subcommand
Create CLI tools with subcommands. A minimalist CLI router based on minimist and cliclopts.

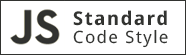

basic usage
first, define your CLI API in JSON like this:
var commands = [
{
name: 'foo',
options: [
{
name: 'loud',
boolean: true,
default: false,
abbr: 'v'
}
],
command: function foo (args) {
}
},
{
name: 'bar',
command: function bar (args) {
}
}
]
then pass them into subcommand
:
var subcommand = require('subcommand')
var match = subcommand(config, opts)
subcommand
returns a function (called match
above) that you can use to match/route arguments to their subcommands
the return value will be true
if a subcommand was matched, or false
if no subcommand was matched
var matched = match(['foo'])
var matched = match(['foo', 'baz', 'taco'])
var matched = match(['bar'])
var matched = match(['uhoh'])
advanced usage
instead of an array, you can also pass an object that looks like this as the first argument to subcommand
:
{
root: // root command options and handler
defaults: // default options
all: // function that gets called always, regardless of match or no match
none: // function that gets called only when there is no matched subcommand
commands: // the commands array from basic usage
}
see test.js
for a concrete example
root
to pass options to the 'root' command (e.g. when no subcommand is passed in), set up your config like this:
var config = {
root: {
options: [
{
name: 'loud',
boolean: true,
default: false,
abbr: 'v'
}
],
command: function (args) {
}
},
commands: yourSubCommandsArray
}
defaults
you can pass in a defaults options array, and all subcommands as well as the root command will inherit the default options
var config = {
defaults: [
{name: 'path', default: process.cwd()}
],
commands: yourSubCommandsArray
}
all
pass a function under the all
key and it will get called with the parsed arguments 100% of the time
var config = {
all: function all (args) { },
commands: yourSubCommandsArray
}
none
pass a function under the none
key and it will get called when no subcommand is matched
var config = {
none: function none (args) { },
commands: yourSubCommandsArray
}