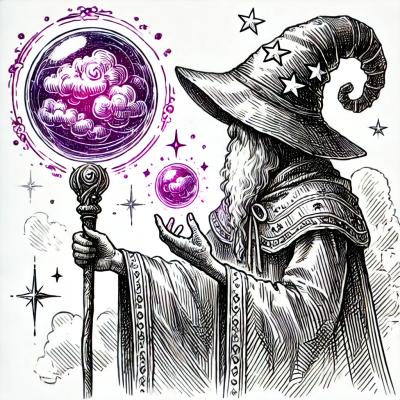
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Render templates from any engine. Make custom template types, use layouts on pages, partials or any custom template type, custom delimiters, helpers, middleware, routes, loaders, and lots more. Powers Assemble v0.6.0, Verb v0.3.0 and your application.
Render templates from any engine. Make custom template types, use layouts on pages, partials or any custom template type, custom delimiters, helpers, middleware, routes, loaders, and lots more. Powers Assemble v0.6.0, Verb v0.3.0 and your application.
Go to the API documentation
page
, layout
and partial
, but you can create special types for any use case.npm i template --save
var Template = require('template');
var template = new Template();
template.page('home.tmpl', 'This home page.');
// add locals
template.page('home.tmpl', 'The <%= title %> page', {title: 'home'});
Using the default Lo-Dash engine:
template.render('home.tmpl', function(err, html) {
if (err) throw err;
console.log(html); //=> 'The home page.'
});
Or you can pass a string (non-cached template):
template.render('foo bar', function(err, html) {
if (err) throw err;
console.log(html); //=> 'foo bar'
});
Locals
Pass locals
as the second parameter:
template.render('foo <%= bar %>', {bar: 'baz'}, function(err, html) {
if (err) throw err;
console.log(html); //=> 'foo baz'
});
Register
Examples
// use handlebars to render templates with the `.hbs` extension
template.engine('hbs', require('engine-handlebars'));
// use lo-dash to render templates with the `.tmpl` extension
template.engine('tmpl', require('engine-lodash'));
Using consolidate.js
You can also use consolidate:
var consolidate = require('consolidate');
template.engine('hbs', consolidate.handlebars);
template.engine('tmpl', consolidate.lodash);
Using a custom function
Render .less
files:
var less = require('less');
template.engine('less', function(str, options, cb) {
try {
less.render(str, options, function (err, res) {
if (err) { return cb(err); }
cb(null, res.css);
});
} catch (err) { return cb(err); }
});
You can also use engine-less.
As glob patterns:
template.pages('pages/*.hbs');
template.pages(['partials/*.hbs', 'includes/*.hbs']);
As key/value pairs:
template.page('home', 'This is home.');
template.page('home', 'This is <%= title %>.', {title: 'home'});
template.page('home', {content: 'This is home.'});
template.page('home', {content: 'This is <%= title %>.', title: 'home'});
template.page('home', {content: 'This is <%= title %>.'}, {title: 'home'});
Note any of the above examples will work with either the singular or plural methods (e.g. page/pages)
Built-in template types are:
page
: the default renderable
template typelayout
: the default layout
template typepartial
: the default partial
template typeIf you need something different, add your own:
template.create('post', { isRenderable: true, isPartial: true });
template.create('section', { isLayout: true });
template.create('include', { isPartial: true });
Setting isRenderable
, isLayout
and isPartial
will add special convenience methods to the new template type. For example, when isRenderable
is true, any templates registered for that that type can be rendered directly by passing the name of a template to the .render()
method.
Loading custom templates
We can now load posts using the .post()
or .posts()
methods, the same way that pages or other default templates are loaded:
template.posts('my-blog-post', 'This is content...');
Note: if you create a new template type with a weird plural form, like cactus
, you can pass cacti
as a second arg. e.g. template.create('cactus', 'cactii')
post
will belong to both the renderable
and partial
types. This means that posts
can be used as partials, and they will be "findable" on the cache by the render methods. Renderable templates also get their own render methods, but more on that later.section
will belong to the layout
type. This means that any section
template can be used as a layout for other templates.include
will belong to the partial
type. This means that any include
template can be used as partial by other templates.Every template subtype uses a built-in loader to load and/or resolve templates. However, if you need something different, just add your own.
Pass an array of functions, each can take any arguments, but the last must pass an object to the callback:
template.create('component', { isPartial: true },
function (filepath, next) {
var str = fs.readFileSync(filepath, 'utf8');
var file = {};
file[filepath] = {path: filepath, content: str};
return file;
}
);
Now, every component
will use this loader.
template.component('components/navbar.html');
//=> {'components/navbar.html': {path: 'components/navbar.html', content: '...'}};
When the last argument passed to a template is an array, or more specifically an array of functions, that array will be concatenated to the loader array for the template's subtype.
Example
template.component('components/navbar.html',
function(file) {
file.data = {foo: 'bar'};
return file;
}
})
//=> {navbar: {path: 'components/navbar.html', content: '...', data: {foo: 'bar'}}};
As mentioned in the previous section, loader functions may take any arguments long as the last function returns a valid template object.
Valid template object
A valid template object is a key/value pair that looks like this:
// {key: value}
{'foo.txt': {content: 'this is content'}};
key
{String}: the unique identifier for the template. Usually a name or the filepath that was used for loading the templatevalue
{Object}: the actual template object, value
must have the following properties:
content
{String}: the string to be renderedAny additional properties may be added. Useful ones are:
path
{String}: If present, can be used to determine engines, delimiters, etc.ext
{String}: Like path
, can be used to determine engines, delimiters, etc.options
{Object}: If present, options are passed to engines, and can also be useful in determining engines, delimiters, etc.locals
{Object}: data to pass to templatesInstall devDependencies:
npm i -d && verb
Install devDependencies:
npm i -d && verb test
TBC...
Jon Schlinkert
Brian Woodward
Copyright (c) 2014-2015 Jon Schlinkert
Released under the MIT license
This file was generated by verb on February 13, 2015.
FAQs
Render templates using any engine. Supports, layouts, pages, partials and custom template types. Use template helpers, middleware, routes, loaders, and lots more. Powers assemble, verb and other node.js apps.
The npm package template receives a total of 0 weekly downloads. As such, template popularity was classified as not popular.
We found that template demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.