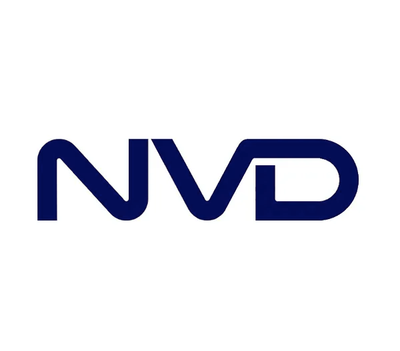
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
stream-collect
Advanced tools
Collects a readable streams data as a string buffer or, for object streams, an array.
npm install stream-collect
Collect the contents of a stream. The collected data will either be a Buffer
, String
or Array
depending on whether encoding
has been supplied, or it is an object stream.
Returns a Promise
that will resolve with the collected data.
stream
the stream to collectencoding
the encoding to return data incb
a callback that will be called with the collected data.var collect = require('stream-collect');
var file = fs.createReadableStream( 'myfile' );
// Collect using a promise
collect(file)
.then( function(theWholeFile) {
// Do something
} );
// Collect using a callback
collect( file, function(theWholeFile) {
// Do something
} )
// Specify an encoding
collect( file, 'base64' )
.then( function(theWholeFileInBase64) {
// Do something
} );
A PassThrough stream that has been augmented with a collect event. This will be emitted in the end
event with the collected contents of the stream.
var collect = require('stream-collect');
var file = fs.createReadableStream( 'myfile' );
file.pipe( new collect.PassThrough() )
.on( 'collect', function(data) {
// data = contents of the file
} );
Augment any stream with the collect event used on collect.PassThrough
.
Returns the augmented stream.
FAQs
Collects the output of a stream
The npm package stream-collect receives a total of 46 weekly downloads. As such, stream-collect popularity was classified as not popular.
We found that stream-collect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.