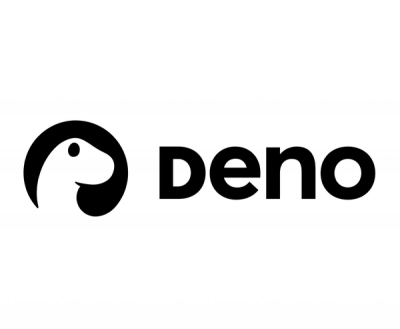
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Easily test your Electron apps using ChromeDriver and WebdriverIO.
This minor version of this library tracks the minor version of the Electron
versions released. So if you are using Electron 0.33.x
you would want to use
a spectron
dependency of ^0.33
in your package.json
file.
Learn more from this presentation.
npm install --save-dev spectron
Spectron works with any testing framework but the following example uses mocha:
var Application = require('spectron').Application
var assert = require('assert')
describe('application launch', function () {
this.timeout(10000)
beforeEach(function () {
this.app = new Application({
path: '/Applications/MyApp.app/Contents/MacOS/MyApp'
})
return this.app.start()
})
afterEach(function () {
if (this.app && this.app.isRunning()) {
return this.app.stop()
}
})
it('shows an initial window', function () {
return this.app.client.getWindowCount().then(function (count) {
assert.equal(count, 1)
})
})
})
WebdriverIO is promise-based and so it pairs really well with the Chai as Promised library that builds on top of Chai.
Using these together allows you to chain assertions together and have fewer callback blocks. See below for a simple example:
npm install --save-dev chai
npm install --save-dev chai-as-promised
var Application = require('spectron').Application
var chai = require('chai')
var chaiAsPromised = require('chai-as-promised')
var path = require('path')
chai.should()
chai.use(chaiAsPromised)
describe('application launch', function () {
beforeEach(function () {
this.app = new Application({
path: '/Applications/MyApp.app/Contents/MacOS/MyApp'
})
return this.app.start()
})
beforeEach(function () {
chaiAsPromised.transferPromiseness = this.app.client.transferPromiseness
})
afterEach(function () {
if (this.app && this.app.isRunning()) {
return this.app.stop()
}
})
it('opens a window', function () {
return this.app.client.waitUntilWindowLoaded()
.getWindowCount().should.eventually.equal(1)
.isWindowMinimized().should.eventually.be.false
.isWindowDevToolsOpened().should.eventually.be.false
.isWindowVisible().should.eventually.be.true
.isWindowFocused().should.eventually.be.true
.getWindowWidth().should.eventually.be.above(0)
.getWindowHeight().should.eventually.be.above(0)
})
})
You will want to add the following to your .travis.yml
file when building on
Linux:
before_script:
- "export DISPLAY=:99.0"
- "sh -e /etc/init.d/xvfb start"
- sleep 3 # give xvfb some time to start
Check out Spectron's .travis.yml file for a production example.
You will want to add the following to your appveyor.yml
file:
os: unstable
Check out Spectron's appveyor.yml file for a production example.
Create a new application with the following options:
path
- String path to the application executable to launch. Requiredargs
- Array of arguments to pass to the executable.
See here
for details on the Chrome arguments.env
- Object of additional environment variables to set in the launched
application.host
- String host name of the launched chromedriver
process.
Defaults to 'localhost'
.port
- Number port of the launched chromedriver
process.
Defaults to 9515
.quitTimeout
- Number in milliseconds to wait for application quitting.
Defaults to 1000
milliseconds.waitTimeout
- Number in milliseconds to wait for calls like
waitUntilTextExists
and waitUntilWindowLoaded
to complete.
Defaults to 5000
milliseconds.Starts the application. Returns a Promise
that will be resolved when the
application is ready to use. You should always wait for start to complete
before running any commands.
Stops the application. Returns a Promise
that will be resolved once the
application has stopped.
Spectron uses WebdriverIO and exposes the managed
client
property on the created Application
instances.
The full client
API provided by WebdriverIO can be found
here.
Several additional commands are provided specific to Electron.
All the commands return a Promise
.
Get the argv
array from the main process.
app.client.getArgv().then(function (argv) {
console.log(argv)
})
Gets the clipboard text.
app.client.getClipboardText().then(function (clipboardText) {
console.log(clipboardText)
})
Gets the represented file name. Only supported on Mac OS X.
app.client.getRepresentedFilename().then(function (filename) {
console.log(filename)
})
Get the selected text in the current window.
app.client.getSelectedText().then(function (selectedText) {
console.log(selectedText)
})
Gets the number of open windows.
app.client.getWindowCount().then(function (count) {
console.log(count)
})
Gets the bounds of the current window. Object returned has
x
, y
, width
, and height
properties.
app.client.getWindowBounds().then(function (bounds) {
console.log(bounds.x, bounds.y, bounds.width, bounds.height)
})
Get the height of the current window.
app.client.getWindowHeight().then(function (height) {
console.log(height)
})
Get the width of the current window.
app.client.getWindowWidth().then(function (width) {
console.log(width)
})
Returns true if the document is edited, false otherwise. Only supported on Mac OS X.
app.client.isDocumentEdited().then(function (edited) {
console.log(edited)
})
Returns whether the current window's dev tools are opened.
app.client.isWindowDevToolsOpened().then(function (devToolsOpened) {
console.log(devToolsOpened)
})
Returns whether the current window has focus.
app.client.isWindowFocused().then(function (focused) {
console.log(focused)
})
Returns whether the current window is in full screen mode.
app.client.isWindowFullScreen().then(function (fullScreen) {
console.log(fullScreen)
})
Returns whether the current window is loading.
app.client.isWindowLoading().then(function (loading) {
console.log(loading)
})
Returns whether the current window is maximized.
app.client.isWindowMaximized().then(function (maximized) {
console.log(maximized)
})
Returns whether the current window is minimized.
app.client.isWindowMinimized().then(function (minimized) {
console.log(minimized)
})
Returns whether the current window is visible.
app.client.isWindowVisible().then(function (visible) {
console.log(visible)
})
Paste the text from the clipboard in the current window.
app.client.paste()
Select all the text in the current window.
app.client.selectAll()
Sets the clipboard text.
app.client.setClipboardText('pasta')
Sets the document edited state. Only supported on Mac OS X.
app.client.setDocumentEdited(true)
Sets the represented file name. Only supported on Mac OS X.
app.client.setRepresentedFilename('/foo.js')
Sets the window position and size. The bounds object should have x
, y
,
height
, and width
keys.
app.client.setWindowBounds({x: 100, y: 200, width: 50, height: 75})
Waits until the element matching the given selector contains the given
text. Takes an optional timeout in milliseconds that defaults to 5000
.
app.client.waitUntilTextExists('#message', 'Success', 10000)
Wait until the window is no longer loading. Takes an optional timeout
in milliseconds that defaults to 5000
.
app.client.waitUntilWindowLoaded(10000)
Focus a window using its index from the windowHandles()
array.
app.client.windowByIndex(1)
0.34.1
waitTimeout
config option to Application
that sets the default
millisecond timeout for all wait-based command helpers like waitUntil
,
waitUntilWindowLoaded
, etc. This option defaults to 5 seconds.windowByIndex(index)
command helper that focuses a window by
index in the windowHandles()
array order.setRepresentedFilename
and getRepresentedFilename
command helpers.isDocumentEdited
and setDocumentEdited
command helpers.setWindowDimensions
was renamed to setWindowBounds
to mirror the new
Electron BrowserWindow.setBounds
API. It also takes a bounds
object
argument instead of multiple arguments for size and position. See the
README
for an examplegetWindowDimensions
was renamed to getWindowBounds
to mirror the new
Electron BrowserWindow.getBounds
API. See the README
for an example.FAQs
Easily test your Electron apps using ChromeDriver and WebdriverIO.
The npm package spectron receives a total of 6,502 weekly downloads. As such, spectron popularity was classified as popular.
We found that spectron demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.