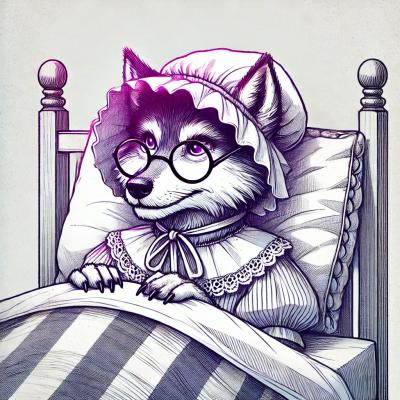
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
startupjs
Advanced tools
:fire: React (Native + Web) starter kit and realtime model synchronization engine for Node.js with ORM (full-stack framework).
This boilerplate launches with a React web app and React Native app and allows to use a single code for all platforms.
The project is super helpful to kick-start your next project, as it provides a lot of the common tools you may reach for, all ready to go. Specifically:
OR
myapp
to your project name (use lower case)npx startupjs init myapp --version=latest && cd myapp
yarn server
http://localhost:3000
yarn web
yarn metro
yarn android
yarn ios
Alternatively you can run a docker development image which has node, yarn, mongo and redis already built in.
You only need docker
for this. And it works everywhere -- Windows, MacOS, Linux.
Keep in mind though that since docker uses its own driver to mount folders, performance (especially when installing modules) might be considerably slower compared to the native installation when working with the large amount of files.
awesomeapp
at the end to your app name):docker run --rm -it -v ${PWD}:/ws:delegated startupjs/dev init awesomeapp
./docker
yarn start
Open http://localhost:3000
and you should see your app.
./docker exec
To get this project running with all dependencies, follow steps given below:
CodePush is a cloud service that enables React Native developers to deploy mobile app updates instantly to their user's devices. Following steps will help in configuring CodePush for project.
npm install -g code-push-cli
// Register
code-push register
// Login if registered already
code-push login
// For Android
code-push app add <App-Name-Android> android react-native
// For iOS
code-push app add <App-Name-Ios> ios react-native
For Android
reactNativeCodePush_androidDeploymentKey
string item to /path_to_your_app/android/app/src/main/res/values/strings.xml
. It may looks like this:<resources>
<string name="reactNativeCodePush_androidDeploymentKey" moduleConfig="true"></string>
<string name="app_name">Lingua.Live</string>
</resources>
code-push deployment ls <App-Name-Android> --displayKeys
and copy both Debug and Release key in /path_to_your_app/android/app/build.gradle
/path_to_your_app/android/app/src/main/java/com/lingua/MainApplication.java
and add code which set keys. It may looks like this:@Override
protected List<ReactPackage> getPackages() {
@SuppressWarnings("UnnecessaryLocalVariable")
List<ReactPackage> packages = new PackageList(this).getPackages();
// Set CodePush deployment keys here, because
// we can't set different keys for debug and
// release on strings.xml (reactNativeCodePush_androidDeploymentKey)
for(ReactPackage reactPackage: packages) {
if (reactPackage instanceof CodePush) {
((CodePush)reactPackage).setDeploymentKey(BuildConfig.CODEPUSH_KEY);
}
}
return packages;
}
For iOS
CodePushDeploymentKey
string item with value $(CODEPUSH_KEY)
to /path_to_your_app/ios/your_app/Info.plist
. It may looks like this:<plist version="1.0">
<dict>
<!-- ...other configs... -->
<key>CFBundleVersion</key>
<string>1</string>
<key>CodePushDeploymentKey</key>
<string>$(CODEPUSH_KEY)</string>
<key>LSRequiresIPhoneOS</key>
<!-- ...other configs... -->
</dict>
</plist>
/path_to_your_app/ios
using Xcode
and copy both Debug and Release key inIf you have any problem, search for the issues in this repository. If you don't find anything, you can raise an issue here.
(MIT)
FAQs
React Native + Web + Node.js full-stack framework
The npm package startupjs receives a total of 126 weekly downloads. As such, startupjs popularity was classified as not popular.
We found that startupjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.