Stream Mock

Mock nodejs streams.
Features
Quick start
yarn add --dev stream-mock
Or, if you are more a npm
person
npm -i -D stream-mock
Basic usage
You are building an awesome brand new Transform stream that rounds all your values.
import { Transform } from 'stream';
export default class Rounder extends Transform {
_transform(chunk, encoding, callback) {
this.push(Math.round(chunk));
callback();
}
}
Now you need / want to test it.
import {ReadableMock, WritableMock } from 'stream-mock';
import chai from 'chai';
import Rounder from 'the/seven/bloody/hells';
chai.should();
describe('Test me if you can', (done) => {
it('Round me like one of your french girls', {
const input = [1.2, 2.6, 3.7];
const reader = new ReadableMock(input, {objectMode: true});
const writer = new WritableMock({objectMode: true});
const transform = new Rounder({objectMode: true});
reader.pipe(transform).pipe(writer);
writer.on('finish', ()=>{
writer.data.should.deep.equal(input.map(Math.round));
})
});
});
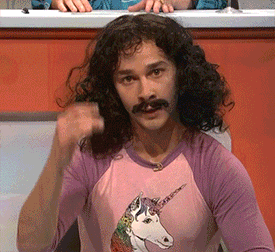
API documentation
Full API doc is hosted here
Contributing
stream-mock is currently on early stage.
I'm happily receiving new PR or discussing about new features,
improvement or bug report.
If you need something that this module is lacking
(related to Nodejs stream mock obviously), do not hesitate to raise an issue.
Developper side
If you have to write code in that repository,
please be kind to run unit tests and lint before pushing.
Yeah the linter is quite strict, but it's for the best !
yarn run test
yarn run lint
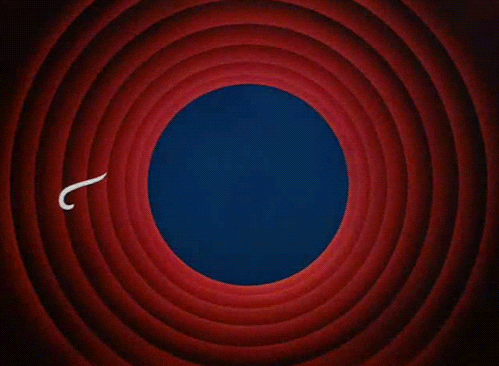
License
MIT