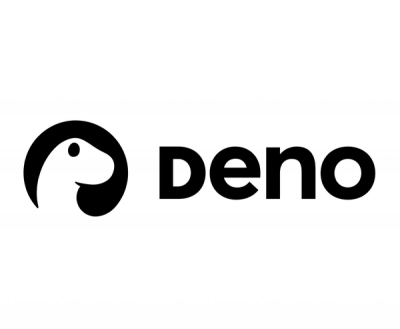
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
socket.io-client
Advanced tools
The socket.io-client npm package is a client-side library that enables real-time, bidirectional and event-based communication between web clients and servers. It works on every platform, browser or device, focusing equally on reliability and speed.
Connecting to a server
This code sample demonstrates how to connect to a Socket.IO server running on localhost at port 3000.
const io = require('socket.io-client');
const socket = io('http://localhost:3000');
Emitting events
This code sample shows how to emit an event to the server with an event name and associated data.
socket.emit('event_name', { some: 'data' });
Listening for events
This code sample illustrates how to listen for events emitted by the server and handle them with a callback function.
socket.on('event_name', function(data) {
console.log(data);
});
Disconnecting from the server
This code sample shows how to handle a disconnection event when the client gets disconnected from the server.
socket.on('disconnect', function() {
console.log('Disconnected from server');
});
The 'ws' package is a simple to use, blazing fast, and thoroughly tested WebSocket client and server implementation. Unlike socket.io-client, which provides an abstraction over WebSocket with additional features like auto-reconnection, 'ws' is a bare WebSocket implementation.
Faye is a set of tools for simple publish-subscribe messaging between web clients. It's similar to socket.io-client in that it provides an abstraction over WebSocket for real-time communication, but it has a different API and feature set.
SockJS-client is a browser JavaScript library that provides a WebSocket-like object. SockJS gives you a coherent, cross-browser, Javascript API which creates a low latency, full duplex, cross-domain communication channel between the browser and the web server, with WebSockets or without. It is similar to socket.io-client but focuses more on providing fallback options for environments where WebSocket is not available.
Please see the documentation here.
The source code of the website can be found here. Contributions are welcome!
In order to see all the client debug output, run the following command on the browser console – including the desired scope – and reload your app page:
localStorage.debug = '*';
And then, filter by the scopes you're interested in. See also: https://socket.io/docs/v4/logging-and-debugging/
FAQs
Realtime application framework client
The npm package socket.io-client receives a total of 4,179,859 weekly downloads. As such, socket.io-client popularity was classified as popular.
We found that socket.io-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.