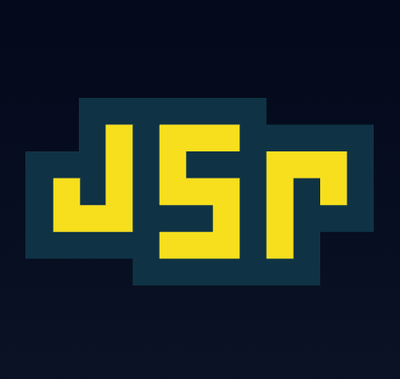
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Container for mealy state machines
From idle
to fetching
by using fetch data
action.
// Just pass the new state as a handler for an action
Machine.create('app', {
'idle': {
'fetch data': 'fetching'
}
});
// Return a string in the handler
Machine.create('app', {
'idle': {
'fetch data': function () {
return 'fetching';
}
}
});
// Return a state object
Machine.create('app', {
'idle': {
'fetch data': function () {
return { name: 'fetching' };
}
}
});
// Yield a string in the handler's generator
Machine.create('app', {
'idle': {
'fetch data': function * () {
yield 'fetching';
// or you can yield a state object
// `yield { name: 'fetching' }`
}
}
});
For the cases where you want to do something as a result of two (or more) actions. From idle
state to done
when fetching in progress
and success
(or fail
) actions are dispatched.
Machine.create('app', {
'idle': {
'fetching in progress': function * () {
const [ data, error ] = yield wait(['success', 'fail']);
// or just `const data = yield wait('success')`
// if we are interested only in one action
return data ? { name: 'done', data } : { name: 'done', error };
}
}
});
This example is a bit silly. We'll probably go with a separate state when data fetching is in progress.
import { Machine } from 'mealy';
const machine = Machine.create('app', {
state: { name: 'idle', todos: [] },
transitions: {
'idle': {
'add new todo': function ({ todos }, todo) {
todos.push(todo);
return { todos };
},
'delete todo': function ({ todos }, index) {
return { todos: todos.splice(index, 1) };
},
'fetch todos': function * () {
yield 'fetching';
try {
const todos = yield call(getTodos, '/api/todos');
} catch (error) {
return { name: 'fetching failed', error };
}
return { name: 'idle', todos };
}
},
'fetching failed': {
'fetch todos': function * () {
yield { name: 'idle', error: null };
this.fetchTodos();
}
}
}
});
machine.fetchTodos();
import React from 'react';
import { connect } from 'mealy/react';
class TodoList extends React.Component {
render() {
const { todos, error, isFetching, fetchTodos, deleteTodo } = this.props;
if (isFetching()) return <p>Loading</p>;
if (error) return (
<div>
Error fetching todos: { error }<br />
<button onClick={ fetchTodos }>try again</button>
</div>
);
return (
<ul>
{ todos.map(({ text}) => <li onClick={ deleteTodo }>{ text }</li>) }
</ul>
);
}
}
export default connect(TodoList)
.with('app')
.map(({ state, isFetching, fetchTodos, deleteTodo } => {
todos: state.todos,
error: state.error,
isFetching,
fetchTodos,
deleteTodo
}));
FAQs
Stent is combining the ideas of redux with the concept of state machines
We found that stent demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.