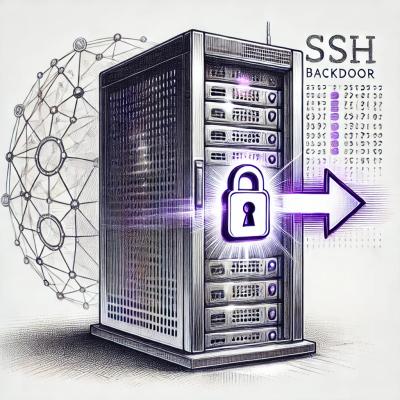
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Javascript implementation of the Tar archive utility. The goal is to make tar JavaScript friendly by incorporating such ideals as:
The Tar object is actually a NodeJS stream, so it supports the usual events: data, error, and end. Since it is a stream, this module can either be simply piped to standard out or to a file, or the chunks can be processed as they come. This makes it really convenient when working with webservers.
No compression is used, so an external compression library is necessary. This is by design and not likely to be implemented.
npm install tar-async
The only external module that Tar uses is futures, and only the forEachAsync method at that. This module will add to the Array prototype, so any for..in loops will need to be converted to forEach loops. This module is used to allow for graceful handling of asynchronous calls in a forEach loop.
This module can be installed from npm:
npm install futures
Tar also requires the built-in fs and stream modules.
This tar utility inherits from stream, so any methods that work with stream will work with this utility. Tar archives are processed in 10KiB chunks. No compression is applied because the libraries for compression are not stable enough for my liking, but that is easily implemented.
The constructor takes an array of options as its only parameter:
addHidden
- add hidden files to archive (default false)
consolidate
- consolidates files into a single directory (default false)
normalize
- normalize each file before archiving (default true)recurse
- recursively add files to archive (default true)output
- output stream (default undefined)
Files can be added to the archive until the close function is called. There are three functions that add data to the archive:
There are a few examples in the examples directory, but if you are lazy, here are a couple brief examples.
To tar all of the files in a directory:
var Tar = require('tar'),
options = {
output: process.stdout
},
tape = new Tar(options);
tape.addDirectory("./", function (err) {
if (err) {
throw err;
}
tape.close();
});
To tar a bunch of random files together:
var Tar = require('./tar'),
options = {
output: process.stdout
},
tape = new Tar(),
files;
files = [
"./tar.js",
"./header.js"
];
tape.addFiles(files, function (err) {
if (err) {
throw err;
}
tape.close();
});
All files that are tarred will preserve the directory structure of the path of each file that was given unless otherwise specified in the options. The addFiles method requires the full path on the file.
Stores the header format and a method for formatting the header:
The header is an array with two properties in each element:
Each field in the header is a string of ASCII characters (one byte each).
Exports a Tar object with several public methods:
Utilities to make life more convenient:
FAQs
Asynchronous tar and untar
The npm package tar-async receives a total of 98 weekly downloads. As such, tar-async popularity was classified as not popular.
We found that tar-async demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.