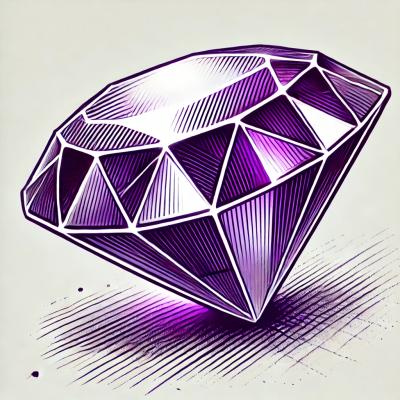
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
The 'tap' npm package is a Test Anything Protocol (TAP) producer for Node.js. It is used for writing tests in JavaScript and provides a simple way to create and run tests, generate reports, and handle assertions.
Basic Test Creation
This feature allows you to create basic tests using the 'tap' module. The example demonstrates a simple test that checks if 1 + 1 equals 2.
const tap = require('tap');
tap.test('basic test', t => {
t.equal(1 + 1, 2, '1 + 1 should equal 2');
t.end();
});
Asynchronous Testing
This feature allows you to write asynchronous tests. The example shows how to use async/await to handle asynchronous operations within a test.
const tap = require('tap');
tap.test('async test', async t => {
const result = await new Promise(resolve => setTimeout(() => resolve(42), 100));
t.equal(result, 42, 'result should be 42');
t.end();
});
Nested Tests
This feature allows you to create nested tests. The example demonstrates a parent test containing a child test, which helps in organizing tests hierarchically.
const tap = require('tap');
tap.test('parent test', t => {
t.test('child test', t => {
t.equal(2 * 2, 4, '2 * 2 should equal 4');
t.end();
});
t.end();
});
Assertions
This feature provides various assertion methods to validate test conditions. The example shows different types of assertions like ok, notOk, equal, and notEqual.
const tap = require('tap');
tap.test('assertions test', t => {
t.ok(true, 'this should be true');
t.notOk(false, 'this should be false');
t.equal(3, 3, '3 should equal 3');
t.notEqual(3, 4, '3 should not equal 4');
t.end();
});
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple and fun. It provides a variety of interfaces (BDD, TDD, etc.) and supports a wide range of reporters. Compared to 'tap', Mocha is more flexible and has a larger community.
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. It works out of the box for most JavaScript projects and provides a rich API for assertions, mocking, and more. Jest is more opinionated and comes with built-in features like snapshot testing, which 'tap' does not offer.
AVA is a test runner for Node.js with a concise API, detailed error output, and process isolation. It runs tests concurrently, which can lead to faster test execution. AVA's approach to concurrency and simplicity makes it different from 'tap', which runs tests sequentially by default.
A TAP test framework for Node.js.
This is a mix-and-match set of utilities that you can use to write test harnesses and frameworks that communicate with one another using the Test Anything Protocol.
It is also a test runner for consuming TAP-generating test scripts, and a framework for writing such scripts.
Write your tests in JavaScript
var tap = require('tap')
// you can test stuff just using the top level object.
// no suites or subtests required.
tap.equal(1, 1, 'check if numbers still work')
tap.notEqual(1, 2, '1 should not equal 2')
// also you can group things into sub-tests.
// Sub-tests will be run in sequential order always,
// so they're great for async things.
tap.test('first stuff', function (t) {
t.ok(true, 'true is ok')
t.similar({a: [1,2,3]}, {a: [1,2,3]})
// call t.end() when you're done
t.end()
})
// you can specify a 'plan' if you know how many
// tests there will be in advance. Handy when
// order is irrelevant and things happen in parallel.
tap.test('planned test', function (t) {
t.plan(2)
setTimeout(function () {
t.ok(true, 'a timeout')
})
setTimeout(function () {
t.ok(true, 'b timeout')
})
})
// you can do `var test = require('tap').test` if you like
// it's pre-bound to the root tap object.
var test = require('tap').test
// subtests can have subtests
test('parent', function (t) {
t.test('child', function (tt) {
tt.throws(function () {
throw new Error('fooblz')
}, {
message: 'fooblz'
}, 'throw a fooblz')
tt.throws(function () { throw 1 }, 'throw whatever')
tt.end()
})
t.end()
})
// thrown errors just fail the current test, so you can
// also use your own assert library if you like.
// Of course, this means it won't be able to print out the
// number of passing asserts, since passes are silent.
test('my favorite assert lib', function (t) {
var assert = require('assert')
assert.ok(true, 'true is ok')
assert.equal(1, 1, 'math works')
// Since it can't read the plan, using a custom assert lib
// means that you MUST use t.end()
t.end()
})
// You can mark tests as 'todo' either using a conf object,
// or simply by omitting the callback.
test('solve halting problem')
test('prove p=np', { todo: true }, function (t) {
// i guess stuff goes here
t.fail('traveling salesmen must pack their own bags')
t.end()
})
// Prefer mocha/rspec/lab style global objects?
// Got you covered. This is a little experimental,
// patches definitely welcome.
tap.mochaGlobals()
describe('suite ride bro', function () {
it('should have a wheel', function () {
assert.ok(thingie.wheel, 'wheel')
})
it('can happen async', function (done) {
setTimeout(function () {
assert.ok('ok')
done()
})
})
})
// Read on for a complete list of asserts, methods, etc.
You can run tests using the tap
executable. Put this in your
package.json file:
{
"scripts": {
"test": "tap test/*.js"
}
}
and then you can run npm test
to run your test scripts.
Command line behavior and flags:
$ tap -h
Usage:
tap [options] <files>
Executes all the files and interprets their output as TAP
formatted test result data.
To parse TAP data from stdin, specify "-" as a filename.
Options:
-c --color Force use of colors
-C --no-color Force no use of colors
-R<type> --reporter=<type> Use the specified reporter. Defaults to
'classic' when colors are in use, or 'tap'
when colors are disabled.
Available reporters:
classic doc dot dump html htmlcov json
jsoncov jsonstream landing list markdown
min nyan progress silent spec tap xunit
-gc --expose-gc Expose the gc() function to Node tests
--debug Run JavaScript tests with node --debug
--debug-brk Run JavaScript tests with node --debug-brk
--harmony Enable all Harmony flags in JavaScript tests
--strict Run JS tests in 'use strict' mode
-t<n> --timeout=<n> Time out tests after this many seconds.
Defaults to 120, or the value of the
TAP_TIMEOUT environment variable.
-h --help print this thing you're looking at
-v --version show the version of this program
-- Stop parsing flags, and treat any additional
command line arguments as filenames.
The root tap
object is an instance of the Test class with a few
slight modifications.
plan()
and test()
methods are pre-bound onto the root
object, so that you don't have to call them as methods.The Test
class is the main thing you'll be touching when you use
this module.
The most common way to instantiate a Test
object by calling the
test
method on the root or any other Test
object. The callback
passed to test(name, fn)
will receive a child Test
object as its
argument.
A Test
object is a Readable Stream. Child tests automatically send
their data to their parent, and the root require('tap')
object pipes
to stdout by default. However, you can instantiate a Test
object
and then pipe it wherever you like. The only limit is your imagination.
Create a subtest.
If the function is omitted, then it will be marked as a "todo" or "pending" test.
The options object is the same as would be passed to any assert.
Specify that a given number of tests are going to be run.
This may only be called before running any asserts or child tests.
Call when tests are done running. This is not necessary if t.plan()
was used.
Pull the proverbial ejector seat.
Use this when things are severely broken, and cannot be reasonably handled. Immediately terminates the entire test run.
Return true if everything so far is ok.
Note that all assert methods also return true
if they pass.
Print the supplied message as a TAP comment.
Note that you can always use console.error()
for debugging (or
console.log()
as long as the message doesn't look like TAP formatted
data).
Emit a failing test point. This method, and pass()
, are the basic
building blocks of all fancier assertions.
Emit a passing test point. This method, and fail()
, are the basic
building blocks of all fancier assertions.
These methods are primarily for internal use, but can be handy in some unusual situations. If you find yourself using them frequently, you may be Doing It Wrong. However, if you find them useful, you should feel perfectly comfortable using them.
Parse standard input as if it was a child test named /dev/stdin
.
This is primarily for use in the test runner, so that you can do
some-tap-emitting-program | tap other-file.js - -Rnyan
.
Sometimes, instead of running a child test directly inline, you might want to run a TAP producting test as a child process, and treat its standard output as the TAP stream.
That's what this method does.
It is primarily used by the executable runner, to run all of the filename arguments provided on the command line.
This is used for creating assertion methods on the Test
class.
It's a little bit advanced, but it's also super handy sometimes. All of the assert methods below are created using this function, and it can be used to create application-specific assertions in your tests.
The name is the method name that will be created. The length is the
number of arguments the assertion operates on. (The message
and
extra
arguments will alwasy be appended to the end.)
For example, you could have a file at test/setup.js
that does the
following:
var tap = require('tap')
// convenience
if (module === require.main) {
tap.pass('ok')
return
}
// Add an assertion that a string is in Title Case
// It takes one argument (the string to be tested)
tap.Test.prototype.addAssert('titleCase', 1, function (str, message, extra) {
message = message || 'should be in Title Case'
// the string in Title Case
// A fancier implementation would avoid capitalizing little words
// to get `Silence of the Lambs` instead of `Silence Of The Lambs`
// But whatever, it's just an example.
var tc = str.toLowerCase().replace(/\b./, function (match) {
return match.toUpperCase()
})
// should always return another assert call, or
// this.pass(message) or this.fail(message, extra)
return this.equal(str, tc, message, extra)
})
Then in your individual tests, you'd do this:
require('./setup.js') // adds the assert
var tap = require('tap')
tap.titleCase('This Passes')
tap.titleCase('however, tHis tOTaLLy faILS')
Call the end()
method on all child tests, and then on this one.
Return the currently active test.
The Test
object has a collection of assertion methods, many of which
are given several synonyms for compatibility with other test runners
and the vagaries of human expectations and spelling. When a synonym
is multi-word in camelCase
the corresponding lower case and
snake_case
versions are also created as synonyms.
All assertion methods take optional message
and extra
arguments as
the last two params. The message
is the name of the test. The
extra
argument can contain any arbitrary data about the test, but
the following fields are "special".
todo
Set to boolean true
or a String to mark this as pendingskip
Set to boolean true
or a String to mark this as skippedat
Generated by the framework. The location where the assertion
was called. Do not set.stack
Generated by the framework. The stack trace to the point
where the assertion was called.Verifies that the object is truthy.
Synonyms: t.true
, t.assert
Verifies that the object is not truthy.
Synonyms: t.false
, t.assertNot
If the object is an error, then the assertion fails.
Note: if an error is encountered unexpectedly, it's often better to simply throw it. The Test object will handle this as a failure.
Synonyms: t.ifErr
, t.ifError
Expect the function to throw an error. If an expected error is provided, then also verify that the thrown error matches the expected error.
Synonyms: t.throw
Verify that the provided function does not throw.
Note: if an error is encountered unexpectedly, it's often better to simply throw it. The Test object will handle this as a failure.
Synonyms: t.notThrow
Verify that the object found is exactly the same (that is, ===
) to
the object that is wanted.
Synonyms: t.equals
, t.isEqual
, t.is
, t.strictEqual
,
t.strictEquals
, t.strictIs
, t.isStrict
, t.isStrictly
Inverse of equal()
.
Verify that the object found is not exactly the same (that is, !==
) as
the object that is wanted.
Synonyms: t.inequal
, t.notEqual
, t.notEquals
,
t.notStrictEqual
, t.notStrictEquals
, t.isNotEqual
, t.isNot
,
t.doesNotEqual
, t.isInequal
Verify that the found object is deeply equivalent to the wanted
object. Use non-strict equality for scalars (ie, ==
).
Synonyms: t.equivalent
, t.looseEqual
, t.looseEquals
,
t.deepEqual
, t.deepEquals
, t.isLoose
, t.looseIs
Inverse of same()
.
Verify that the found object is not deeply equivalent to the
unwanted object. Uses non-strict inequality (ie, !=
) for scalars.
Synonyms: t.inequivalent
, t.looseInequal
, t.notDeep
,
t.deepInequal
, t.notLoose
, t.looseNot
Strict version of same()
.
Verify that the found object is deeply equivalent to the wanted
object. Use strict equality for scalars (ie, ===
).
Synonyms: t.strictEquivalent
, t.strictDeepEqual
, t.sameStrict
,
t.deepIs
, t.isDeeply
, t.isDeep
, t.strictDeepEquals
Inverse of strictSame()
.
Verify that the found object is not deeply equivalent to the unwanted
object. Use strict equality for scalars (ie, ===
).
Synonyms: t.strictInequivalent
, t.strictDeepInequal
,
t.notSameStrict
, t.deepNot
, t.notDeeply
, t.strictDeepInequals
,
t.notStrictSame
Verify that the found object matches the pattern provided.
If pattern is a regular expression, and found is a string, then verify that the string matches the pattern.
If pattern is an object, the verify that the found object contains all the keys in the pattern, and that all of the found object's values match the corresponding fields in the pattern.
This is useful when you want to verify that an object has a certain set of required fields, but additional fields are ok.
Synonyms: t.has
, t.hasFields
, t.matches
, t.similar
, t.like
,
t.isLike
, t.includes
, t.include
Interse of match()
Verify that the found object does not match the pattern provided.
Synonyms: t.dissimilar
, t.unsimilar
, t.notSimilar
, t.unlike
,
t.isUnlike
, t.notLike
, t.isNotLike
, t.doesNotHave
,
t.isNotSimilar
, t.isDissimilar
Verify that the object is of the type provided.
Type can be a string that matches the typeof
value of the object, or
the string name of any constructor in the object's prototype chain, or
a constructor function in the object's prototype chain.
For example, all the following will pass:
t.type(new Date(), 'object')
t.type(new Date(), 'Date')
t.type(new Date(), Date)
Synonyms: t.isa
, t.isA
FAQs
A Test-Anything-Protocol library for JavaScript
The npm package tap receives a total of 244,177 weekly downloads. As such, tap popularity was classified as popular.
We found that tap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.