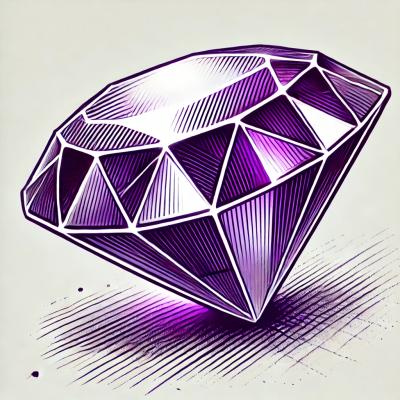
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
stream-http
Advanced tools
The stream-http npm package is designed to provide a streaming HTTP client for browser-based applications that mimics node's native http module, allowing for code that uses http to be more easily ported to the browser.
HTTP GET request
This feature allows you to perform an HTTP GET request and stream the response data as it is received.
const http = require('stream-http');
http.get('http://example.com', function (response) {
response.on('data', function (chunk) {
console.log('BODY: ' + chunk);
});
});
HTTP request with options
This feature allows you to perform an HTTP request with custom options such as method, path, and headers, and to send data in the body of the request.
const http = require('stream-http');
const options = {
method: 'POST',
path: '/submit',
headers: {'Content-Type': 'application/json'}
};
const req = http.request(options, function (response) {
console.log('STATUS: ' + response.statusCode);
response.on('data', function (chunk) {
console.log('BODY: ' + chunk);
});
});
req.write(JSON.stringify({ key: 'value' }));
req.end();
Handling request errors
This feature demonstrates how to handle errors that may occur during an HTTP request.
const http = require('stream-http');
http.get('http://example.com', function (response) {
response.on('data', function (chunk) {
console.log('BODY: ' + chunk);
});
}).on('error', function (e) {
console.error('Request failed: ' + e.message);
});
Axios is a promise-based HTTP client for the browser and node.js. It provides a simple API for performing HTTP requests and is often preferred for its ease of use and promise support compared to stream-http.
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It is built into modern browsers and offers a more modern, promise-based approach to asynchronous HTTP requests compared to stream-http.
Superagent is a small progressive client-side HTTP request library that offers a simple, fluent API. It is often used for its chaining syntax and built-in support for JSON, which can make it more convenient than stream-http for certain use cases.
Got is a human-friendly and powerful HTTP request library for Node.js. It is designed to be a simpler and more robust alternative to the built-in http module, with features like retries and streams, which can make it a more feature-rich alternative to stream-http.
This module is an implementation of node's native http
module for the browser.
It tries to match node's api and behavior as closely as possible, but some features
aren't available, since browsers don't give nearly as much control over requests.
This is heavily inspired by, and intended to replace, http-browserify
In accordance with its name, stream-http
tries to provide data to its caller before
the request has completed whenever possible.
The following browsers support true streaming, where only a small amount of the request has to be held in memory at once:
fetch
api)moz-chunked-arraybuffer
responseType with xhr)The following browsers support pseudo-streaming, where the data is available before the request finishes, but the entire response must be held in memory:
All browsers newer than IE8 support binary responses. All of the above browsers that support true streaming or pseudo-streaming support that for binary data as well except for IE10. Old (presto-based) Opera also does not support binary streaming either.
As of version 2.0.0, IE8 support requires the user to supply polyfills for
Object.keys
, Array.prototype.forEach
, and Array.prototype.indexOf
. Example
implementations are provided in ie8-polyfill.js; alternately,
you may want to consider using es5-shim.
All browsers with full ES5 support shouldn't require any polyfills.
The intent is to have the same api as the client part of the node HTTP module. The interfaces are the same wherever practical, although limitations in browsers make an exact clone of the node api impossible.
This module implements http.request
, http.get
, and most of http.ClientRequest
and http.IncomingMessage
in addition to http.METHODS
and http.STATUS_CODES
. See the
node docs for how these work.
The message.url
property provides access to the final url after all redirects. This
is useful since the browser follows all redirects silently, unlike node. It is available
in Chrome 37 and newer, Firefox 32 and newer, and Safari 9 and newer.
The options.withCredentials
boolean flag, used to indicate if the browser should send
cookies or authentication information with a CORS request. Default false.
This module has to make some tradeoffs to support binary data and/or streaming. Generally, the module can make a fairly good decision about which underlying browser features to use, but sometimes it helps to get a little input from the user.
options.mode
field passed into http.request
or http.get
can take on one of the
following values:
http.Agent
is only a stubhttp.ClientRequest
.request.setTimeout
, that operate directly on the underlying
socket.message.httpVersion
message.rawHeaders
is modified by the browser, and may not quite match what is sent by
the server.message.trailers
and message.rawTrailers
will remain empty.http.get('/bundle.js', function (res) {
var div = document.getElementById('result');
div.innerHTML += 'GET /beep<br>';
res.on('data', function (buf) {
div.innerHTML += buf;
});
res.on('end', function () {
div.innerHTML += '<br>__END__';
});
})
There are two sets of tests: the tests that run in node (found in test/node
) and the tests
that run in the browser (found in test/browser
). Normally the browser tests run on
Sauce Labs.
Running npm test
will run both sets of tests, but in order for the Sauce Labs tests to run
you will need to sign up for an account (free for open source projects) and put the
credentials in a .zuulrc
file.
To run just the node tests, run npm run test-node
.
To run the browser tests locally, run npm run test-browser-local
and point your browser to
http://localhost:8080/__zuul
MIT. Copyright (C) John Hiesey and other contributors.
FAQs
Streaming http in the browser
We found that stream-http demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.