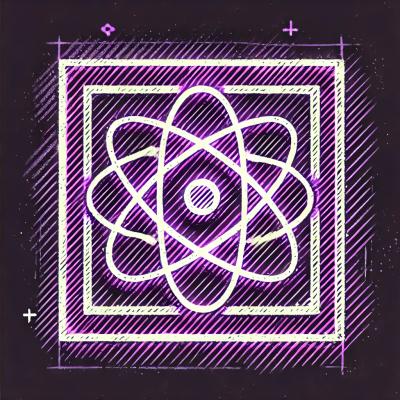
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
A collection of useful little things that I like to reuse across projects
A collection of utils for calculating simple times.
Each unit (e.g. second) has: a type (second
), a constant (SECOND
) and a function for getting multiples (seconds(x: second) => ms
)
unit | type | constant | function |
---|---|---|---|
millisecond | ms | MILLISECOND | milliseconds(x: ms) => ms |
second | second | SECOND | seconds(x: second) => ms |
minute | minute | MINUTE | minutes(x: minute) => ms |
hour | hour | HOUR | hours(x: hour) => ms |
day | day | DAY | days(x: day) => ms |
week | week | WEEK | weeks(x: week) => ms |
month | month | MONTH | months(x: month) => ms |
year | year | YEAR | years(x: year) => ms |
decade | decade | DECADE | decades(x: decade) => ms |
century | century | CENTURY | centuries(x: century) => ms |
millennium | millennium | MILLENNIUM | millenniums(x: millennium) => ms |
Examples
import { MINUTE, hours, minutes } from 'swiss-ak';
const start = Date.now();
// wait for 2 hours and 12 minutes (132 minutes)
setTimeout(() => {
const duration = Date.now() - start;
console.log(`It's been ${duration / MINUTE} mins`); // Result: It's been 132 minutes
}, hours(2) + minutes(12));
Async functions that return promises at or after a given time.
'Accurate/pinged' waiters ping at intermediary points to resolve at a more accurate time.
Name | Description | Example |
---|---|---|
wait | Standard wait promise (using setTimeout) | minutes(2) = in 2 minutes |
waitFor | Accurate (pinged) wait the given ms | minutes(2) = in 2 minutes |
waitUntil | Accurate (pinged) wait until given time | Date.now() + minutes(2) = in 2 minutes |
waitEvery | Accurate (pinged) wait for next 'every X' event | hours(1) = next full hour (e.g. 17:00, 22:00) |
interval | Accurate (pinged) interval for every 'every X' event | hours(1) = every hour, on the hour |
import { wait } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await wait(minutes(2));
console.log(new Date().toTimeString()); // 12:32:10
import { waitFor } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await waitFor(minutes(5));
console.log(new Date().toTimeString()); // 12:35:10
import { waitUntil } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await waitUntil(Date.now() + minutes(10));
console.log(new Date().toTimeString()); // 12:40:10
import { waitEvery } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
await waitEvery(hours(2));
console.log(new Date().toTimeString()); // 14:00:00
import { interval, stopInterval } from 'swiss-ak';
console.log(new Date().toTimeString()); // 12:30:10
interval((intID, count) => {
console.log(new Date().toTimeString()); // 13:00:00, 14:00:00, 15:00:00
if (count === 3) {
stopInterval(intID);
}
}, hours(1));
A handy little tool for tracking how long things are taking
const timer = getTimer('Example', false, chalk.red, chalk, {
TOTAL: 'TOTAL',
INTRO: 'Action 1',
ENDING: 'Action 2'
});
timer.start(timer.TOTAL, timer.INTRO);
await wait(seconds(4)); // do something async
timer.switch(timer.INTRO, timer.ENDING); // same as calling end(timer.INTRO) and start(timer.ENDING)
await wait(seconds(6)); // do something async
timer.end(timer.TOTAL, timer.ENDING);
timer.log();
Output:
Example Times:
TOTAL: 10s
Action 1: 4s
Action 2: 6s
There is also a global instance for smaller projects/scripts
import { timer } from 'swiss-ak';
timer.start(timer.TOTAL);
Returns an array of the given length, where each value is equal to it's index e.g. [0, 1, 2, ..., length]
range(3); // [0, 1, 2]
range(5); // [0, 1, 2, 3, 4]
range(10); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Converts multiple arrays into an array of 'tuples' for each value at the corresponding indexes.
Limited to the length of the shortest provided array
Inspired by python's 'zip'
Note: The typing of this is messy - needs improvement
zip([1, 2, 3, 4], ['a', 'b', 'c']); // [ [1, 'a'], [2, 'b'], [3, 'c'] ]
Sort an array by a mapped form of the values, but returning the initial values
sortByMapped(['2p', '3p', '1p'], (v) => Number(v.replace('p', ''))); // ['1p', '2p', '3p']
sortByMapped(
['2p', '3p', '1p'],
(v) => Number(v.replace('p', '')),
(a, b) => b - a
); // ['3p', '2p', '1p']
Returns a clone of the provided array with it's items in a random order
randomise([1, 2, 3, 4, 5, 6]); // [ 5, 3, 4, 1, 2, 6 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 5, 1, 3, 2, 4, 6 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 6, 1, 4, 5, 2, 3 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 1, 4, 5, 2, 3, 6 ]
randomise([1, 2, 3, 4, 5, 6]); // [ 2, 6, 1, 3, 4, 5 ]
Returns a new array with the order reversed without affecting original array
const arr1 = [1, 2, 3];
arr1; // [1, 2, 3]
arr1.reverse(); // [3, 2, 1]
arr1; // [3, 2, 1]
const arr2 = [1, 2, 3];
arr2; // [1, 2, 3]
reverse(arr2); // [3, 2, 1]
arr2; // [1, 2, 3]
Returns array of 'tuples' of index/value pairs
const arr = ['a', 'b', 'c'];
entries(arr); // [ [0, 'a'], [1, 'b'], [2, 'c'] ]
for (let [index, value] of entries(arr)) {
console.log(index); // 0, 1, 2
console.log(value); // 'a', 'b', 'c'
}
Returns an array with the given items repeated
repeat(5, 'a', 'b'); // 'a', 'b', 'a', 'b', 'a'
A good old-fashioned (not recommended) deferred promise.
import { getDeferred } from 'swiss-ak';
const run = () => {
const deferred = getDeferred<number>();
doSomethingWithACallback('a', 'b', (err: Error, result: number) => {
// callback (just an example - don't actually do this this way)
if (err) return deferred.reject(err);
deferred.resolve(result);
});
return deferred.promise;
};
const luckyNumber: number = await run();
An alias for Promise.all
Like Promise.all, but limits the numbers of concurrently running items.
Takes an array of functions (that return Promises), rather than an array of Promises
import { PromiseUtils, timer, ms, seconds } from 'swiss-ak';
const give = async (delay: ms, result: number, label: string) => {
await waitFor(delay);
timer.end(label);
return result;
};
timer.start('allLimit', 'a', 'b', 'c', 'd');
const results = PromiseUtils.allLimit<number>(2, [
give(seconds(5), 1, 'a'),
give(seconds(5), 2, 'b'),
give(seconds(5), 3, 'c'),
give(seconds(5), 4, 'd')
]);
timer.end('allLimit');
console.log(results); // [ 1, 2, 3, 4 ]
timer.log();
// Times:
// allLimit: 10s
// a: 5s
// b: 5s
// c: 10s
// d: 10s
Run an async function against each item in an array
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
await PromiseUtils.each<number>(arr, async (val: number) => {
await wait(seconds(2));
sendToSomewhere(val);
});
console.log(''); // after 2 seconds
Run an async function against each item in an array, limiting the number of items that can run concurrently.
See PromiseUtils.allLimit for information about limited functions.
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
await PromiseUtils.eachLimit<number>(2, arr, async (val: number) => {
await wait(seconds(2));
sendToSomewhere(val);
});
console.log(''); // after 4 seconds
Run an async map function against each item in an array, mapping the results to a returned array
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
const mapped = await PromiseUtils.map<number>(arr, async (val: number) => {
await wait(seconds(2));
return val * 2;
});
console.log(mapped); // [2, 4, 6, 8] (after 2 seconds)
Run an async map function against each item in an array, mapping the results to a returned array, and limiting the number of items that can run concurrently.
See PromiseUtils.allLimit for information about limited functions.
import { PromiseUtils, ms, seconds, wait } from 'swiss-ak';
const arr = [1, 2, 3, 4];
const mapped = await PromiseUtils.mapLimit<number>(2, arr, async (val: number) => {
await wait(seconds(2));
return val * 2;
});
console.log(mapped); // [2, 4, 6, 8] (after 4 seconds)
Like Promise.all, but takes/gives an object instead of an array
import { PromiseUtils, timer, ms, seconds } from 'swiss-ak';
const give = async (delay: ms, result: number, label: string) => {
await waitFor(delay);
timer.end(label);
return result;
};
timer.start('allObj', 'a', 'b', 'c');
const results = PromiseUtils.allObj<number>({
a: give(seconds(10), 1, 'a'),
b: give(seconds(15), 2, 'b'),
c: give(seconds(20), 3, 'c')
});
timer.end('allObj');
console.log(results); // { a: 1, b: 2, c: 3 }
timer.log();
// Times:
// allObj: 20s
// a: 10s
// b: 15s
// c: 20s
A mix of allObj and allLimit.
Takes an array of functions (that return Promises), and limits the numbers of concurrently running items.
import { PromiseUtils, timer, ms, seconds } from 'swiss-ak';
const give = async (delay: ms, result: number, label: string) => {
await waitFor(delay);
timer.end(label);
return result;
};
timer.start('allLimitObj', 'a', 'b', 'c', 'd');
const results = PromiseUtils.allLimitObj<number>(2, {
a: give(seconds(5), 1, 'a'),
b: give(seconds(5), 2, 'b'),
c: give(seconds(5), 3, 'c'),
d: give(seconds(5), 4, 'd')
});
timer.end('allLimitObj');
console.log(results); // { a: 1, b: 2, c: 3, d: 4 }
timer.log();
// Times:
// allLimitObj: 10s
// a: 5s
// b: 5s
// c: 10s
// d: 10s
A util for creating a 'visual' progress bar for better representing progress of multiple operations.
All options are optional.
Property | Default | Description |
---|---|---|
prefix | '' | String to show to left of progress bar |
prefixWidth | 1 | Min width of prefix - 10 => Example˽˽˽ |
maxWidth | process.stdout.columns or 100 | The maximum width the entire string may extend |
chalk | nothing | the chalk module, if available |
wrapperFn | nothing | function to wrap the printed string (eg chalk.cyan) |
showPercent | false | Show percentage completed - ( 69%) |
showCount | true | Show numerical values of the count - [11 / 15] |
countWidth | 0 | Min width of nums for showCount - 3 => [˽˽1 / ˽15] |
progChar | '█' | Character to use for progress section of bar |
emptyChar | ' ' | Character to use for empty (rail) section of bar |
startChar | '▕' | Character to start the progress bar with |
endChar | '▏' | Character to end the progress bar with |
import chalk from 'chalk';
import { getProgressBar } from 'swiss-ak';
console.log('-'.repeat(20) + ' < 20 Chars');
const progress = getProgressBar(5, {
prefix: 'ABC',
maxWidth: 20,
chalk,
wrapperFn: chalk.green
});
for (let i = 1; i <= 5; i++) {
progress.set(i);
}
progress.finish();
Output:
-------------------- < 20 Chars
ABC ▕ ▏ [0 / 5]
ABC ▕█ ▏ [1 / 5]
ABC ▕██ ▏ [2 / 5]
ABC ▕████ ▏ [3 / 5]
ABC ▕█████ ▏ [4 / 5]
ABC ▕██████▏ [5 / 5]
Can use instead of console.log
Overwrites the previous line if possible (i.e. node);
import { printLn } from 'swiss-ak';
printLn('A');
printLn('B'); // Replaces the 'A' line
printLn('C'); // Replaces the 'B' line
printLn(); // Jumps a line
printLn('D'); // Replaces the empty line
Output
C
D
These are used in non-vital personal projects and scripts.
Need to be better tested before being used in prod.
Failing/erroring/rejected promises may not behave as expected.
FAQs
A collection of useful little things that I like to reuse across projects
We found that swiss-ak demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.