What is svelte?
Svelte is a modern JavaScript compiler that allows you to write high-performance user interfaces with significantly less boilerplate code than traditional frameworks. It shifts much of the work to compile time, producing highly optimized vanilla JavaScript at the end.
What are svelte's main functionalities?
Reactive Declarations
Svelte allows you to write reactive statements using the $: syntax. When the state changes, the reactive statements automatically update to reflect the new state.
let count = 0;
$: doubled = count * 2;
Component Definition
Components in Svelte are defined using a combination of HTML, CSS, and JavaScript, which are encapsulated within a single file with a .svelte extension.
<script>
export let name;
</script>
<h1>Hello {name}!</h1>
Store Management
Svelte provides a simple store mechanism to manage global state. The 'writable' store is a basic store that allows reading and writing values reactively.
import { writable } from 'svelte/store';
const count = writable(0);
Transitions and Animations
Svelte makes it easy to add transitions and animations to elements when they enter or leave the DOM.
import { fade } from 'svelte/transition';
<div in:fade={{ delay: 0, duration: 200 }}>Fade In</div>
Bindings
Svelte provides a concise syntax for two-way data binding to HTML elements, allowing for easy synchronization between the DOM and component state.
<script>
let value = '';
</script>
<input bind:value={value} />
Other packages similar to svelte
react
React is a popular JavaScript library for building user interfaces. It uses a virtual DOM for efficient updates, and it's known for its component-based architecture. Unlike Svelte, React requires a runtime library and often involves more boilerplate code.
vue
Vue is a progressive JavaScript framework used for building UIs and single-page applications. It is similar to Svelte in its component structure and reactivity model but differs in that it uses a virtual DOM and requires a runtime.
angular
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. It is more prescriptive than Svelte, with a complex ecosystem and a steep learning curve, and it includes features like dependency injection and RxJS integration.
preact
Preact is a fast, 3kB alternative to React with the same modern API. It provides a similar component-based architecture but with a smaller footprint. Preact is closer to Svelte in terms of size but still operates with a virtual DOM.
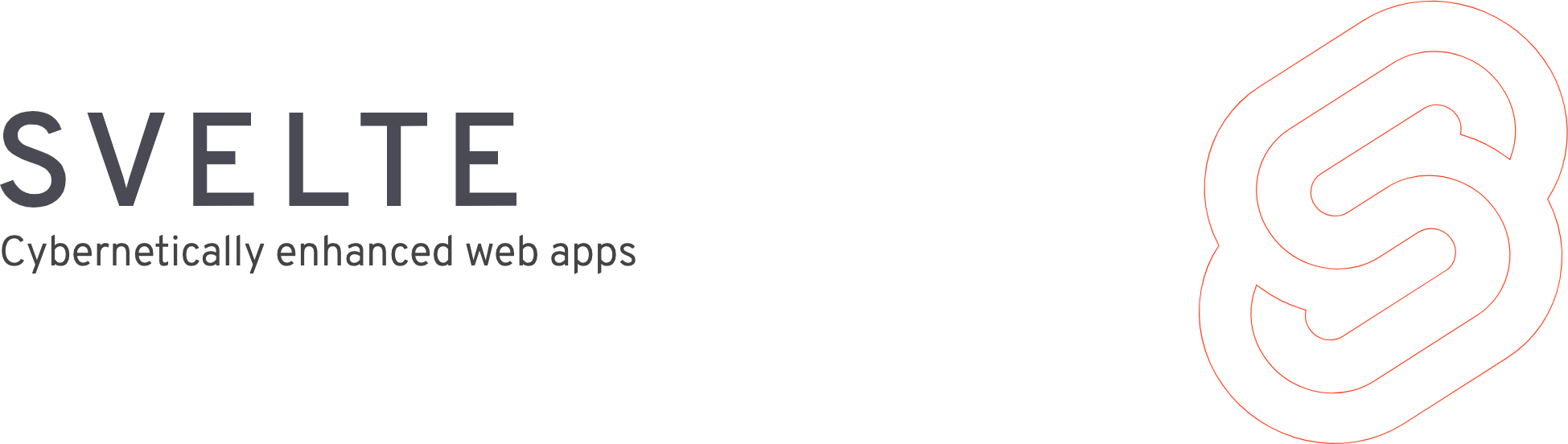

What is Svelte?
Svelte is a new way to build web applications. It's a compiler that takes your declarative components and converts them into efficient JavaScript that surgically updates the DOM.
Learn more at the Svelte website, or stop by the Discord chatroom.
Getting started
You can play around with Svelte in the tutorial, examples, and REPL.
When you're ready to build a full-fledge application, we recommend using SvelteKit:
npx sv create my-app
cd my-app
npm install
npm run dev
See the SvelteKit documentation to learn more.
Changelog
The Changelog for this package is available on GitHub.
Supporting Svelte
Svelte is an MIT-licensed open source project with its ongoing development made possible entirely by fantastic volunteers. If you'd like to support their efforts, please consider:
Funds donated via Open Collective will be used for compensating expenses related to Svelte's development.