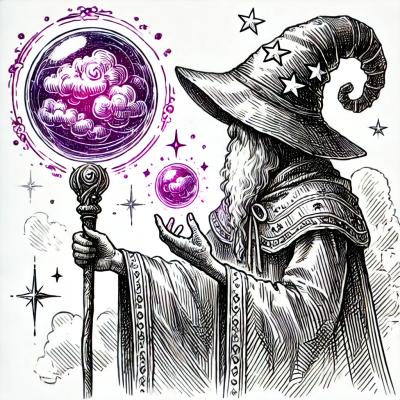
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
sql-formatter
Advanced tools
The sql-formatter npm package is a library for formatting SQL queries. It supports various SQL dialects and provides a clean and readable output for SQL statements.
Basic SQL Formatting
This feature allows you to format a basic SQL query into a more readable format.
const sqlFormatter = require('sql-formatter');
const formattedSQL = sqlFormatter.format('SELECT * FROM table WHERE column = value');
console.log(formattedSQL);
Dialect-Specific Formatting
This feature allows you to format SQL queries according to specific SQL dialects like MySQL, PostgreSQL, etc.
const sqlFormatter = require('sql-formatter');
const formattedSQL = sqlFormatter.format('SELECT * FROM table WHERE column = value', { language: 'mysql' });
console.log(formattedSQL);
Custom Indentation
This feature allows you to customize the indentation used in the formatted SQL output.
const sqlFormatter = require('sql-formatter');
const formattedSQL = sqlFormatter.format('SELECT * FROM table WHERE column = value', { indent: ' ' });
console.log(formattedSQL);
sql-formatter-plus is another SQL formatter that offers similar functionality to sql-formatter. It also supports multiple SQL dialects and provides options for customizing the output format. However, it may have different default formatting styles and additional configuration options.
prettier-plugin-sql is a plugin for the Prettier code formatter that adds support for formatting SQL queries. It integrates with Prettier's ecosystem, allowing you to format SQL queries alongside other code formats supported by Prettier. This can be useful if you are already using Prettier for other parts of your codebase.
SQL Formatter is a JavaScript library for pretty-printing SQL queries. It started as a port of a PHP Library, but has since considerably diverged. It supports Standard SQL and Couchbase N1QL dialects.
Get the latest version from NPM:
npm install --save sql-formatter
import sqlFormatter from "sql-formatter";
console.log(sqlFormatter.format("SELECT * FROM table1"));
This will output:
SELECT
*
FROM
table1
You can also pass in configuration options:
sqlFormatter.format("SELECT *", {
language: "n1ql" // Defaults to "sql"
indent: " " // Defaults to two spaces
});
Currently just two SQL dialects are supported:
// Named placeholders
sqlFormatter.format("SELECT * FROM tbl WHERE foo = @foo", {
params: {foo: "'bar'"}
}));
// Indexed placeholders
sqlFormatter.format("SELECT * FROM tbl WHERE foo = ?", {
params: ["'bar'"]
}));
Both result in:
SELECT
*
FROM
tbl
WHERE
foo = 'bar'
If you don't use a module bundler, clone the repository, run npm install
and grab a file from /dist
directory to use inside a <script>
tag.
This makes SQL Formatter available as a global variable window.sqlFormatter
.
# run linter and tests
$ npm run check
...and you're ready to poke us with a pull request.
FAQs
Format whitespace in a SQL query to make it more readable
The npm package sql-formatter receives a total of 532,192 weekly downloads. As such, sql-formatter popularity was classified as popular.
We found that sql-formatter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.