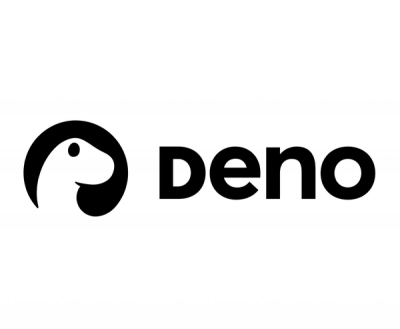
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
stream-forward
Advanced tools
Forward events to the next stream in the pipeline.
$ npm install --save stream-forward
var streamForward = require('stream-forward');
var streamForward = require('stream-forward');
var request = require('request');
var opts = {
events: ['response']
};
streamForward(request('http://yahoo.com'), opts)
.pipe(process.stdout)
.on('response', function (response) {
// `response` from the request stream.
});
Note: don't neglect proper event handling on the individual parts of your stream.
This is just a convenience when you have to manually listen and re-emit events across a middleman/spy pipe. Consider the following example:
var fs = require('fs');
function getRequestStream(reqOpts) {
var opts = {
events: ['complete']
};
return streamForward(request(reqOpts), opts)
.pipe(fs.createWriteStream('./request-cache'));
}
getRequestStream('http://yahoo.com')
.on('complete', function () {
// Without `stream-forward`, this couldn't emit.
});
Type: Stream
The source stream to spy on. This is returned from the function to allow chaining.
Optional. Configuration options.
Type: Boolean
Default: false
If true, when a new stream is attached to stream
, it will receive the events emitted by the original.
var sourceStream = request('http://yahoo.com');
var through = require('through2');
streamForward(sourceStream)
.pipe(through())
.on('complete', function () {
// Called.
})
.pipe(through())
.on('complete', function () {
// Not called.
});
var sourceStream = request('http://yahoo.com');
var through = require('through2');
streamForward(sourceStream, { continuous: true })
.pipe(through())
.on('complete', function () {
// Called.
})
.pipe(through())
.on('complete', function () {
// Called.
});
Type: Array
Event names to re-emit on attached streams.
FAQs
Forward stream events.
We found that stream-forward demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.