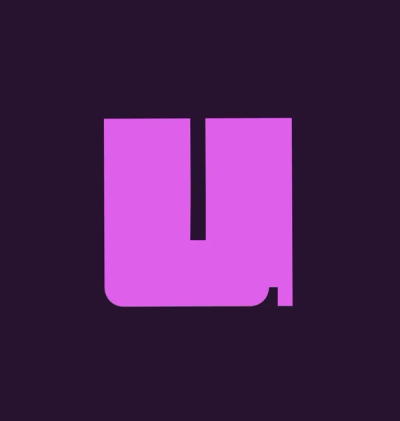
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Home | Docs | GitHub | npm | Changelog | YouTube | Small math function library
smath can be installed from the official npm package repository. It is highly recommended to install the latest version, which is installed by default with the following command.
npm i smath@1.7.0
Is there a way we can make smath better? Please report all bugs, issues, and new feature requests to the issues page in the official repository. For critical security issues, please send an email to smath@nicfv.com.
Thank you for your interest in contributing to smath! smath is an open source software package maintained by Nicolas Ventura (@nicfv) and built by users like you! You are allowed to fork the repository as permitted by the MIT License terms. Contributions are welcome by submitting a pull request. Please follow the existing code styling if submitting a pull request. Thank you for your consideration!
Small math? Simple math? Or supplemental math? Canonically, "SMath" is pronounced "smath" and stands for "small math (library.)" Similar to JavaScript's builtin Math
object, SMath
exports one global object with several math-related helper functions. There is no need to instantiate the class, just call functions directly. See the examples below to get started using SMath!
SMath is also packaged with an executabe that can be run directly through npx
in the terminal - even outside of a NodeJS project! In fact, open your terminal now, and type the following to show a list of valid npx smath
commands!
npx smath
Commands are all structured like this.
npx smath [cmd] [args]
This example command returns the value 0.4.
npx smath normalize 4 0 10
Here are a few quickstart examples written in JavaScript that showcase some out-of-box features of the smath
package.
Sometimes, JavaScript does weird math! Try adding 0.1 + 0.2
in your NodeJS terminal. What did you get?
Hint: It's not 0.3!
The function SMath.approx()
is an attempt to mitigate some of the issues that arise when doing arithmetic with non-whole numbers.
JavaScript-Math-Oddities.mjs
node JavaScript-Math-Oddities.mjs
import { SMath } from 'smath';
// Determine the value of 0.1 + 0.2 using vanilla JavaScript and SMath
console.log('0.1 + 0.2 == 0.3 is ' + (0.1 + 0.2 == 0.3));
console.log('SMath.approx(0.1 + 0.2, 0.3) is ' + SMath.approx(0.1 + 0.2, 0.3));
0.1 + 0.2 == 0.3 is false
SMath.approx(0.1 + 0.2, 0.3) is true
This example demonstrates a simple temperature converter from Celsius to Fahrenheit, using SMath.translate()
to linearly interpolate between unit systems. The translation uses freezing and boiling points to fix the bounds of the linear interpolation.
Temperature-Conversion.mjs
node Temperature-Conversion.mjs
import { SMath } from 'smath';
// Water always freezes at the
// same temperature, but the
// units might be different.
// Define some constants to
// create two number ranges.
const C_Freeze = 0,
C_Boil = 100,
F_Freeze = 32,
F_Boil = 212;
// Use the `SMath` class to
// generate an array of five
// linearly spaced temperature
// values from 0 to 20.
const C = SMath.linspace(0, 20, 5);
// Use the `SMath` class to linearly
// interpolate the temperature in the
// C number range to a temperature
// in the F number range.
const F = C.map(c => SMath.translate(c, C_Freeze, C_Boil, F_Freeze, F_Boil));
// Print out each temperature
// in both units of C and F.
for (let i = 0; i < C.length; i++) {
console.log(C[i].toFixed() + 'C is ' + F[i].toFixed() + 'F')
}
0C is 32F
5C is 41F
10C is 50F
15C is 59F
20C is 68F
FAQs
Small math function library
We found that smath demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.