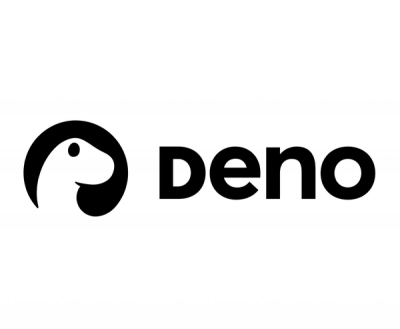
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
social-api-js
Advanced tools
A library that provides a standard JS interface to dynamically load (or lazy load) all social network APIs (good for sites that integrate multiple social network APIs together).
Supports the following APIs:
In order to use this package, you must be using a compiler to utilize the latest ES6 javascript syntax.
I recommend installing babel (or similiar) and import
ing the source files directly.
import {Facebook} from 'social-api';
let options = {
apiConfig: {appId: 'MyAP33IYEK3y'}
};
Facebook.load(options, function (FB) {
// API loaded! Now, do something with the FB object
console.log(FB);
});
import {Twitter} from 'social-api';
Twitter.load({}, function (twttr) {
// API loaded! Now, do something with the twitter object
console.log(twttr);
});
import {Tumblr} from 'social-api';
let options = {
apiConfig: {
api_key: 'vtoiBQGHzJfvtNaFXK7T5DJdIM8ozpjPPzcF9z6EUxZDSELGxd',
'base-hostname': 'janey-smith.tumblr.com'
}
};
Tumblr.load(options, function () {
// API loaded!
});
import {Instagram} from 'social-api';
Instagram.load({}, function () {
// API loaded!
});
import {Vine} from 'social-api';
let el = document.getElementsByTagName('div')[0];
Vine.load({}, function () {
// API loaded! Show an embed
el.innerHTML = '<iframe class="vine-embed" src="https://vine.co/v/sf90dfs/embed/simple" width="600" height="600" frameborder="0"></iframe>';
});
FAQs
Social network API library
The npm package social-api-js receives a total of 1 weekly downloads. As such, social-api-js popularity was classified as not popular.
We found that social-api-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.