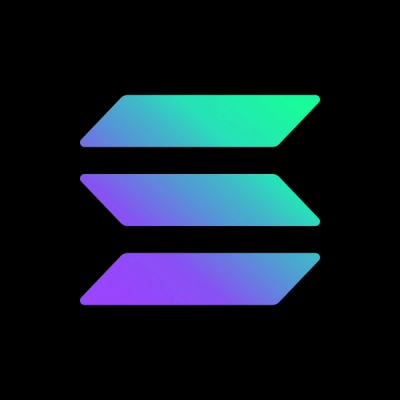
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
teen_process
Advanced tools
The teen_process npm package provides utilities for executing system commands and managing child processes in Node.js. It simplifies the process of running shell commands, handling their output, and managing subprocesses.
exec
The `exec` function allows you to run shell commands and capture their output. In this example, the `ls -la` command is executed, and its output is logged to the console.
const { exec } = require('teen_process');
async function runCommand() {
try {
const { stdout, stderr } = await exec('ls', ['-la']);
console.log('Output:', stdout);
console.error('Error:', stderr);
} catch (err) {
console.error('Command failed:', err);
}
}
runCommand();
spawn
The `spawn` function is used to launch a new process with a given command. This example demonstrates how to spawn the `ls -la` command and handle its stdout, stderr, and exit events.
const { spawn } = require('teen_process');
function runSpawn() {
const child = spawn('ls', ['-la']);
child.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
child.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
child.on('close', (code) => {
console.log(`child process exited with code ${code}`);
});
}
runSpawn();
execFile
The `execFile` function is similar to `exec`, but it is specifically designed for executing files. In this example, the `node -v` command is executed to get the Node.js version.
const { execFile } = require('teen_process');
async function runExecFile() {
try {
const { stdout, stderr } = await execFile('node', ['-v']);
console.log('Node version:', stdout);
console.error('Error:', stderr);
} catch (err) {
console.error('Command failed:', err);
}
}
runExecFile();
The `child_process` module is a core Node.js module that provides similar functionality to `teen_process`. It allows you to spawn new processes, execute commands, and handle their output. However, `teen_process` offers a more user-friendly API and additional features like promise-based execution.
The `execa` package is a popular alternative to `teen_process` for executing shell commands in Node.js. It provides a promise-based API, better error handling, and more features for managing child processes. Compared to `teen_process`, `execa` is more feature-rich and widely used in the community.
The `shelljs` package provides a portable (Windows/Linux/OS X) implementation of Unix shell commands in Node.js. It allows you to run shell commands and scripts with a simple API. While `shelljs` focuses on providing a shell-like environment, `teen_process` is more focused on managing child processes and executing commands.
A grown-up version of Node's child_process. exec
is really useful, but it
suffers many limitations. This is an es7 (async
/await
) implementation of
exec
that uses spawn
under the hood. It takes care of wrapping commands and
arguments so we don't have to care about escaping spaces. It can also return
stdout/stderr even when the command fails, or times out. Importantly, it's also
not susceptible to max buffer issues.
Examples:
import { exec } from 'teen_process';
// basic usage
let {stdout, stderr, code} = await exec('ls', ['/usr/local/bin']);
console.log(stdout.split("\n")); // array of files
console.log(stderr); // ''
console.log(code); // 0
// works with spaces
await exec('/command/with spaces.sh', ['foo', 'argument with spaces'])
// as though we had run: "/command/with spaces.sh" foo "argument with spaces"
// nice error handling that still includes stderr/stdout/code
try {
await exec('echo_and_exit', ['foo', '10']);
} catch (e) {
console.log(e.message); // "Exited with code 10"
console.log(e.stdout); // "foo"
console.log(e.code); // 10
}
The exec
function takes some options, with these defaults:
{
cwd: undefined,
env: process.env,
timeout: null,
killSignal: 'SIGTERM',
encoding: 'utf8',
ignoreOutput: false
}
Most of these are self-explanatory. ignoreOutput
is useful if you have a very
chatty process whose output you don't care about and don't want to add it to
the memory consumed by your program.
Example:
try {
await exec('sleep', ['10'], {timeout: 500, killSignal: 'SIGINT'});
} catch (e) {
console.log(e.message); // "'sleep 10' timed out after 500ms"
}
FAQs
A grown up version of Node's spawn/exec
The npm package teen_process receives a total of 420,359 weekly downloads. As such, teen_process popularity was classified as popular.
We found that teen_process demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.