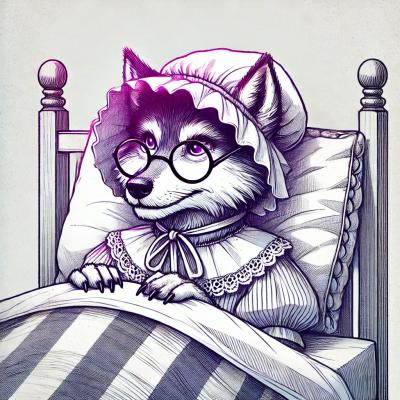
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The Stripe npm package is a library that provides a powerful and easy-to-use interface to the Stripe API, allowing developers to integrate payment processing into their Node.js applications. It supports a wide range of payment operations, from charging credit cards to managing subscriptions and handling disputes.
Charging a Credit Card
This feature allows you to create a charge on a credit card. The amount is specified in the smallest currency unit (e.g., cents for USD).
stripe.charges.create({
amount: 2000,
currency: 'usd',
source: 'tok_amex',
description: 'Charge for jenny.rosen@example.com'
}).then(function(charge) {
// asynchronously called
});
Creating a Customer
This feature enables you to create a new customer object, which can be used for recurring charges and tracking multiple charges that are associated with the same customer.
stripe.customers.create({
email: 'customer@example.com'
}).then(function(customer) {
// asynchronously called
});
Managing Subscriptions
This feature allows you to create and manage subscriptions for recurring payments. You can specify the plan and customer to associate with the subscription.
stripe.subscriptions.create({
customer: 'cus_4fdAW5ftNQow1a',
items: [{
plan: 'plan_CBXbz9i7AIOTzr'
}]
}).then(function(subscription) {
// asynchronously called
});
Handling Webhooks
This feature is for setting up a webhook endpoint to listen for events from Stripe. This is useful for receiving notifications about various events, such as successful payments or subscription cancellations.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.post('/webhook', bodyParser.raw({type: 'application/json'}), (request, response) => {
let event;
try {
event = JSON.parse(request.body);
} catch (err) {
response.status(400).send(`Webhook Error: ${err.message}`);
return;
}
// Handle the event
switch (event.type) {
case 'payment_intent.succeeded':
const paymentIntent = event.data.object;
console.log(`PaymentIntent was successful!`);
break;
// ... handle other event types
default:
console.log(`Unhandled event type ${event.type}`);
}
response.status(200).end();
});
app.listen(8000, () => {
console.log('Running on port 8000');
});
Braintree is a full-stack payment platform that makes it easy to accept payments in your app or website. It offers similar functionalities to Stripe, including payment processing, subscription management, and fraud protection. Braintree is known for its PayPal integration, which can be a deciding factor for some businesses.
Square Connect is the official Square npm package. It provides access to various Square services, including payment processing. While it offers similar features to Stripe, such as handling transactions and managing customers, it is particularly tailored for businesses that use Square's point of sale system.
Mollie is a payment service provider that offers an easy-to-implement process for integrating payments into a website or app. It supports various payment methods and is known for its simplicity. However, it might not have as extensive a feature set as Stripe, particularly in terms of global reach and customization options.
Read about Version 2 here (Released October 18th, 2013)
npm install stripe
Documentation is available at https://stripe.com/docs/api/node.
Every resource is accessed via your stripe
instance:
var stripe = require('stripe')(' your stripe API key ');
// stripe.{ RESOURCE_NAME }.{ METHOD_NAME }
Every resource method accepts an optional callback as the last argument:
stripe.customers.create(
{ email: 'customer@example.com' },
function(err, customer) {
err; // null if no error occurred
customer; // the created customer object
}
);
Additionally, every resource method returns a promise, so you don't have to use the regular callback. E.g.
// Create a new customer and then a new charge for that customer:
stripe.customers.create({
email: 'foo-customer@example.com'
}).then(function(customer) {
return stripe.charges.create({
amount: 1600,
currency: 'usd',
customer: customer.id
});
}).then(function(charge) {
// New charge created on a new customer
}, function(err) {
// Deal with an error
});
Where you see params
it is a plain JavaScript object, e.g. { email: 'foo@example.com' }
retrieve()
retrieve()
listTransactions([params])
retrieveTransaction(transactionId)
create(params)
list([params])
retrieve(chargeId)
capture(chargeId[, params])
refund(chargeId[, params])
update(chargeId[, params])
updateDispute(chargeId[, params])
closeDispute(chargeId[, params])
setMetadata(chargeId, metadataObject)
(metadata info)setMetadata(chargeId, key, value)
getMetadata(chargeId)
create(params)
list([params])
retrieve(chargeId)
del(chargeId)
create(params)
list([params])
update(customerId[, params])
retrieve(customerId)
del(customerId)
setMetadata(customerId, metadataObject)
(metadata info)setMetadata(customerId, key, value)
getMetadata(customerId)
updateSubscription(customerId, subscriptionId, [, params])
cancelSubscription(customerId, subscriptionId, [, params])
createCard(customerId[, params])
listCards(customerId)
retrieveCard(customerId, cardId)
updateCard(customerId, cardId[, params])
deleteCard(customerId, cardId)
deleteDiscount(customerId)
list([params])
retrieve(eventId)
create(params)
list([params])
update(invoiceItemId[, params])
retrieve(invoiceItemId)
del(invoiceItemId)
create(params)
list([params])
update(invoiceId[, params])
retrieve(invoiceId)
retrieveLines(invoiceId)
retrieveUpcoming(customerId[, subscriptionId])
pay(invoiceId)
create(params)
list([params])
update(planId[, params])
retrieve(planId)
del(planId)
create(params)
list([params])
update(recipientId[, params])
retrieve(recipientId)
del(recipientId)
setMetadata(recipientId, metadataObject)
(metadata info)setMetadata(recipientId, key, value)
getMetadata(recipientId)
create(params)
retrieve(tokenId)
create(params)
list([params])
retrieve(transferId)
update(transferId[, params])
cancel(transferId)
listTransactions(transferId[, params])
setMetadata(transferId, metadataObject)
(metadata info)setMetadata(transferId, key, value)
getMetadata(transferId)
stripe.setApiKey(' your secret api key ');
stripe.setTimeout(20000); // in ms
(default is node's default: 120000ms
)To run the tests you'll need a Stripe Test API key (from your Stripe Dashboard):
$ npm install -g mocha
$ npm test
Note: On Windows use SET
instead of export
for setting the STRIPE_TEST_API_KEY
environment variable.
If you don't have a prefixed key (in the form sk_test_...
) you can get one by rolling your "Test Secret Key". This can be done under your dashboard (Account Setting -> API Keys -> Click the roll icon next to the "test secret key"). This should give you a new prefixed key ('sk_test_..'), which will then be usable by the node mocha tests.
Originally by Ask Bjørn Hansen (ask@develooper.com). Development was sponsored by YellowBot. Now officially maintained by Stripe.
2.9.0 - 2014-11-12
FAQs
Stripe API wrapper
The npm package stripe receives a total of 909,216 weekly downloads. As such, stripe popularity was classified as popular.
We found that stripe demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.