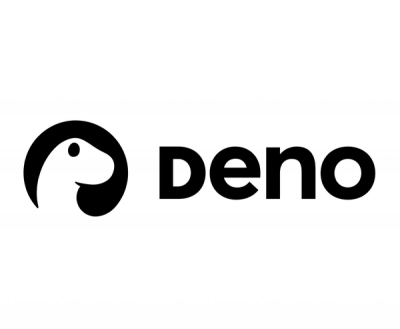
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
telenode-js
Advanced tools
Lightweight Telegram API framework for Node.js
npm install telenode-js
In order to listen to updates from Telegram servers you have to set up a webhook.
The webhook url will be stored in a .env
file in the root of your project as WEBHOOK=https://your_amazing_webhook.com
.
Then you can execute the following command:
npx set-webhook
const Telenode = require('telenode-js');
require('dotenv').config();
const bot = new Telenode({
apiToken: process.env.API_TOKEN,
});
bot.createServer();
bot.onTextMessage('hello', async (messageBody) => {
console.log(messageBody);
await bot.sendTextMessage('hello back', messageBody.chat.id);
});
In this example the bot will listen only to 'hello' text messages and will respond to the user 'hello back'. Any other message will be ignored.
Additional examples can be found in the examples folder.
Each feature of Telenode
is demonstrated in an example file inside the examples
folder.
For local development you need to set a webhook as well with the set-webhook
command. How you execute the command is slightly different from using the installed package like explained above. Instead of npx
just use npm run
:
npm run set-webhook
The webhook url should be presented in the .env
file or be exported as an environment variable.
In order to develop a new feature or to run an existing one you should use the dev
command from the package.json
with the --file
flag like so:
npm run dev --file=<example>
✅ Explicit messages handlers
✅ Fallback messages handler (empty string)
✅ Regex matching on text messages
✅ Buttons support (inline keyboard, reply keyboard and remove reply keyboard)
FAQs
Lightweight Telegram API framework for Node.js
The npm package telenode-js receives a total of 944 weekly downloads. As such, telenode-js popularity was classified as not popular.
We found that telenode-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.