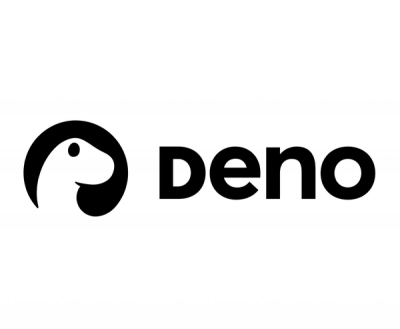
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
sweetalert2
Advanced tools
A beautiful, responsive, customizable and accessible (WAI-ARIA) replacement for JavaScript's popup boxes, supported fork of sweetalert
SweetAlert2 is a beautiful, responsive, customizable, and accessible (WAI-ARIA) replacement for JavaScript's popup boxes. It provides a wide range of alert types, including success, error, warning, and information alerts, as well as more complex modal dialogs.
Basic Alert
Displays a basic alert with a message.
Swal.fire('Hello world!');
Success Alert
Displays a success alert with a title, message, and success icon.
Swal.fire('Good job!', 'You clicked the button!', 'success');
Error Alert
Displays an error alert with a title, message, and error icon.
Swal.fire({ icon: 'error', title: 'Oops...', text: 'Something went wrong!' });
Custom HTML Alert
Displays an alert with custom HTML content, including bold text, links, and custom buttons.
Swal.fire({ title: '<strong>HTML <u>example</u></strong>', icon: 'info', html: 'You can use <b>bold text</b>, <a href="//sweetalert2.github.io">links</a> and other HTML tags', showCloseButton: true, showCancelButton: true, focusConfirm: false, confirmButtonText: '<i class="fa fa-thumbs-up"></i> Great!', confirmButtonAriaLabel: 'Thumbs up, great!', cancelButtonText: '<i class="fa fa-thumbs-down"></i>', cancelButtonAriaLabel: 'Thumbs down' });
Input Alert
Displays an alert with an input field for the user to submit data, and performs an asynchronous operation based on the input.
Swal.fire({ title: 'Submit your Github username', input: 'text', inputAttributes: { autocapitalize: 'off' }, showCancelButton: true, confirmButtonText: 'Look up', showLoaderOnConfirm: true, preConfirm: (login) => { return fetch(`//api.github.com/users/${login}`) .then(response => { if (!response.ok) { throw new Error(response.statusText) } return response.json() }) .catch(error => { Swal.showValidationMessage(`Request failed: ${error}`) }) }, allowOutsideClick: () => !Swal.isLoading() }).then((result) => { if (result.isConfirmed) { Swal.fire({ title: `${result.value.login}'s avatar`, imageUrl: result.value.avatar_url }) } });
Noty is a dependency-free notification library that makes it easy to create alert, success, error, warning, and information messages as an alternative to SweetAlert2. Noty is more focused on notifications rather than modal dialogs and offers a different set of customization options.
Toastr is a simple JavaScript toast notification library that is similar to SweetAlert2 but focuses on non-blocking notifications. It is lightweight and easy to use, making it a good choice for simple alert needs without the need for modal dialogs.
Bootbox.js is a small JavaScript library that allows you to create programmatic dialog boxes using Bootstrap modals. It provides similar functionalities to SweetAlert2 but is tightly integrated with Bootstrap, making it a good choice if you are already using Bootstrap in your project.
A beautiful, responsive, customizable, accessible (WAI-ARIA) replacement for JavaScript's popup boxes. Zero dependencies.
:shipit: The author of SweetAlert2 (@limonte) is looking for short-term to medium-term working contracts in front-end, preferably OSS.
:point_right: Upgrading from v8.x to v9.x? Read the release notes!
If you're upgrading from v7.x, please upgrade from v7 to v8 first!
If you're upgrading from v6.x, please upgrade from v6 to v7 first!
:point_right: Migrating from SweetAlert? SweetAlert 1.x to SweetAlert2 migration guide
npm install --save sweetalert2
Or grab from jsdelivr CDN :
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@9"></script>
<script src="sweetalert2/dist/sweetalert2.all.min.js"></script>
<!-- Include a polyfill for ES6 Promises (optional) for IE11 -->
<script src="https://cdn.jsdelivr.net/npm/promise-polyfill9/dist/polyfill.js"></script>
You can also include the stylesheet separately if desired:
<script src="sweetalert2/dist/sweetalert2.min.js"></script>
<link rel="stylesheet" href="sweetalert2/dist/sweetalert2.min.css">
Or:
// ES6 Modules or TypeScript
import Swal from 'sweetalert2'
// CommonJS
const Swal = require('sweetalert2')
Or with JS modules:
<link rel="stylesheet" href="sweetalert2/dist/sweetalert2.css">
<script type="module">
import Swal from 'sweetalert2/src/sweetalert2.js'
</script>
It's possible to import JS and CSS separately, e.g. if you need to customize styles:
import Swal from 'sweetalert2/dist/sweetalert2.js'
import 'sweetalert2/src/sweetalert2.scss'
Please note that TypeScript is well-supported, so you don't have to install a third-party declaration file.
The most basic message:
Swal.fire('Hello world!')
A message signaling an error:
Swal.fire('Oops...', 'Something went wrong!', 'error')
Handling the result of SweetAlert2 modal:
Swal.fire({
title: 'Are you sure?',
text: 'You will not be able to recover this imaginary file!',
icon: 'warning',
showCancelButton: true,
confirmButtonText: 'Yes, delete it!',
cancelButtonText: 'No, keep it'
}).then((result) => {
if (result.value) {
Swal.fire(
'Deleted!',
'Your imaginary file has been deleted.',
'success'
)
// For more information about handling dismissals please visit
// https://sweetalert2.github.io/#handling-dismissals
} else if (result.dismiss === Swal.DismissReason.cancel) {
Swal.fire(
'Cancelled',
'Your imaginary file is safe :)',
'error'
)
}
})
IE11* | Edge | Chrome | Firefox | Safari | Opera | UC Browser |
---|---|---|---|---|---|---|
:heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: |
* ES6 Promise polyfill should be included, see usage example.
Note that SweetAlert2 does not and will not provide support or functionality of any kind on IE10 and lower.
sweetalert2-themes ↗
)@gverni | @zenflow | @toverux |
If you would like to contribute enhancements or fixes, please do the following:
Fork the sweetalert2
repository and clone it locally.
When in the SweetAlert2 directory, run npm install
or yarn install
to install dependencies.
To begin active development, run npm start
or yarn start
. This does several things for you:
dist
folder![]() | ![]() | ![]() | ![]() | |
---|---|---|---|---|
Become a sponsor | FlowCrypt | WP Reset | SebaEBC | Lapakle |
![]() | ![]() | ![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|---|---|
SexualAlpha | STED | YourDoll | STC | Bingato | RealSexDoll | DoctorClimax |
Has SweetAlert2 helped you create an amazing application? You can show your support by making a donation:
16Z7RvFv7PsV3XzFvchYwPnRfw9KeLTZQJ
0x192096161eB2273f12b1cB4E31aBB09Bfc03a7F3
qz28x66hrljtdz3052p8ya3cmkwwva5avy0msz2ej3
GDUM4VJZYDNRHBTKUQBOPC374AP6MMMVOJDMSHIPEJPEMBCY4ZHH6NDY
FAQs
A beautiful, responsive, customizable and accessible (WAI-ARIA) replacement for JavaScript's popup boxes, supported fork of sweetalert
The npm package sweetalert2 receives a total of 435,853 weekly downloads. As such, sweetalert2 popularity was classified as popular.
We found that sweetalert2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.