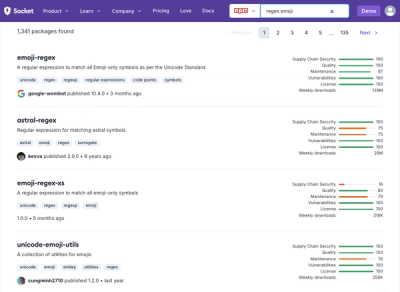
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Synchronous Node.js HTTP for API testing/scripting/automation.
npm install synchttp
This snippet fetches a list of 10 images from Flickr and dump: title, owned and URL. An additional request is performed to fetch the user name.
var synchttp = require(synchttp);
var API_KEY = '<YOUR APP KEY>;
synchttp(function (sh) {
var response = sh
.secure(true)
.host('api.flickr.com')
.path('/services/rest/')
.query({
'api_key': API_KEY,
'method': 'flickr.interestingness.getList',
'format': 'json',
'nojsoncallback': 1,
'per_page': 10
}).get();
response.photos.photo.forEach(function (photo) {
var user = sh.query({
'api_key': API_KEY,
'method': 'flickr.people.getInfo',
'format': 'json',
'nojsoncallback': 1,
'user_id': photo.owner
}).get();
console.log(
'"%s" by "%s"\n[https://www.flickr.com/photos/%s/%s]\n',
photo.title,
user.person.username._content,
photo.owner,
photo.id
);
});
});
Assume you have a dummy API for your blog that allows you to create a post and then to add some tags:
POST /api/posts/ # title, body
POST /api/posts/:post_id/tags/ # label
GET /api/posts/:post_id
In the following snippet, the id of the new post is used to add the tags and to finally fetch the whole post resource.
var synchttp = require('synchttp');
synchttp(function (sh) {
var response = sh.path('/api/posts/').post({
'title': 'Awesome post',
'body': 'Lorem ipsum...'
});
// the path is kept across the following requests...
sh.path('/api/posts/' + response.id + '/tags/');
['nodejs', 'javascript', 'sh'].forEach(function (tag) {
// ... so only the body is needed here!
sh.post({
'label': tag
});
});
var post = sh.path('/api/posts/' + response.id).get();
console.log(JSON.stringify(post, null, 4));
});
This would result in something like:
{
"_id": "53b1ab76b2029860505e2c18",
"title": "Awesome post",
"body": "Lorem ipsum...",
"tags": [
{
"_id": "53b1ab76b2029860505e2c19",
"label": "nodejs"
},
{
"_id": "53b1ab76b2029860505e2c1a",
"label": "javascript"
},
{
"_id": "53b1ab76b2029860505e2c1b",
"label": "http"
}
]
}
Define how raw incoming/outgoing data should be parsed/formatted.
Actually supported:
application/json
or json
;application/x-www-form-urlencoded
or urlencoded
.Single entry point of this module, it runs callback
in a synchronous
environment.
callback
takes an object of type Synchttp
.
This object is used to issue HTTP commands to the server, and it maintains a set of connection parameters so there is no need to repeat parameters that do not change in every request.
synchttp(function (sh) {
sh.host('example.com');
sh.path('/foo').get(); // GET http://example.com/foo
sh.path('/bar').get(); // GET http://example.com/bar
sh.path('/baz').get(); // GET http://example.com/baz
});
Parameters are set by calling method on this object, a sort of builder pattern
can be used. Only the final actions (get
, post
, etc.) actually perform the
communication:
synchttp(function (sh) {
sh.host('example.com').port(1337).path('/foo/bar/baz').post({
'name': 'value'
});
});
Parameters come with a default value, if the corresponding method is called without arguments then the default value is restored:
synchttp(function (sh) {
sh.host('example.com').get(); // GET http://example.com/
sh.host().get(); // GET http://localhost/
});
Default synchttp/<version>
.
Define the User-Agent
to be send along with the HTTP requests.
Default application/json
.
Define the content type to be used when sending messages to the server. For
incoming messages instead the Content-Type
header is used.
Default application/json
.
Define the content type to be used when sending/receiving messages to/from the server.
Default localhost
.
Define the host to be reached.
Default false
.
Define whether a secure connection should be used or not..
Default 80
or 443
according to the secure
parameter.
Define the host port to be reached.
Default /
.
Define the path component of the URL to query.
Default {}
.
Define the key-value pairs to be used as query string.
Default {}
.
Define the key-value pairs to be used as HTTP headers.
Default undefined
(no authentication).
Define the basic HTTP authentication to use.
These methods return the received payload as a JavaScript object according to
the Content-Type
returned by the server (if possible). An exception of type
Error
is thrown in case of errors.
Perform an HTTP GET
.
Perform an HTTP POST
.
Perform an HTTP PUT
.
Perform an HTTP DELETE
.
Send and receive messages through WebSockets; messages are properly
parsed/formatted according to the value of the wsContentType
parameter.
Try to establish a WebSocket to the server. A new WebSocket is created whenever this method is called with a different resulting URL (according to the connection parameters).
Send a message through the WebSocket.
Receive a message from the WebSocket.
FAQs
Synchronous Node.js HTTP for API testing/scripting/automation
The npm package synchttp receives a total of 1 weekly downloads. As such, synchttp popularity was classified as not popular.
We found that synchttp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.