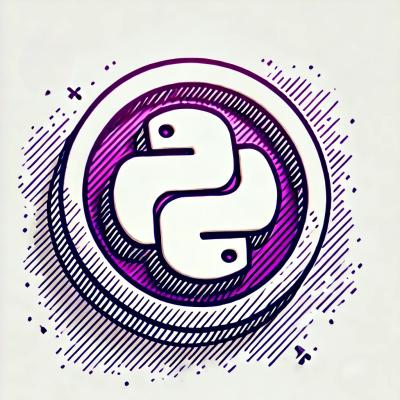
Security News
Python Overtakes JavaScript as Top Programming Language on GitHub
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
swagger-client
Advanced tools
The swagger-client npm package is a JavaScript client for Swagger/OpenAPI specifications. It allows you to interact with APIs described by Swagger/OpenAPI documents, making it easier to consume and interact with RESTful services.
Initialize Client
This feature allows you to initialize a Swagger client by providing the URL to the Swagger/OpenAPI specification. The client can then be used to interact with the API described by the specification.
const Swagger = require('swagger-client');
const client = new Swagger({ url: 'http://petstore.swagger.io/v2/swagger.json' });
Make API Calls
This feature allows you to make API calls using the initialized client. In this example, the client is used to call the 'getPetById' operation from the Petstore API.
client.then(client => {
client.apis.pet.getPetById({ petId: 1 }).then(response => {
console.log(response);
});
});
Handle Authentication
This feature allows you to handle authentication by providing authorization details when initializing the client. In this example, an API key is provided for authentication.
const client = new Swagger({
url: 'http://petstore.swagger.io/v2/swagger.json',
authorizations: {
api_key: new Swagger.ApiKeyAuthorization('api_key', 'YOUR_API_KEY', 'query')
}
});
Axios is a promise-based HTTP client for the browser and Node.js. While it does not specifically target Swagger/OpenAPI specifications, it can be used to make HTTP requests to any API. Compared to swagger-client, axios is more general-purpose and does not provide built-in support for Swagger/OpenAPI documents.
openapi-client-axios is a package that combines the capabilities of Axios with OpenAPI specifications. It allows you to generate an Axios client from an OpenAPI document, similar to swagger-client. However, it leverages Axios for making HTTP requests, providing a more flexible and widely-used HTTP client.
swagger-js is another JavaScript client for Swagger/OpenAPI specifications. It provides similar functionality to swagger-client, allowing you to interact with APIs described by Swagger/OpenAPI documents. The main difference is in the implementation and API design, but both packages serve the same purpose.
This is the Swagger javascript client for use with swagger enabled APIs. It's written in javascript and tested with mocha, and is the fastest way to enable a javascript client to communicate with a swagger-enabled server.
The goal of Swagger™ is to define a standard, language-agnostic interface to REST APIs which allows both humans and computers to discover and understand the capabilities of the service without access to source code, documentation, or through network traffic inspection. When properly defined via Swagger, a consumer can understand and interact with the remote service with a minimal amount of implementation logic. Similar to what interfaces have done for lower-level programming, Swager removes the guesswork in calling the service.
Check out Swagger-Spec for additional information about the Swagger project, including additional libraries with support for other languages and more.
Install swagger-client:
npm install swagger-client
Then let swagger do the work!
var client = require("swagger-client")
var swagger = new client.SwaggerClient({
url: 'http://petstore.swagger.wordnik.com/v2/swagger.json',
success: function() {
swagger.apis.pet.getPetById({petId:1});
}
});
That's it! You'll get a JSON response with the default callback handler:
{
"id": 1,
"category": {
"id": 2,
"name": "Cats"
},
"name": "Cat 1",
"photoUrls": [
"url1",
"url2"
],
"tags": [
{
"id": 1,
"name": "tag1"
},
{
"id": 2,
"name": "tag2"
}
],
"status": "available"
}
Need to pass an API key? Configure one as a querystring:
client.authorizations.add("apiKey", new client.ApiKeyAuthorization("api_key","special-key","query"));
...or with a header:
client.authorizations.add("apiKey", new client.ApiKeyAuthorization("api_key","special-key","header"));
Download swagger-client.js
and shred.bundle.js
into your lib folder
<script src='lib/shred.bundle.js' type='text/javascript'></script>
<script src='lib/swagger-client.js' type='text/javascript'></script>
<script type="text/javascript">
// initialize swagger, point to a resource listing
window.swagger = new SwaggerClient({
url: "http://petstore.swagger.wordnik.com/api/api-docs",
success: function() {
// upon connect, fetch a pet and set contents to element "mydata"
swagger.apis.pet.getPetById({petId:1}, function(data) {
document.getElementById("mydata").innerHTML = JSON.stringify(data.obj);
});
}
});
</script>
var pet = {
id: 100,
name: "dog"};
swagger.apis.pet.addPet({body: pet});
var pet = "<Pet><id>2</id><name>monster</name></Pet>";
swagger.apis.pet.addPet({body: pet}, {requestContentType:"application/xml"});
swagger.apis.pet.getPetById({petId:1}, {responseContentType:"application/xml"});
You can easily write your own request signing code for Swagger. For example:
var CustomRequestSigner = function(name) {
this.name = name;
};
CustomRequestSigner.prototype.apply = function(obj, authorizations) {
var hashFunction = this._btoa;
var hash = hashFunction(obj.url);
obj.headers["signature"] = hash;
return true;
};
In the above simple example, we're creating a new request signer that simply
base 64 encodes the URL. Of course you'd do something more sophisticated, but
after encoding it, a header called signature
is set before sending the request.
The swagger javascript client reads the swagger api definition directly from the server. As it does, it constructs a client based on the api definition, which means it is completely dynamic. It even reads the api text descriptions (which are intended for humans!) and provides help if you need it:
s.apis.pet.getPetById.help()
'* petId (required) - ID of pet that needs to be fetched'
The HTTP requests themselves are handled by the excellent shred library, which has a ton of features itself. But it runs on both node and the browser.
Please fork the code and help us improve swagger-client.js. Send us a pull request and we'll mail you a wordnik T-shirt!
swagger-js use gulp for Node.js.
# Install the gulp client on the path
npm install -g gulp
# Install all project dependencies
npm install
# List all tasks.
gulp -T
# Run the test suite
gulp test
# Build the library (minified and unminified) in the dist folder
gulp build
# continuously run the test suite:
gulp watch
# run jshint report
gulp lint
# run a coverage report
gulp cover
Copyright 2011-2015 Reverb Technologies, Inc.
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
FAQs
SwaggerJS - a collection of interfaces for OAI specs
The npm package swagger-client receives a total of 445,253 weekly downloads. As such, swagger-client popularity was classified as popular.
We found that swagger-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
Security News
Dutch National Police and FBI dismantle Redline and Meta infostealer malware-as-a-service operations in Operation Magnus, seizing servers and source code.
Research
Security News
Socket is tracking a new trend where malicious actors are now exploiting the popularity of LLM research to spread malware through seemingly useful open source packages.