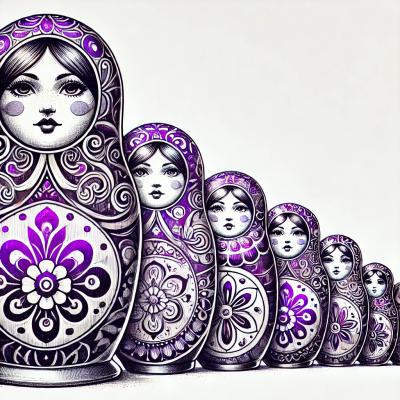
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
socket.io-parser
Advanced tools
The socket.io-parser npm package is a component of the Socket.IO ecosystem, which is used for encoding and decoding messages that are sent over the Socket.IO protocol. It handles the serialization and deserialization of the various types of messages that can be transmitted, such as events, binary data, and control packets.
Encoding messages
This feature allows you to encode messages into a format that can be transmitted over the Socket.IO protocol. The code sample represents a JSON string that has been encoded by the socket.io-parser for an event with some data.
{"type":2,"nsp":"/","data":["event",{"some":"data"}]}
Decoding messages
This feature is used to decode messages received over the Socket.IO protocol. The code sample shows how an encoded message (a string) is decoded back into its original form using the socket.io-parser.
const encodedMessage = '4hello world';
const decodedMessage = parser.decode(encodedMessage);
console.log(decodedMessage);
Binary support
The socket.io-parser can handle binary data by encoding and decoding it as part of the message. The code sample demonstrates how to encode an ArrayBuffer as binary data within a message.
const binaryData = new ArrayBuffer(100);
const message = parser.encodeAsBinary({ type: 5, data: binaryData });
console.log(message);
This package is part of the Engine.IO library, which is a lower-level component of the Socket.IO framework. It provides similar encoding and decoding functionalities for the Engine.IO protocol, which is a bit more focused on the transport layer compared to the higher-level abstraction provided by socket.io-parser.
While not a direct alternative to socket.io-parser, the 'ws' package is a popular WebSocket library for Node.js that allows for sending and receiving data over WebSockets. It handles the WebSocket protocol directly, without the additional features and abstractions that Socket.IO provides.
json-socket is a wrapper around the Node.js net library that adds a layer for seamless JSON message sending and receiving. It's similar to socket.io-parser in that it deals with message serialization and deserialization, but it's tailored for use with raw TCP sockets rather than the Socket.IO protocol.
A socket.io encoder and decoder written in JavaScript complying with version 3
of socket.io-protocol.
Used by socket.io and
socket.io-client.
socket.io-parser is the reference implementation of socket.io-protocol. Read the full API here: socket.io-protocol.
var parser = require('socket.io-parser');
var encoder = new parser.Encoder();
var packet = {
type: parser.EVENT,
data: 'test-packet',
id: 13
};
encoder.encode(packet, function(encodedPackets) {
var decoder = new parser.Decoder();
decoder.on('decoded', function(decodedPacket) {
// decodedPacket.type == parser.EVENT
// decodedPacket.data == 'test-packet'
// decodedPacket.id == 13
});
for (var i = 0; i < encodedPackets.length; i++) {
decoder.add(encodedPackets[i]);
}
});
var parser = require('socket.io-parser');
var encoder = new parser.Encoder();
var packet = {
type: parser.BINARY_EVENT,
data: {i: new Buffer(1234), j: new Blob([new ArrayBuffer(2)])}
id: 15
};
encoder.encode(packet, function(encodedPackets) {
var decoder = new parser.Decoder();
decoder.on('decoded', function(decodedPacket) {
// decodedPacket.type == parser.BINARY_EVENTEVENT
// Buffer.isBuffer(decodedPacket.data.i) == true
// Buffer.isBuffer(decodedPacket.data.j) == true
// decodedPacket.id == 15
});
for (var i = 0; i < encodedPackets.length; i++) {
decoder.add(encodedPackets[i]);
}
});
See the test suite for more examples of how socket.io-parser is used.
FAQs
socket.io protocol parser
The npm package socket.io-parser receives a total of 8,224,850 weekly downloads. As such, socket.io-parser popularity was classified as popular.
We found that socket.io-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.