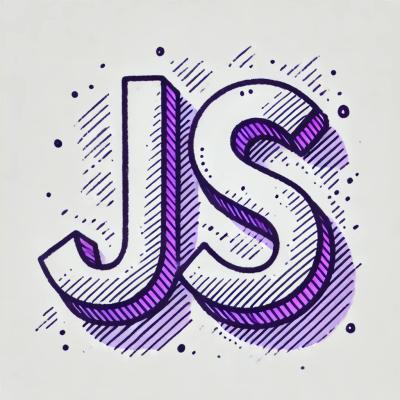
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
The 'standard' npm package is a JavaScript style guide, linter, and formatter all in one. It enforces a consistent coding style without the need for configuration, making it easier to maintain code quality across projects.
Linting
Linting is the process of running a program that will analyze code for potential errors. The 'standard' package provides a zero-configuration linter that checks for style and programming errors.
npx standard
Auto-fixing
The 'standard' package can automatically fix some of the issues it finds in your code. This feature helps in maintaining code quality by automatically correcting common style and formatting issues.
npx standard --fix
Integration with Editors
The 'standard' package can be integrated with various code editors like VSCode, Sublime Text, and Atom. This allows for real-time linting and auto-fixing as you write code.
/* Example for VSCode */
{
"editor.formatOnSave": true,
"javascript.validate.enable": false,
"standard.enable": true
}
ESLint is a highly configurable linter for JavaScript and JSX. Unlike 'standard', which comes with a predefined set of rules, ESLint allows you to define your own rules or extend from popular style guides like Airbnb or Google.
Prettier is an opinionated code formatter that supports many languages. It focuses on code formatting rather than linting. While 'standard' includes both linting and formatting, Prettier is often used in conjunction with ESLint for a more comprehensive solution.
XO is a JavaScript linter with great defaults and minimal configuration. It is similar to 'standard' in that it aims to provide a zero-config experience, but it also allows for some customization and extends ESLint under the hood.
No decisions to make, no .jshintrc
or .jscs
files to manage. It just works.
npm install standard
"in this lil' string"
(
or [
:
;
like this ;[1, 2, 3].join(' ')
if (condition) { ... }
function name (arg1, arg2) { ... }
self
:
var self = this
===
instead of ==
To get a better idea, take a look at a sample file written in JavaScript Standard Style.
The easiest way to use standard
is to install it globally as a Node command line
program. To do so, simply run the following command in your terminal (flag -g
installs
standard
globally on your system, omit it if you want to install in the current working
directory):
npm install standard -g
After you've done that you should be able to use the standard
program. The simplest use
case would be checking the style of all JavaScript files in the current working directory:
$ standard
Error: Code style check failed:
/Users/feross/code/webtorrent/lib/torrent.js:950:11: Expected '===' and instead saw '=='.
The paths node_modules/
, .git/
, *.min.js
, and bundle.js
are automatically excluded
when looking for .js
files to style check.
package.json
{
"name": "my-cool-package",
"devDependencies": {
"standard": "*"
},
"scripts": {
"test": "standard && node my-normal-tests.js"
}
}
npm test
$ npm test
Error: Code style check failed:
/Users/feross/code/webtorrent/lib/torrent.js:950:11: Expected '===' and instead saw '=='.
MIT. Copyright (c) Feross Aboukhadijeh.
FAQs
JavaScript Standard Style
The npm package standard receives a total of 337,003 weekly downloads. As such, standard popularity was classified as popular.
We found that standard demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 16 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.