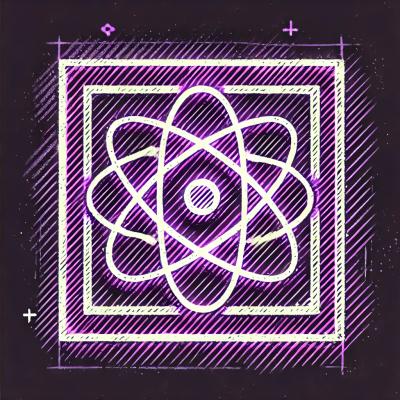
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
A collection of useful little things that I like to reuse across projects
A collection of utils for calculating simple times.
Each unit (e.g. second) has: a type (second
), a constant (SECOND
) and a function for getting multiples (seconds(x: second) => ms
)
unit | type | constant | function |
---|---|---|---|
millisecond | ms | MILLISECOND | milliseconds(x: ms) => ms |
second | second | SECOND | seconds(x: second) => ms |
minute | minute | MINUTE | minutes(x: minute) => ms |
hour | hour | HOUR | hours(x: hour) => ms |
day | day | DAY | days(x: day) => ms |
week | week | WEEK | weeks(x: week) => ms |
month | month | MONTH | months(x: month) => ms |
year | year | YEAR | years(x: year) => ms |
decade | decade | DECADE | decades(x: decade) => ms |
century | century | CENTURY | centuries(x: century) => ms |
millennium | millennium | MILLENNIUM | millenniums(x: millennium) => ms |
Examples
import { MINUTE, hours, minutes } from 'swiss-ak';
const start = Date.now();
// wait for 2 hours and 12 minutes (132 minutes)
setTimeout(() => {
const duration = Date.now() - start;
console.log(`It's been ${duration / MINUTE} mins`); // Result: It's been 132 minutes
}, hours(2) + minutes(12));
Async functions that return promises at or after a given time.
'Accurate/pinged' waiters ping at intermediary points to resolve at a more accurate time.
Name | Description | Example |
---|---|---|
wait | Standard wait promise (using setTimeout) | minutes(2) = in 2 minutes |
waitFor | Accurate (pinged) wait the given ms | minutes(2) = in 2 minutes |
waitUntil | Accurate (pinged) wait until given time | Date.now() + minutes(2) = in 2 minutes |
waitEvery | Accurate (pinged) wait for next 'every X' event | hours(1) = next full hour (e.g. 17:00, 22:00) |
interval | Accurate (pinged) interval for every 'every X' event | hours(1) = every hour, on the hour |
import { wait } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await wait(minutes(2));
console.log(new Date().toTimeString()); // 12:32:10
import { waitFor } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await waitFor(minutes(5));
console.log(new Date().toTimeString()); // 12:35:10
import { waitUntil } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await waitUntil(Date.now() + minutes(10));
console.log(new Date().toTimeString()); // 12:40:10
import { waitEvery } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await waitEvery(hours(2));
console.log(new Date().toTimeString()); // 14:00:00
import { interval, stopInterval } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
interval((intID, count) => {
console.log(new Date().toTimeString()); // 13:00:00, 14:00:00, 15:00:00
if (count === 3) {
stopInterval(intID);
}
}, hours(1));
No operation. Do nothing, return nothing.
const run = condition ? doSomething : fn.noop;
run();
No action. Returns the first argument it receives.
const items = stuff.map(condition ? mapSomething : fn.noact);
Returns a function that returns a function that returns the first argument.
const items = stuff.filter(condition ? mapSomething : fn.result(true));
Returns an async function that resolves to the first argument
Like fn.result, but wrapped in a Promise
Returns an async function that rejects with the first argument
Fixes floating point errors that may occur when adding/subtracting/multiplying/dividing real/float numbers
0.1 + 0.2; // 0.30000000000000004
fixFloat(0.1 + 0.2); // 0.3
Adds all numbers together. Each argument is a number (use spread operator to pass in an array) similar to Math.min/Math.max
addAll(1, 2, 3, 4, 5); // 15
Returns true if item isn't null or undefined.
[null, 1, undefined, 2].filter(fn.exists); // [1, 2]
Returns true if item is truthy.
[0, 1, 2].filter(fn.isTruthy); // [1, 2]
['', 'a', 'b'].filter(fn.isTruthy); // ['a', 'b']
Returns true if item is falsy.
[0, 1, 2].filter(fn.isFalsy); // [0]
['', 'a', 'b'].filter(fn.isFalsy); // ['']
Returns true if item's length is 0
['', 'a', 'b'].filter(fn.isEmpty); // ['']
[[], [1], [2]].filter(fn.isEmpty); // [[]]
Returns true if item's length is 1 or more
['', 'a', 'b'].filter(fn.isNotEmpty); // ['a', 'b']
[[], [1], [2]].filter(fn.isNotEmpty); // [[1], [2]]
Returns a function that returns true if the item is equal to provided value.
[0, 1, 2].filter(fn.isEqual(1)); // [1]
Returns a function that returns true if the item is not equal to provided value.
[0, 1, 2].filter(fn.isNotEqual(1)); // [0, 2]
Maps the item to a string.
[0, 1, 2].map(fn.toString); // ['0', '1', '2']
Maps the item to a number.
['0', '1', '2'].map(fn.toNumber); // [0, 1, 2]
Maps the item to a boolean.
[0, 1, 2].map(fn.toBool); // [false, true, true]
['true', 'false', '', 'text'].map(fn.toBool); // [true, false, false, true]
Maps the item to a given property of the item
[{ name: 'Jack' }, { name: 'Jill' }].map(fn.toProp('name')); // ['Jack', 'Jill']
Sort ascending.
[2, 4, 3, 1].sort(fn.asc); // [1, 2, 3, 4]
Sort descending.
[2, 4, 3, 1].sort(fn.asc); // [4, 3, 2, 1]
Sort by a given property.
const people = [{ age: 2 }, { age: 4 }, { age: 3 }, { age: 1 }];
people.sort(fn.byProp('age', fn.asc)); // [{age: 1}, {age: 2}, {age: 3}, {age: 4}]
Sort by the nearest value to the given value.
const people = [2, 4, 3, 1];
people.sort(fn.nearestTo(3)); // [3, 2, 4, 1]
Sort by the furthest value to the given value.
const people = [2, 4, 3, 1];
people.sort(fn.furthestFrom(3)); // [1, 2, 4, 3]
Sort an array of arrays in ascending order
Sort an array of arrays in descending order
Adds or concats the items
[1, 2, 3].reduce(fn.combine); // 6
['a', 'b', 'c'].reduce(fn.combine); // 'abc'
Adds or concats the given property of the items
const people = [
{ name: 'a', age: 1 },
{ name: 'b', age: 2 },
{ name: 'c', age: 3 }
];
people.reduce(fn.combineProp('age')); // 6
people.reduce(fn.combineProp('name')); // 'abc'
Returns if all the items are equal to one another.
[1, 1, 1].every(fn.isAllEqual); // true
[1, 2, 1].every(fn.isAllEqual); // false
Floors a number down to the nearest multiple of the given number.
fn.round.floorTo(10, 102); // 100
fn.round.floorTo(5, 53); // 50
fn.round.floorTo(0.1, 0.25); // 0.2
Floors a number down to the nearest multiple of the given number.
fn.round.to(10, 102); // 100
fn.round.to(5, 53); // 55
fn.round.to(0.1, 0.25); // 0.3
Floors a number down to the nearest multiple of the given number.
fn.round.ceilTo(10, 102); // 110
fn.round.ceilTo(5, 53); // 55
fn.round.ceilTo(0.1, 0.25); // 0.3
Try to execute a function and return its result if it succeeds, or return the default value if it fails.
const result = tryOr('default', () => getSomething());
Try to execute a function and return its result if it succeeds, or retry a given number of times until it succeeds.
const result = tryOr(5, seconds(1),, true, () => getSomething());
Combination of retry and tryOr.
Try to execute a function and return its result if it succeeds, or retry a given number of times until it succeeds. Return the default value if it fails too many times
const result = retryOr('default', 5, seconds(1), true, () => getSomething());
A handy little tool for tracking how long things are taking
const timer = getTimer('Example', false, chalk.red, chalk, {
TOTAL: 'TOTAL',
INTRO: 'Action 1',
ENDING: 'Action 2'
});
timer.start(timer.TOTAL, timer.INTRO);
await wait(seconds(4)); // do something async
timer.switch(timer.INTRO, timer.ENDING); // same as calling end(timer.INTRO) and start(timer.ENDING)
await wait(seconds(6)); // do something async
timer.end(timer.TOTAL, timer.ENDING);
timer.log();
Output:
Example Times:
TOTAL: 10s
Action 1: 4s
Action 2: 6s
There is also a global instance for smaller projects/scripts
import { timer } from 'swiss-ak';
timer.start(timer.TOTAL);
Returns an array of the given length, where each value is equal to it's index e.g. [0, 1, 2, ..., length]
range(3); // [0, 1, 2]
range(5); // [0, 1, 2, 3, 4]
range(10); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
range(3, 2); // [0, 2, 4]
range(5, 2); // [0, 2, 4, 6, 8]
range(10, 10); // [0, 10, 20, 30, 40, 50, 60, 70, 80, 90]
Converts multiple arrays into an array of 'tuples' for each value at the corresponding indexes.
Limited to the length of the shortest provided array
Inspired by python's 'zip'
zip([1, 2, 3, 4], ['a', 'b', 'c']); // [ [1, 'a'], [2, 'b'], [3, 'c'] ]
Sort an array by a mapped form of the values, but returning the initial values
sortByMapped(['2p', '3p', '1p'], (v) => Number(v.replace('p', ''))); // ['1p', '2p', '3p']
sortByMapped(
['2p', '3p', '1p'],
(v) => Number(v.replace('p', '')),
(a, b) => b - a
); // ['3p', '2p', '1p']
Returns a clone of the provided array with it's items in a random order
randomise([1, 2, 3, 4, 5, 6]); // [ 5, 3, 4, 1, 2, 6 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 5, 1, 3, 2, 4, 6 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 6, 1, 4, 5, 2, 3 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 1, 4, 5, 2, 3, 6 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 2, 6, 1, 3, 4, 5 ]
Returns a new array with the order reversed without affecting original array
const arr1 = [1, 2, 3];
arr1; // [1, 2, 3]
arr1.reverse(); // [3, 2, 1]
arr1; // [3, 2, 1]
const arr2 = [1, 2, 3];
arr2; // [1, 2, 3]
reverse(arr2); // [3, 2, 1]
arr2; // [1, 2, 3]
Returns array of 'tuples' of index/value pairs
const arr = ['a', 'b', 'c'];
entries(arr); // [ [0, 'a'], [1, 'b'], [2, 'c'] ]
for (let [index, value] of entries(arr)) {
console.log(index); // 0, 1, 2
console.log(value); // 'a', 'b', 'c'
}
Returns an array with the given items repeated
repeat(5, 'a'); // [ 'a', 'a', 'a', 'a', 'a' ]
repeat(5, 'a', 'b'); // [ 'a', 'b', 'a', 'b', 'a' ]
'Roll' the array by a given amount so that is has a new first item. Length and contents remain the same, but the order is changed
roll(1, [0, 1, 2, 3, 4, 5, 6, 7]); // [ 1, 2, 3, 4, 5, 6, 7, 0 ]
roll(4, [0, 1, 2, 3, 4, 5, 6, 7]); // [ 4, 5, 6, 7, 0, 1, 2, 3 ]
Alphabetically sorts a list of strings, but keeps multi-digit numbers in numerical order (rather than alphabetical)
const names = ['name1', 'name10', 'name2', 'foo20', 'foo10', 'foo9'];
names.sort(); // [ 'foo10', 'foo20', 'foo9', 'name1', 'name10', 'name2' ]
sortNumberedText(names); // [ 'foo9', 'foo10', 'foo20', 'name1', 'name2', 'name10' ]
Maps the keys and values of an object in a similar way to Array.map
ObjectUtils.map({ a: 1, b: 2, c: 3 }, (key, value) => [key, key + value]); // {a: 'a1', b: 'b2', c: 'c3'}
Maps the values of an object in a similar way to Array.map
ObjectUtils.map({ a: 1, b: 2, c: 3 }, (key, value) => key.repeat(value)); // {a: 'a', b: 'bb', c: 'ccc'}
Maps the values of an object in a similar way to Array.map
ObjectUtils.map({ a: 1, b: 2, c: 3 }, (key, value) => key.repeat(value)); // {a: 1, bb: 2, ccc: 3}
A good old-fashioned (not recommended) deferred promise.
import { getDeferred } from 'swiss-ak';
const run = () => {
const deferred = getDeferred<number>();
doSomethingWithACallback('a', 'b', (err: Error, result: number) => {
// callback (just an example - don't actually do this this way)
if (err) return deferred.reject(err);
deferred.resolve(result);
});
return deferred.promise;
};
const luckyNumber: number = await run();
An alias for Promise.all
Like Promise.all, but limits the numbers of concurrently running items.
Takes an array of functions (that return Promises), rather than an array of Promises
import { PromiseUtils, timer, ms, seconds } from 'swiss-ak';
const give = async (delay: ms, result: number, label: string) => {
await waitFor(delay);
timer.end(label);
return result;
};
timer.start('allLimit', 'a', 'b', 'c', 'd');
const results = PromiseUtils.allLimit<number>(2, [
give(seconds(5), 1, 'a'),
give(seconds(5), 2, 'b'),
give(seconds(5), 3, 'c'),
give(seconds(5), 4, 'd')
]);
timer.end('allLimit');
console.log(results); // [ 1, 2, 3, 4 ]
timer.log();
// Times:
// allLimit: 10s
// a: 5s
// b: 5s
// c: 10s
// d: 10s
Run an async function against each item in an array
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
await PromiseUtils.each<number>(arr, async (val: number) => {
await wait(seconds(2));
sendToSomewhere(val);
});
console.log(''); // after 2 seconds
Run an async function against each item in an array, limiting the number of items that can run concurrently.
See PromiseUtils.allLimit for information about limited functions.
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
await PromiseUtils.eachLimit<number>(2, arr, async (val: number) => {
await wait(seconds(2));
sendToSomewhere(val);
});
console.log(''); // after 4 seconds
Run an async map function against each item in an array, mapping the results to a returned array
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
const mapped = await PromiseUtils.map<number>(arr, async (val: number) => {
await wait(seconds(2));
return val * 2;
});
console.log(mapped); // [2, 4, 6, 8] (after 2 seconds)
Run an async map function against each item in an array, mapping the results to a returned array, and limiting the number of items that can run concurrently.
See PromiseUtils.allLimit for information about limited functions.
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
const mapped = await PromiseUtils.mapLimit<number>(2, arr, async (val: number) => {
await wait(seconds(2));
return val * 2;
});
console.log(mapped); // [2, 4, 6, 8] (after 4 seconds)
Like Promise.all, but takes/gives an object instead of an array
import { PromiseUtils, timer, ms, seconds } from 'swiss-ak';
const give = async (delay: ms, result: number, label: string) => {
await waitFor(delay);
timer.end(label);
return result;
};
timer.start('allObj', 'a', 'b', 'c');
const results = PromiseUtils.allObj<number>({
a: give(seconds(10), 1, 'a'),
b: give(seconds(15), 2, 'b'),
c: give(seconds(20), 3, 'c')
});
timer.end('allObj');
console.log(results); // { a: 1, b: 2, c: 3 }
timer.log();
// Times:
// allObj: 20s
// a: 10s
// b: 15s
// c: 20s
A mix of allObj and allLimit.
Takes an array of functions (that return Promises), and limits the numbers of concurrently running items.
import { PromiseUtils, timer, ms, seconds } from 'swiss-ak';
const give = async (delay: ms, result: number, label: string) => {
await waitFor(delay);
timer.end(label);
return result;
};
timer.start('allLimitObj', 'a', 'b', 'c', 'd');
const results = PromiseUtils.allLimitObj<number>(2, {
a: give(seconds(5), 1, 'a'),
b: give(seconds(5), 2, 'b'),
c: give(seconds(5), 3, 'c'),
d: give(seconds(5), 4, 'd')
});
timer.end('allLimitObj');
console.log(results); // { a: 1, b: 2, c: 3, d: 4 }
timer.log();
// Times:
// allLimitObj: 10s
// a: 5s
// b: 5s
// c: 10s
// d: 10s
A dictionary of different colour names and their RGB values
ColourUtils.namedColours.blue; // [0, 0, 255]
ColourUtils.namedColours.red; // [255, 0, 0]
ColourUtils.namedColours.green; // [0, 255, 0]
ColourUtils.namedColours.azure; // [240, 255, 255]
ColourUtils.namedColours.darkorange; // [255, 140, 0]
ColourUtils.namedColours.dodgerblue; // [30, 144, 255]
Parse a string into a colour object (RGB array) Not extensive. Currently limited to:
ColourUtils.parse('#FF0000'); // [255, 0, 0]
ColourUtils.parse('rgb(255, 0, 0)'); // [255, 0, 0]
ColourUtils.parse('red'); // [255, 0, 0]
Convert a colour object (RGB array) to a hex string
ColourUtils.toHex([255, 0, 0]); // '#FF0000'
Get the luminance value of a given colour.
Between 0 and 255. Calculated using the formula: (RED × 0.299) + (GREEN × 0.587) + (BLUE × 0.114)
getLuminance([255, 0, 0]); // 76.245
getLuminance([0, 255, 0]); // 149.685
getLuminance([0, 0, 255]); // 29.07
Get the opposite colour of a given colour.
invertColour([255, 0, 0]); // [0, 255, 255]
invertColour([0, 255, 0]); // [ 255, 0, 255 ]
invertColour([0, 0, 255]); // [ 255, 255, 0 ]
Get the colour that contrasts the most with a given colour. (White or black)
Returned colour can be used as a text colour on top of the provided colour
getContrastedColour([255, 0, 0]); // [255, 255, 255]
getContrastedColour([255, 255, 0]); // [0, 0, 0]
A util for creating a 'visual' progress bar for better representing progress of multiple operations.
All options are optional.
Property | Default | Description |
---|---|---|
prefix | '' | String to show to left of progress bar |
prefixWidth | 1 | Min width of prefix - 10 => Example˽˽˽ |
maxWidth | process.stdout.columns or 100 | The maximum width the entire string may extend |
chalk | nothing | the chalk module, if available |
wrapperFn | nothing | function to wrap the printed string (eg chalk.cyan) |
showPercent | false | Show percentage completed - ( 69%) |
showCount | true | Show numerical values of the count - [11 / 15] |
countWidth | 0 | Min width of nums for showCount - 3 => [˽˽1 / ˽15] |
progChar | '█' | Character to use for progress section of bar |
emptyChar | ' ' | Character to use for empty (rail) section of bar |
startChar | '▕' | Character to start the progress bar with |
endChar | '▏' | Character to end the progress bar with |
import chalk from 'chalk';
import { getProgressBar } from 'swiss-ak';
console.log('-'.repeat(20) + ' < 20 Chars');
const progress = getProgressBar(5, {
prefix: 'ABC',
maxWidth: 20,
chalk,
wrapperFn: chalk.green
});
for (let i = 1; i <= 5; i++) {
progress.set(i);
}
progress.finish();
Output:
-------------------- < 20 Chars
ABC ▕ ▏ [0 / 5]
ABC ▕█ ▏ [1 / 5]
ABC ▕██ ▏ [2 / 5]
ABC ▕████ ▏ [3 / 5]
ABC ▕█████ ▏ [4 / 5]
ABC ▕██████▏ [5 / 5]
Can use instead of console.log
Overwrites the previous line if possible (i.e. node);
import { printLn } from 'swiss-ak';
printLn('A');
printLn('B'); // Replaces the 'A' line
printLn('C'); // Replaces the 'B' line
printLn(); // Jumps a line
printLn('D'); // Replaces the empty line
Output
C
D
A series of characters that can be used for display symbols
Key | Symbol |
---|---|
symbols.TAB | |
symbols.TICK | ✔ |
symbols.CROSS | ✖ |
symbols.PLUS | + |
symbols.MINUS | - |
symbols.TIMES | × |
symbols.DIVIDE | ÷ |
symbols.ELLIPSIS | … |
symbols.BULLET | • |
symbols.EJECT | ⏏ |
symbols.TILDE | ~ |
symbols.HOME | ~ |
symbols.CHEV_LFT | ‹ |
symbols.CHEV_RGT | › |
symbols.TRI_UPP | ▲ |
symbols.TRI_DWN | ▼ |
symbols.TRI_RGT | ▶ |
symbols.TRI_LFT | ◀ |
symbols.ARROW_UPP | ↑ |
symbols.ARROW_DWN | ↓ |
symbols.ARROW_RGT | → |
symbols.ARROW_LFT | ← |
symbols.ARROW_UPP_RGT | ↗ |
symbols.ARROW_DWN_RGT | ↘ |
symbols.ARROW_DWN_LFT | ↙ |
symbols.ARROW_UPP_LFT | ↖ |
symbols.ARROW_STILL | • |
symbols.ARROW_FLIP_H | ↔ |
symbols.ARROW_FLIP_V | ↕ |
symbols.ARROW_ROTATE_UPP | ⤴ |
symbols.ARROW_ROTATE_DWN | ⤵ |
symbols.ARROW_ROTATE_LFT | ⤶ |
symbols.ARROW_ROTATE_RGT | ⤷ |
symbols.ARROW_ROTATE_CLOCK | ↻ |
symbols.ARROW_ROTATE_ANTI_CLOCK | ↺ |
symbols.FRACTION_1_4 | ¼ |
symbols.FRACTION_1_2 | ½ |
symbols.FRACTION_3_4 | ¾ |
symbols.SUPERSCRIPT['1'] | ¹ |
symbols.SUPERSCRIPT['2'] | ² |
symbols.SUPERSCRIPT['3'] | ³ |
symbols.SUPERSCRIPT['4'] | ⁴ |
symbols.SUPERSCRIPT['5'] | ⁵ |
symbols.SUPERSCRIPT['6'] | ⁶ |
symbols.SUPERSCRIPT['7'] | ⁷ |
symbols.SUPERSCRIPT['8'] | ⁸ |
symbols.SUPERSCRIPT['9'] | ⁹ |
symbols.SUPERSCRIPT['0'] | ⁰ |
symbols.SUPERSCRIPT['-'] | ⁻ |
symbols.SUPERSCRIPT['+'] | ⁺ |
symbols.SUPERSCRIPT['='] | ⁼ |
symbols.SUPERSCRIPT['('] | ⁽ |
symbols.SUPERSCRIPT[')'] | ⁾ |
symbols.SUPERSCRIPT['i'] | ⁱ |
symbols.SUPERSCRIPT['n'] | ⁿ |
symbols.SUPERSCRIPT['o'] | ° |
symbols.SUPERSCRIPT['*'] | ° |
Makes all properties in T optional.
interface ITest {
a: string;
b: boolean;
}
type PartialTest = Partial<ITest>; // { a?: string, b?: boolean }
Makes all the values equal to the keys of T
interface ITest {
a: string;
b: boolean;
}
type KeysOnlyTest = KeysOnly<ITest>; // { a: 'a', b: 'b' }
Makes all the values numbers
interface ITest {
a: string;
b: boolean;
}
type NumberedTest = Numbered<ITest>; // { a: number, b: number }
Makes all the properties of object T have type U
An object with any properties of type T
These are used in non-vital personal projects and scripts.
Need to be better tested before being used in prod.
Failing/erroring/rejected promises may not behave as expected.
FAQs
A collection of useful little things that I like to reuse across projects
The npm package swiss-ak receives a total of 0 weekly downloads. As such, swiss-ak popularity was classified as not popular.
We found that swiss-ak demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.