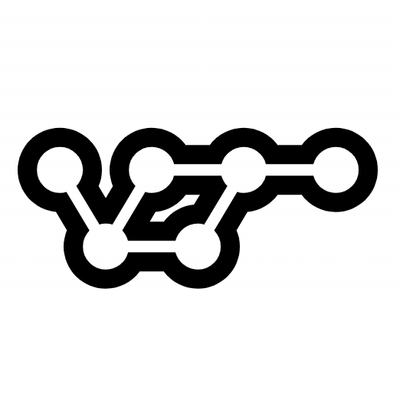
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
npm i steamapi
Once signed into Steam, head over to http://steamcommunity.com/dev/apikey to make an API key
First, start by making a new SteamAPI "user"
const SteamAPI = require('steamapi');
const steam = new SteamAPI('steam token');
Now, you can call methods to the steam
object
For example, lets get the SteamID64 of a user. SteamAPI provides a resolve
method which allows urls/id/profile
steam.resolve('https://steamcommunity.com/id/DimGG').then(id => {
console.log(id); // 76561198146931523
});
Now let's take that ID and get their profile
steam.getUserSummary('76561198146931523').then(summary => {
console.log(summary);
/**
PlayerSummary {
avatar: {
small: 'https://steamcdn-a.akamaihd.net/steamcommunity/public/images/avatars/7f/7fdf55394eb5765ef6f7be3b1d9f834fa9c824e8.jpg',
medium: 'https://steamcdn-a.akamaihd.net/steamcommunity/public/images/avatars/7f/7fdf55394eb5765ef6f7be3b1d9f834fa9c824e8_medium.jpg',
large: 'https://steamcdn-a.akamaihd.net/steamcommunity/public/images/avatars/7f/7fdf55394eb5765ef6f7be3b1d9f834fa9c824e8_full.jpg'
},
steamID: '76561198146931523',
url: 'http://steamcommunity.com/id/DimGG/',
created: 1406393110,
lastLogOff: 1517725233,
nickname: 'Dim',
primaryGroupID: '103582791457347196',
personaState: 1,
personaStateFlags: 0,
commentPermission: 1,
visibilityState: 3
}
*/
});
FAQs
A nice Steam API wrapper.
We found that steamapi demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.