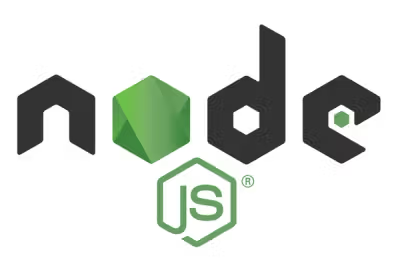
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
task-handler
Advanced tools
A simple, dependency-free Task Manager to make handling of your Javascript Timers easier to work with.
Combined with pubchan, provides a lightweight and powerful toolkit for managing asynchronous coordination of your applications events.
Note: Documentation is currently a work in progress. However, the code itself is at a stable level and used in production. This module has been lifted from our private repositories and released to the public.
yarn add task-handler
or
npm install --save task-handler
Proudly built with 100% Flow Coverage and exported .flow.js files so your flow projects will benefit!
We strongly recommend you look over the types in the source. This will give you an idea of how the various pieces of the package work.
Note: There are certain things Flow is not capable of providing type coverage for, such as try/catch blocks. These are not included in our assessment of "100% Coverage".
/* @flow */
import createTaskHandler from 'task-handler';
const task = createTaskHandler('simple');
// after timeout
task.after('task:one', 3000, () => log('task:one execute'));
// every interval, execute
task.every('task:two', 3000, () => log('task:two execute'));
// immediately execute (nextTick, immediate, timeout priority - first found)
task.defer('task:four', () => log('task:four execute'));
// every interval and immediately (defer), execute
task.everyNow('task:three', 3000, () => log('task:three execute'));
// clear all tasks, killing the event queue and completing execution
task.after('complete', 10000, () => {
log('complete - clearing tasks');
task.clear();
});
For more examples you can check out the examples directory
createTaskHandler
(Function) (default)A factory for building and retrieving TaskHandler
instances. If an id
is
provided as the functions argument, it will return a TaskHandler
with the
given id. If that TaskHandler
was previously created, it returns it, otherwise
it creates a new instance and returns that.
import createTaskHandler from 'task-handler';
const task = createTaskHandler();
declare function createTaskHandler(id?: string): TaskHandler;
TaskHandler
(Class)Documentation coming soon...
// public interface for TaskHandler
class TaskHandler {
// how many scheduled tasks of any type do we have?
get size(): number
// create a timeout, cancelling any timeouts
// currently scheduled with the given id if any
after(
id: TaskID,
delay: number,
fn: CallbackFn,
...args: Array<any>
): CallbackRef
defer(id: TaskID, fn: CallbackFn, ...args: Array<any>): CallbackRef
every(
id: TaskID,
interval: number,
fn: CallbackFn,
...args: Array<any>
): CallbackRef
everyNow(
id: TaskID,
interval: number,
fn: CallbackFn,
...args: Array<any>
): CallbackRef
// cancel the given timeout (optionally provide a type if it should only
// be cancelled if its of the given type).
// returns true if a task was cancelled.
cancel(id: TaskID, type?: TaskTypes): boolean
// cancel all of a given type (or all if no argument provided)
clear(...types: Array<TaskTypes>): void
// are the given tasks currently scheduled? returns true if all tasks
// given are present.
has(...ids: Array<TaskID>): boolean
}
TaskRef
(Object)type TaskID = string;
type TaskCancelFunction = () => boolean;
type WhileConditionFn = (ref: CallbackRef, ...args: Array<*>) => boolean;
type CallbackRef = {|
+task: TaskHandler,
+id: TaskID,
+cancel: TaskCancelFunction,
+while: (condition: WhileConditionFn) => void,
|};
FAQs
Handle Javascript Timers like a boss! https://odo-network.github.io/task-handler/
We found that task-handler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.