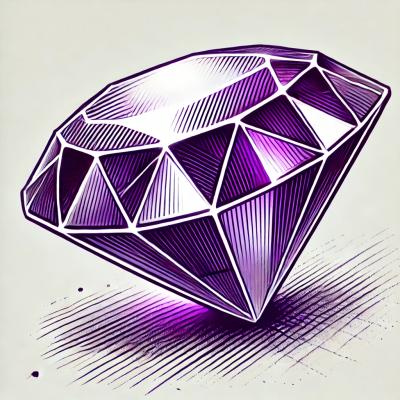
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
string-kit
Advanced tools
A string manipulation toolbox, featuring a string formater (inspired by printf), a variable inspector (output featuring ANSI color and HTML) and various escape function (shell argument, regexp, html, etc).
string-kit is a versatile JavaScript library for string manipulation. It provides a wide range of utilities for working with strings, including formatting, padding, alignment, and more.
String Formatting
The `format` function allows you to format strings using placeholders, similar to printf in C. This is useful for creating dynamic strings based on variable values.
const string = require('string-kit');
console.log(string.format('%s is %d years old.', 'Alice', 30));
String Padding
The `pad` function pads a string to a specified length with a given character. This is useful for aligning text in console output or creating fixed-width string representations.
const string = require('string-kit');
console.log(string.pad('Hello', 10, ' '));
String Alignment
The `align` function aligns a string within a specified width, either left, right, or center. This is useful for formatting text in tables or other structured formats.
const string = require('string-kit');
console.log(string.align('Hello', 10, 'center', ' '));
String Truncation
The `truncate` function shortens a string to a specified length, optionally adding an ellipsis or other suffix. This is useful for displaying previews or summaries of longer text.
const string = require('string-kit');
console.log(string.truncate('This is a long string', 10));
The `string` package provides a wide range of string manipulation utilities, including trimming, padding, and formatting. It is similar to string-kit but offers a different API and some additional features.
Lodash is a utility library that provides a wide range of functions for working with arrays, objects, and strings. It includes string manipulation functions like padding, truncation, and case conversion. While it is more general-purpose than string-kit, it offers similar string manipulation capabilities.
underscore.string is a string manipulation library that extends Underscore.js with additional string functions. It provides utilities for trimming, padding, formatting, and more. It is similar to string-kit but designed to work seamlessly with Underscore.js.
A string manipulation toolbox, featuring a string formater (inspired by printf), a variable inspector (output featuring ANSI color and HTML) and various escape function (shell argument, regexp, html, etc).
Use Node Package Manager:
npm install string-kit
String
the string to filterIt escapes the string so that it will be suitable as a shell command's argument.
String
the string to filterIt escapes the string so that it will be suitable to inject it in a regular expression's pattern as a literal string.
Example of a search and replace from a user's input:
var result = data.replace(
new RegExp( stringKit.escape.regExp( userInputSearch ) , 'g' ) ,
stringKit.escape.regExpReplacement( userInputReplace )
) ;
String
the string to filterIt escapes the string so that it will be suitable as a literal string for a regular expression's replacement.
String
the string to filterIt escapes the string so that it will be suitable as HTML content.
Only < > &
are replaced by HTML entities.
String
the string to filterIt escapes the string so that it will be suitable as an HTML tag attribute's value.
Only < > & "
are replaced by HTML entities.
It assumes valid HTML: the attribute's value should be into double quote, not in single quote.
String
the string to filterIt escapes all HTML special characters, < > & " '
are replaced by HTML entities.
String
the string to filterIt escapes all ASCII control characters (code lesser than or equals to 0x1F, or backspace).
Carriage return, newline and tabulation are respectively replaced by \r
, \n
and \t
.
Other characters are replaced by the unicode notation, e.g. NUL
is replaced by \x00
.
Full BDD spec generated by Mocha:
should perform basic examples.
expect( format( 'Hello world' ) ).to.be( 'Hello world' ) ;
expect( format( 'Hello %s' , 'world' ) ).to.be( 'Hello world' ) ;
expect( format( 'Hello %s %s, how are you?' , 'Joe' , 'Doe' ) ).to.be( 'Hello Joe Doe, how are you?' ) ;
expect( format( 'I have %i cookies.' , 3 ) ).to.be( 'I have 3 cookies.' ) ;
%u should format unsigned integer.
expect( format( '%u' , 123 ) ).to.be( '123' ) ;
expect( format( '%u' , 0 ) ).to.be( '0' ) ;
expect( format( '%u' , -123 ) ).to.be( '0' ) ;
expect( format( '%u' ) ).to.be( '0' ) ;
%U should format positive unsigned integer.
expect( format( '%U' , 123 ) ).to.be( '123' ) ;
expect( format( '%U' , 0 ) ).to.be( '1' ) ;
expect( format( '%U' , -123 ) ).to.be( '1' ) ;
expect( format( '%U' ) ).to.be( '1' ) ;
should perform well the argument's number feature.
expect( format( '%s%s%s' , 'A' , 'B' , 'C' ) ).to.be( 'ABC' ) ;
expect( format( '%+1s%-1s%s' , 'A' , 'B' , 'C' ) ).to.be( 'BAC' ) ;
expect( format( '%3s%s' , 'A' , 'B' , 'C' ) ).to.be( 'CBC' ) ;
format.count() should count the number of arguments found.
expect( format.count( 'blah blih blah' ) ).to.be( 0 ) ;
expect( format.count( '%i %s' ) ).to.be( 2 ) ;
when using a filter object as the this context, the %[functionName] format should use a custom function to format the input.
var filters = {
fixed: function() { return 'F' ; } ,
double: function( str ) { return '' + str + str ; } ,
fxy: function( a , b ) { return '' + ( a * a + b ) ; }
} ;
expect( format.call( filters , '%[fixed]%s%s%s' , 'A' , 'B' , 'C' ) ).to.be( 'FABC' ) ;
expect( format.call( filters , '%s%[fxy:%a%a]' , 'f(x,y)=' , 5 , 3 ) ).to.be( 'f(x,y)=28' ) ;
expect( format.call( filters , '%s%[fxy:%+1a%-1a]' , 'f(x,y)=' , 5 , 3 ) ).to.be( 'f(x,y)=14' ) ;
escape.control() should escape control characters.
expect( string.escape.control( 'Hello\n\t... world!' ) ).to.be( 'Hello\\n\\t... world!' ) ;
expect( string.escape.control( 'Hello\\n\\t... world!' ) ).to.be( 'Hello\\n\\t... world!' ) ;
expect( string.escape.control( 'Hello\\\n\\\t... world!' ) ).to.be( 'Hello\\\\n\\\\t... world!' ) ;
expect( string.escape.control( 'Hello\\\\n\\\\t... world!' ) ).to.be( 'Hello\\\\n\\\\t... world!' ) ;
expect( string.escape.control( 'Nasty\x00chars\x1bhere\x7f!' ) ).to.be( 'Nasty\\x00chars\\x1bhere\\x7f!' ) ;
escape.shellArg() should escape a string so that it will be suitable as a shell command's argument.
//console.log( 'Shell arg:' , string.escape.shellArg( "Here's my shell's argument" ) ) ;
expect( string.escape.shellArg( "Here's my shell's argument" ) ).to.be( "'Here'\\''s my shell'\\''s argument'" ) ;
escape.regExp() should escape a string so that it will be suitable as a literal string into a regular expression pattern.
//console.log( 'String in RegExp:' , string.escape.regExp( "(This) {is} [my] ^$tring^... +doesn't+ *it*? |yes| \\no\\ /maybe/" ) ) ;
expect( string.escape.regExp( "(This) {is} [my] ^$tring^... +doesn't+ *it*? |yes| \\no\\ /maybe/" ) )
.to.be( "\\(This\\) \\{is\\} \\[my\\] \\^\\$tring\\^\\.\\.\\. \\+doesn't\\+ \\*it\\*\\? \\|yes\\| \\\\no\\\\ \\/maybe\\/" ) ;
escape.regExpReplacement() should escape a string so that it will be suitable as a literal string into a regular expression replacement.
expect( string.escape.regExpReplacement( "$he love$ dollar$ $$$" ) ).to.be( "$$he love$$ dollar$$ $$$$$$" ) ;
expect(
'$he love$ dollar$ $$$'.replace(
new RegExp( string.escape.regExp( '$' ) , 'g' ) ,
string.escape.regExpReplacement( '$1' )
)
).to.be( "$1he love$1 dollar$1 $1$1$1" ) ;
escape.html() should escape a string so that it will be suitable as HTML content.
//console.log( string.escape.html( "<This> isn't \"R&D\"" ) ) ;
expect( string.escape.html( "<This> isn't \"R&D\"" ) ).to.be( "<This> isn't \"R&D\"" ) ;
escape.htmlAttr() should escape a string so that it will be suitable as an HTML tag attribute's value.
//console.log( string.escape.htmlAttr( "<This> isn't \"R&D\"" ) ) ;
expect( string.escape.htmlAttr( "<This> isn't \"R&D\"" ) ).to.be( "<This> isn't "R&D"" ) ;
escape.htmlSpecialChars() should escape all HTML special characters.
//console.log( string.escape.htmlSpecialChars( "<This> isn't \"R&D\"" ) ) ;
expect( string.escape.htmlSpecialChars( "<This> isn't \"R&D\"" ) ).to.be( "<This> isn't "R&D"" ) ;
should inspect a variable with default options accordingly.
var MyClass = function MyClass() {
this.variable = 1 ;
} ;
MyClass.prototype.report = function report() { console.log( 'Variable value:' , this.variable ) ; } ;
MyClass.staticFunc = function staticFunc() { console.log( 'Static function.' ) ; } ;
var sparseArray = [] ;
sparseArray[ 3 ] = 'three' ;
sparseArray[ 10 ] = 'ten' ;
sparseArray[ 20 ] = 'twenty' ;
sparseArray.customProperty = 'customProperty' ;
var object = {
a: 'A' ,
b: 2 ,
str: 'Woot\nWoot\rWoot\tWoot' ,
sub: {
u: undefined ,
n: null ,
t: true ,
f: false
} ,
emptyString: '' ,
emptyObject: {} ,
list: [ 'one','two','three' ] ,
emptyList: [] ,
sparseArray: sparseArray ,
hello: function hello() { console.log( 'Hello!' ) ; } ,
anonymous: function() { console.log( 'anonymous...' ) ; } ,
class: MyClass ,
instance: new MyClass() ,
buf: new Buffer( 'This is a buffer!' )
} ;
object.sub.circular = object ;
Object.defineProperties( object , {
c: { value: '3' } ,
d: {
get: function() { throw new Error( 'Should not be called by the test' ) ; } ,
set: function( value ) {}
}
} ) ;
//console.log( '>>>>>' , string.escape.control( string.inspect( object ) ) ) ;
//console.log( string.inspect( { style: 'color' } , object ) ) ;
expect( string.inspect( object ) ).to.be( '<Object> <object> {\n a: "A" <string>(1)\n b: 2 <number>\n str: "Woot\\nWoot\\rWoot\\tWoot" <string>(19)\n sub: <Object> <object> {\n u: undefined\n n: null\n t: true\n f: false\n circular: <Object> <object> [circular]\n }\n emptyString: "" <string>(0)\n emptyObject: <Object> <object> {}\n list: <Array>(3) <object> {\n [0] "one" <string>(3)\n [1] "two" <string>(3)\n [2] "three" <string>(5)\n length: 3 <number> <-conf -enum>\n }\n emptyList: <Array>(0) <object> {\n length: 0 <number> <-conf -enum>\n }\n sparseArray: <Array>(21) <object> {\n [3] "three" <string>(5)\n [10] "ten" <string>(3)\n [20] "twenty" <string>(6)\n length: 21 <number> <-conf -enum>\n customProperty: "customProperty" <string>(14)\n }\n hello: <Function> hello(0) <function>\n anonymous: <Function> (anonymous)(0) <function>\n class: <Function> MyClass(0) <function>\n instance: <MyClass> <object> {\n variable: 1 <number>\n }\n buf: <Buffer 54 68 69 73 20 69 73 20 61 20 62 75 66 66 65 72 21> <Buffer>(17)\n c: "3" <string>(1) <-conf -enum -w>\n d: <getter/setter> {\n get: <Function> (anonymous)(0) <function>\n set: <Function> (anonymous)(1) <function>\n }\n}\n' ) ;
FAQs
A string manipulation toolbox, featuring a string formatter (inspired by sprintf), a variable inspector (output featuring ANSI colors and HTML) and various escape functions (shell argument, regexp, html, etc).
The npm package string-kit receives a total of 53,087 weekly downloads. As such, string-kit popularity was classified as popular.
We found that string-kit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.