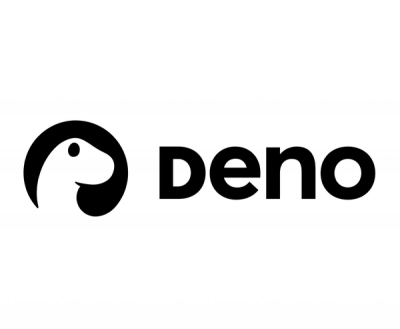
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
stream-chat
Advanced tools
The stream-chat npm package is a powerful tool for building chat applications. It provides a comprehensive set of features for real-time messaging, user management, and chat room creation, among others. It is designed to be highly customizable and scalable, making it suitable for a wide range of applications from simple chat widgets to complex messaging platforms.
Real-time Messaging
This feature allows you to send real-time messages in a chat channel. The code sample demonstrates how to initialize the StreamChat client, create a channel, and send a message.
const StreamChat = require('stream-chat').StreamChat;
const client = new StreamChat('api_key');
async function sendMessage() {
const channel = client.channel('messaging', 'general', {
name: 'General Chat',
});
await channel.create();
await channel.sendMessage({
text: 'Hello, world!',
user_id: 'user123',
});
}
sendMessage();
User Management
This feature allows you to manage users within the chat application. The code sample demonstrates how to create or update a user with specific attributes.
const StreamChat = require('stream-chat').StreamChat;
const client = new StreamChat('api_key');
async function createUser() {
await client.upsertUser({
id: 'user123',
name: 'John Doe',
role: 'user',
});
}
createUser();
Channel Management
This feature allows you to manage chat channels. The code sample demonstrates how to create a new chat channel with a specific name and type.
const StreamChat = require('stream-chat').StreamChat;
const client = new StreamChat('api_key');
async function createChannel() {
const channel = client.channel('messaging', 'general', {
name: 'General Chat',
});
await channel.create();
}
createChannel();
Message Reactions
This feature allows users to add reactions to messages. The code sample demonstrates how to send a message and then add a 'like' reaction to that message.
const StreamChat = require('stream-chat').StreamChat;
const client = new StreamChat('api_key');
async function addReaction() {
const channel = client.channel('messaging', 'general');
await channel.create();
const message = await channel.sendMessage({
text: 'Hello, world!',
user_id: 'user123',
});
await channel.sendReaction(message.id, {
type: 'like',
user_id: 'user123',
});
}
addReaction();
Socket.IO is a library that enables real-time, bidirectional and event-based communication between web clients and servers. While it is more general-purpose and can be used for various real-time applications, it requires more manual setup for chat-specific features compared to stream-chat.
Pusher is a service that provides APIs for building real-time web and mobile applications. Pusher Channels specifically can be used for real-time messaging, but it lacks some of the higher-level abstractions and features specifically tailored for chat applications that stream-chat offers.
Firebase is a platform developed by Google for creating mobile and web applications. Firebase Realtime Database and Firestore can be used to build real-time chat applications. However, Firebase is a more general-purpose backend-as-a-service and may require more custom development to achieve the same level of chat-specific functionality provided by stream-chat.
FAQs
JS SDK for the Stream Chat API
The npm package stream-chat receives a total of 146,024 weekly downloads. As such, stream-chat popularity was classified as popular.
We found that stream-chat demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.