsId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.sheets.updateShare(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Template API methods
####listUserCreatedTemplates back to TOC #####Example using promises
smartsheet.templates.listUserCreatedTemplates({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.templates.listUserCreatedTemplates({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listPublicTemplates back to TOC #####Example using promises
smartsheet.templates.listPublicTemplates({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.templates.listPublicTemplates({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###User API methods
####getUser back to TOC #####Example using promises
smartsheet.users.getUser({id: usersId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.users.getUser({id: usersId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listAllUsers back to TOC #####Example using promises
smartsheet.users.listAllUsers({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.users.listAllUsers({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getCurrentUser back to TOC #####Example using promises
smartsheet.users.getCurrentUser({id: usersId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.users.getCurrentUser({id: usersId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addUser back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.users.addUser(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.users.addUser(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####addUserAndSendEmail back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.users.addUserAndSendEmail(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.users.addUserAndSendEmail(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateUser back to TOC #####Example using promises
var options = {
id: usersId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.users.updateUser(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: usersId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.users.updateUser(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####removeUser back to TOC #####Example using promises
smartsheet.users.removeUser({id: usersId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.users.removeUser({id: usersId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
###Workspace API methods
####listWorkspaces back to TOC #####Example using promises
smartsheet.workspaces.listWorkspaces({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.workspaces.listWorkspaces({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getWorkspace back to TOC #####Example using promises
smartsheet.workspaces.getWorkspace({id: workspacesId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.workspaces.getWorkspace({id: workspacesId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listWorkspaceFolders back to TOC #####Example using promises
smartsheet.workspaces.listWorkspaceFolders({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.workspaces.listWorkspaceFolders({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createWorkspace back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.createWorkspace(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.createWorkspace(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####createFolder back to TOC #####Example using promises
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.createFolder(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.createFolder(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteWorkspace back to TOC #####Example using promises
var options = {
id: workspacesId
}
smartsheet.workspaces.deleteWorkspace(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: workspacesId
}
smartsheet.workspaces.deleteWorkspace(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateWorkspace back to TOC #####Example using promises
var options = {
id: workspacesId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.updateWorkspace(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: workspacesId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.updateWorkspace(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####getShare back to TOC #####Example using promises
smartsheet.workspaces.getShare({id: workspacesId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.workspaces.getShare({id: workspacesId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####listShares back to TOC #####Example using promises
smartsheet.workspaces.listShares({})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.workspaces.listShares({}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####share back to TOC #####Example using promises
smartsheet.workspaces.share({id: workspacesId})
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
smartsheet.workspaces.share({id: workspacesId}, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####deleteShare back to TOC #####Example using promises
var options = {
id: workspacesId
}
smartsheet.workspaces.deleteShare(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: workspacesId
}
smartsheet.workspaces.deleteShare(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
####updateShare back to TOC #####Example using promises
var options = {
id: workspacesId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.updateShare(options)
.then(function(data) {
console.log(data);
})
.catch(function(error) {
console.log(error);
});
#####Example using callbacks
var options = {
id: workspacesId,
body: {
//body of request as specified in the Smartsheet API documentation
},
queryParameters: {
//querystring parameters as specified in the Smartsheet API documentation
}
};
smartsheet.workspaces.updateShare(options, function(error, data) {
if (error) {
console.log(error);
}
console.log(data);
});
##Release Notes Each specific release is available for download via Github.
0.0.2 - August 12, 2015
FAQs
Smartsheet JavaScript client SDK
The npm package smartsheet receives a total of 12,613 weekly downloads. As such, smartsheet popularity was classified as popular.
We found that smartsheet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.