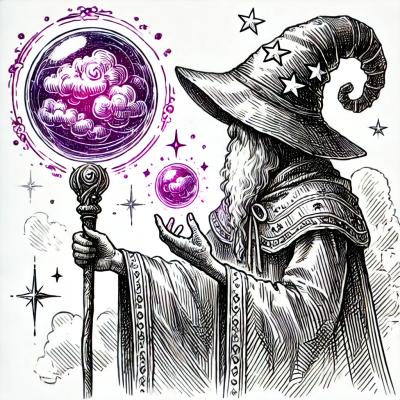
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
sorted-btree
Advanced tools
A list of key-value pairs kept sorted in a fast in-memory B+ tree with a powerful API, similar to Map.
B+ trees are ordered collections of key-value pairs, sorted by key.
This is a fast B+ tree implementation, largely compatible with the standard Map, but with a much more diverse and powerful API. To use it, import BTree<K,V> from 'sorted-btree'
.
Map<K,V>
with methods such as size(), clear()
,
foreach((k,v,tree)=>void), get(K), set(K,V), has(K), delete(K)
,
plus iterator functions keys()
, values()
and entries()
.undefined
is associated
with all keys in a given node.Range
methods for batch operationsNaN
as a key, but infinity is allowed.Additional operations supported on this B+ tree:
t.setIfNotPresent(k,v)
t.changeIfPresent(k,v)
for (pair of t.entriesReversed()) {}
for (let p of t.entries(first)) {}
t.toArray()
, t.keysArray()
, t.valuesArray()
t.getRange(loK, hiK, includeHi)
t.deleteRange(loK, hiK, includeHi)
t.forEachPair((key, value, index) => {...})
t.forRange(lowKey, highKey, includeHighFlag, (k,v) => {...})
c = t.forRange(loK, hiK, includeHi, undefined)
t.minKey()
, t.maxKey()
t.freeze()
(you can also t.unfreeze()
)t.clone()
Note: Confusingly, the ES6 Map.forEach(c)
method calls c(value,key)
instead of c(key,value)
, in contrast to other methods such as set()
and entries()
which put the key first. I can only assume that they reversed the order on the theory that users would usually want to examine values and ignore keys. BTree's forEach()
therefore works the same way, but a second method .forEachPair((key,value)=>{...})
is provided which sends you the key first and the value second; this method is slightly faster because it is the "native" for-each method for this class.
The "scanning" methods (forEach, forRange, editRange, deleteRange
) will normally return the number of elements that were scanned. However, the callback can return {break:R}
to stop iterating early and return a value R
from the scanning method.
B+ trees normally use memory more efficiently than hashtables (such as the standard Map
), although in JavaScript this is not guaranteed because the B+ tree's memory efficiency depends on avoiding wasted space in the arrays for each node, and JavaScript provides no way to detect or control the capacity of an array's underlying memory area.
Given a set of {name: string, age: number}
objects, you can create a tree sorted by name and then by age like this:
// First constructor argument is an optional list of pairs ([K,V][])
var tree = new BTree(undefined, (a, b) => {
if (a.name > b.name)
return 1; // Return a number >0 when a > b
else if (a.name < b.name)
return -1; // Return a number <0 when a < b
else // names are equal (or incomparable)
return a.age - b.age; // Return >0 when a.age > b.age
});
tree.set({name:"Bill", age:17}, "happy");
tree.set({name:"Fran", age:40}, "busy & stressed");
tree.set({name:"Bill", age:55}, "recently laid off");
tree.forEachPair((k, v) => {
console.log(`Name: ${k.name} Age: ${k.age} Status: ${v}`);
});
You can scan a range of items and selectively delete or change some of them using t.editRange
. For example, the following code adds an exclamation mark to each non-boring value and deletes key number 4:
var t = new BTree().setRange([[1,"fun"],[2,"yay"],[4,"whee"],[8,"zany"],[10,"boring"]);
t.editRange(t.minKey(), t.maxKey(), true, (k, v) => {
if (k === 4)
return {delete: true};
if (v !== "boring")
return {value: v + '!'};
})
♥ This package was made to help people learn TypeScript & React.
Are you a C# developer? You might like the similar data structures I made for C#: BDictionary, BList, etc. See http://core.loyc.net/collections/
FAQs
A sorted list of key-value pairs in a fast, typed in-memory B+ tree with a powerful API.
We found that sorted-btree demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.