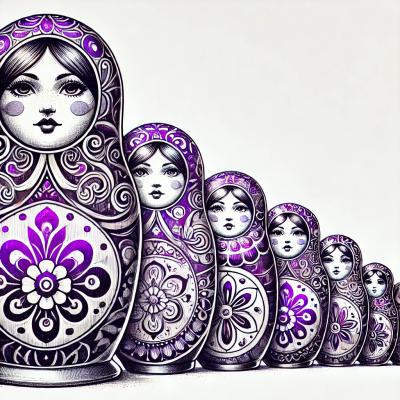
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
styled-breakpoints
Advanced tools
Simple and powerful css breakpoints for styled-components and emotion
Breakpoints serve as adjustable widths that determine the behavior of your responsive layout across different device or viewport sizes.
For own components.
const Box = styled.div`
background-color: pink;
${({ theme }) => theme.breakpoints.up('sm')} {
background-color: hotpink;
}
${({ theme }) => theme.breakpoints.up('md')} {
background-color: red;
}
`;
For third party components.
const Layout = () => {
const isMd = useMediaQuery(useTheme()?.breakpoints.up('md'));
return <>{isMd && <Box />}</>;
};
From smallest to largest
From largest to smallest
Breakpoints act as the fundamental elements of responsive design. They enable you to control when your layout can adapt to a specific viewport or device size.
Utilize media queries to structure your CSS based on breakpoints. Media queries are CSS features that allow you to selectively apply styles depending on a defined set of browser and operating system parameters. The most commonly used media query property is min-width
.
The objective is mobile-first, responsive design. Styled Breakpoints aims to apply the essential styles required for a layout to function at the smallest breakpoint. Additional styles are then added to adjust the design for larger devices. This approach optimizes your CSS, enhances rendering speed, and delivers an excellent user experience.
Styled Breakpoints includes six default breakpoints, often referred to as grid tiers, for building responsive designs. These breakpoints can be customized.
Each breakpoint has been carefully selected to accommodate containers with widths that are multiples of 12. The breakpoints also represent a subset of common device sizes and viewport dimensions, although they do not specifically target every use case or device. Instead, they provide a robust and consistent foundation for building designs that cater to nearly any device.
const breakpoints = {
xs: '0px',
sm: '576px',
md: '768px',
lg: '992px',
xl: '1200px',
xxl: '1400px',
};
npm install styled-components styled-breakpoints
# or
yarn add styled-components styled-breakpoints
styled.d.ts
import 'styled-components';
import { StyledBreakpointsTheme } from 'styled-breakpoints';
declare module 'styled-components' {
export interface DefaultTheme extends StyledBreakpointsTheme {}
}
app.tsx
import styled { ThemeProvider } from 'styled-components';
import { createStyledBreakpointsTheme, StyledBreakpointsTheme } from 'styled-breakpoints';
const Box = styled.div`
display: none;
${({ theme }) => theme.breakpoints.up('sm')} {
display: block;
}
`
const theme = createStyledBreakpointsTheme() as StyledBreakpointsTheme;
const App = () => (
<ThemeProvider theme={theme}>
<Box/>
</ThemeProvider>
)
npm install @emotion/{styled,react} styled-breakpoints
# or
yarn add @emotion/{styled,react} styled-breakpoints
emotion.d.ts
import '@emotion/react';
import { StyledBreakpointsTheme } from 'styled-breakpoints';
declare module '@emotion/react' {
export interface Theme extends StyledBreakpointsTheme {}
}
app.tsx
import styled from '@emotion/styled';
import { ThemeProvider } from '@emotion/react';
import {
createStyledBreakpointsTheme,
StyledBreakpointsTheme,
} from 'styled-breakpoints';
const Box = styled.div`
display: none;
${({ theme }) => theme.breakpoints.up('sm')} {
display: block;
}
`;
const theme = createStyledBreakpointsTheme() as StyledBreakpointsTheme;
const App = () => (
<ThemeProvider theme={theme}>
<Box />
</ThemeProvider>
);
The createTheme
function has been replaced with createStyledBreakpointsTheme
.
- import { createTheme } from "styled-breakpoints";
- const theme = createTheme();
+ import { createStyledBreakpointsTheme } from "styled-breakpoints";
+ const theme = createStyledBreakpointsTheme();
Additionally, the functions up
, down
, between
, and only
have been moved to the theme object. This means that you no longer need to import them individually each time you want to use them.
- import { up } from "styled-breakpoints";
- const Box = styled.div`
- ${up('md')} {
- background-color: red;
- }
+ const Box = styled.div`
+ ${({ theme }) => theme.breakpoints.up('md')} {
+ background-color: red;
+ }
`
- import { up } from 'styled-breakpoints';
- import { useBreakpoint } from 'styled-breakpoints/react-styled';
or
- import { up } from 'styled-breakpoints';
- import { useBreakpoint } from 'styled-breakpoints/react-emotion';
- const Example = () => {
- const isMd = useBreakpoint(only('md'));
-
- return <Layout>{isMd && </Box>}</Layout>
- }
+ import { useMediaQuery } from 'styled-breakpoints/use-media-query';
+ const Example = () => {
+ const isMd = useMediaQuery(useTheme()?.breakpoints.only('md'));
+
+ return <Layout>{isMd && </Box>}</Layout>
+ }
declare function up(
min: string,
orientation?: 'portrait' | 'landscape'
) => string
const Box = styled.div`
display: none;
${({ theme }) => theme.breakpoints.up('sm')} {
display: block;
}
`;
@media (min-width: 768px) {
display: block;
}
We occasionally use media queries that go in the other direction (the given screen size or smaller):
declare function down(
max: string,
orientation?: 'portrait' | 'landscape'
) => string
const Box = styled.div`
display: block;
${({ theme }) => theme.breakpoints.down('md')} {
display: none;
}
`;
@media (max-width: 767.98px) {
display: none;
}
Why subtract .02px? Browsers donโt currently support range context queries, so we work around the limitations of min- and max- prefixes and viewports with fractional widths (which can occur under certain conditions on high-dpi devices, for instance) by using values with higher precision.
There are also media queries and mixins for targeting a single segment of screen sizes using the minimum and maximum breakpoint widths.
declare function only(
name: string,
orientation?: 'portrait' | 'landscape'
) => string
const Box = styled.div`
background-color: pink;
${({ theme }) => theme.breakpoints.only('md')} {
background-color: rebeccapurple;
}
`;
@media (min-width: 768px) and (max-width: 991.98px) {
background-color: rebeccapurple;
}
Similarly, media queries may span multiple breakpoint widths.
declare function between(
min: string,
max: string,
orientation?: 'portrait' | 'landscape'
) => string
const Box = styled.div`
background-color: gold;
${({ theme }) => theme.breakpoints.between('md', 'xl')} {
background-color: rebeccapurple;
}
`;
@media (min-width: 768px) and (max-width: 1199.98px) {
background-color: rebeccapurple;
}
features:
declare function useMediaQuery(query?: string) => boolean
import { useTheme } from 'styled-components'; // or from '@emotion/react'
import { useMediaQuery } from 'styled-breakpoints/use-media-query';
import { Box } from 'third-party-library';
const SomeComponent = () => {
const isMd = useMediaQuery(useTheme()?.breakpoints.only('md'));
return <AnotherComponent>{isMd && <Box />}</AnotherComponent>;
};
app.tsx
import styled from 'styled-components'; // or from '@emotion/react'
import { createStyledBreakpointsTheme, MediaQueries } from 'styled-breakpoints';
const breakpoints = {
small: '0px',
medium: '640px',
large: '1024px',
xLarge: '1200px',
xxLarge: '1440px',
} as const;
type Min = keyof typeof breakpoints;
// For max values remove the first key.
type Max = Exclude<keyof typeof breakpoints, 'small'>;
export interface StyledBreakpointsTheme {
breakpoints: MediaQueries<Min, Max>;
}
const theme = createStyledBreakpointsTheme({
breakpoints,
}) as StyledBreakpointsTheme;
const App = () => (
<ThemeProvider theme={theme}>
<Box />
</ThemeProvider>
);
styled.d.ts
import 'styled-components';
import { StyledBreakpointsTheme } from './app';
declare module 'styled-components' {
export interface DefaultTheme extends StyledBreakpointsTheme {}
}
emotion.d.ts
import '@emotion/react';
import { StyledBreakpointsTheme } from './app';
declare module '@emotion/react' {
export interface Theme extends StyledBreakpointsTheme {}
}
app.tsx
import { ThemeProvider } from 'styled-components'; // or from '@emotion/react';
import { createStyledBreakpointsTheme, StyledBreakpointsTheme } from 'styled-breakpoints';
export const primaryTheme = {
fonts: ['sans-serif', 'Roboto'],
fontSizes: {
small: '1em',
medium: '2em',
large: '3em',
},
} as const;
const const theme = {
...primaryTheme,
...createStyledBreakpointsTheme() as StyledBreakpointsTheme,
}
const App = () => (
<ThemeProvider theme={theme}>
<Box />
</ThemeProvider>
);
styled.d.ts
import 'styled-components';
import { StyledBreakpointsTheme } from 'styled-breakpoints';
import { primaryTheme } from './app';
type PrimaryTheme = typeof primaryTheme;
declare module 'styled-components' {
export interface DefaultTheme extends StyledBreakpointsTheme, PrimaryTheme {}
}
emotion.d.ts
import '@emotion/react';
import { StyledBreakpointsTheme } from 'styled-breakpoints';
import { primaryTheme } from './app';
type PrimaryTheme = typeof primaryTheme;
declare module '@emotion/react' {
export interface Theme extends PrimaryTheme, StyledBreakpointsTheme {}
}
MIT License
Copyright (c) 2018-2019 Maxim Alyoshin.
This project is licensed under the MIT License - see the LICENSE file for details.
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Simple and powerful css breakpoints for styled-components and emotion
The npm package styled-breakpoints receives a total of 7,821 weekly downloads. As such, styled-breakpoints popularity was classified as popular.
We found that styled-breakpoints demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVDโs backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.