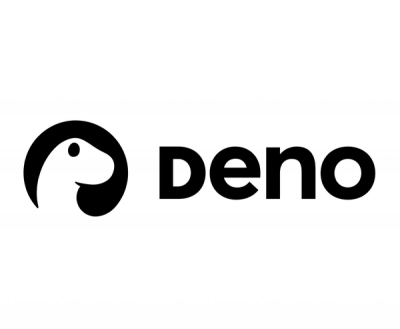
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
templates
Advanced tools
System for creating and managing template collections, and rendering templates with any node.js template engine. Can be used as the basis for creating a static site generator or blog framework.
System for creating and managing template collections, and rendering templates with any node.js template engine. Can be used as the basis for creating a static site generator or blog framework.
Features
app.create('foo')
Example
This is just a very small glimpse at the templates
API!
var templates = require('templates');
var app = templates();
// create a collection
app.create('pages');
// add views to the collection
app.page('a.html', {content: 'this is <%= foo %>'});
app.page('b.html', {content: 'this is <%= bar %>'});
app.page('c.html', {content: 'this is <%= baz %>'});
app.pages.getView('a.html')
.render({foo: 'home'}, function (err, view) {
//=> 'this is home'
});
(Table of contents generated by verb)
Install with npm
$ npm i templates --save
var templates = require('templates');
This function is the main export of the templates module. Initialize an instance of templates
to create your application.
Params
options
{Object}Example
var templates = require('templates');
var app = templates();
Run a plugin on the instance. Plugins are invoked immediately upon creating the collection in the order in which they were defined.
Params
fn
{Function}: Plugin function. If the plugin returns a function it will be passed to the use
method of each collection created on the instance.returns
{Object}: Returns the instance for chaining.Example
var app = assemble()
.use(require('foo'))
.use(require('bar'))
.use(require('baz'))
Set, get and load data to be passed to templates as context at render-time.
Params
key
{String|Object}: Pass a key-value pair or an object to set.val
{any}: Any value when a key-value pair is passed. This can also be options if a glob pattern is passed as the first value.returns
{Object}: Returns an instance of Templates
for chaining.Example
app.data('a', 'b');
app.data({c: 'd'});
console.log(app.cache.data);
//=> {a: 'b', c: 'd'}
Create a new view collection. View collections are decorated with special methods for getting, setting and rendering views from that collection. Collections created with this method are not stored on app.views
as with the create method.
Params
opts
{Object}: Collection optionsreturns
{Object}: Returns the collection
instance for chaining.Example
var collection = app.collection();
collection.addViews({...}); // add an object of views
collection.addView('foo', {content: '...'}); // add a single view
// collection methods are chainable too
collection.addView('home.hbs', {content: 'foo <%= title %> bar'})
.render({title: 'Home'}, function(err, res) {
//=> 'foo Home bar'
});
Create a new view collection that is stored on the app.views
object. For example, if you create a collection named posts
:
posts
will be stored on app.views.posts
post
method will be added to app
, allowing you to add a single view to the posts
collection using app.post()
(equivalent to collection.addView()
)posts
method will be added to app
, allowing you to add views to the posts
collection using app.posts()
(equivalent to collection.addViews()
)Params
name
{String}: The name of the collection. Plural or singular form.opts
{Object}: Collection optionsloaders
{String|Array|Function}: Loaders to use for adding views to the created collection.returns
{Object}: Returns the collection
instance for chaining.Example
app.create('posts');
app.posts({...}); // add an object of views
app.post('foo', {content: '...'}); // add a single view
// collection methods are chainable too
app.post('home.hbs', {content: 'foo <%= title %> bar'})
.render({title: 'Home'}, function(err, res) {
//=> 'foo Home bar'
});
Find a view by name
, optionally passing a collection
to limit the search. If no collection is passed all renderable
collections will be searched.
Params
name
{String}: The name/key of the view to findcolleciton
{String}: Optionally pass a collection name (e.g. pages)returns
{Object|undefined}: Returns the view if found, or undefined
if not.Example
var page = app.find('my-page.hbs');
// optionally pass a collection name as the second argument
var page = app.find('my-page.hbs', 'pages');
Get view key
from the specified collection
.
Params
collection
{String}: Collection name, e.g. pages
key
{String}: Template namefn
{Function}: Optionally pass a renameKey
functionreturns
{Object}Example
var view = app.getView('pages', 'a/b/c.hbs');
// optionally pass a `renameKey` function to modify the lookup
var view = app.getView('pages', 'a/b/c.hbs', function(fp) {
return path.basename(fp);
});
Get all views from a collection
using the collection's singular or plural name.
Params
name
{String}: The collection name, e.g. pages
or page
returns
{Object}Example
var pages = app.getViews('pages');
//=> { pages: {'home.hbs': { ... }}
var posts = app.getViews('posts');
//=> { posts: {'2015-10-10.md': { ... }}
Returns the first view from collection
with a key that matches the given glob pattern.
Params
collection
{String}: Collection name.pattern
{String}: glob patternoptions
{Object}: options to pass to micromatchreturns
{Object}Example
var pages = app.matchView('pages', 'home.*');
//=> {'home.hbs': { ... }, ...}
var posts = app.matchView('posts', '2010-*');
//=> {'2015-10-10.md': { ... }, ...}
Returns any views from the specified collection with keys that match the given glob pattern.
Params
collection
{String}: Collection name.pattern
{String}: glob patternoptions
{Object}: options to pass to micromatchreturns
{Object}Example
var pages = app.matchViews('pages', 'home.*');
//=> {'home.hbs': { ... }, ...}
var posts = app.matchViews('posts', '2010-*');
//=> {'2015-10-10.md': { ... }, ...}
Handle a middleware method
for view
.
Params
method
{String}: Name of the router method to handle. See router methodsview
{Object}: View objectcallback
{Function}: Callback functionreturns
{Object}Example
app.handle('customMethod', view, callback);
Create a new Route for the given path. Each route contains a separate middleware stack.
See the [route API documentation][route-api] for details on adding handlers and middleware to routes.
Params
path
{String}returns
{Object} Route
: for chainingExample
app.create('posts');
app.route(/blog/)
.all(function(view, next) {
// do something with view
next();
});
app.post('whatever', {path: 'blog/foo.bar', content: 'bar baz'});
Special route method that works just like the router.METHOD()
methods, except that it matches all verbs.
Params
path
{String}callback
{Function}returns
{Object} this
: for chainingExample
app.all(/\.hbs$/, function(view, next) {
// do stuff to view
next();
});
Add callback triggers to route parameters, where name
is the name of the parameter and fn
is the callback function.
Params
name
{String}fn
{Function}returns
{Object}: Returns the instance of Templates
for chaining.Example
app.param('title', function (view, next, title) {
//=> title === 'foo.js'
next();
});
app.onLoad('/blog/:title', function (view, next) {
//=> view.path === '/blog/foo.js'
next();
});
Register a view engine callback fn
as ext
.
Params
exts
{String|Array}: String or array of file extensions.fn
{Function|Object}: or settings
settings
{Object}: Optionally pass engine options as the last argument.Example
app.engine('hbs', require('engine-handlebars'));
// using consolidate.js
var engine = require('consolidate');
app.engine('jade', engine.jade);
app.engine('swig', engine.swig);
// get a registered engine
var swig = app.engine('swig');
Compile content
with the given locals
.
Params
view
{Object|String}: View object.locals
{Object}isAsync
{Boolean}: Load async helpersreturns
{Object}: View object with fn
property with the compiled function.Example
var indexPage = app.page('some-index-page.hbs');
var view = app.compile(indexPage);
// view.fn => [function]
// you can call the compiled function more than once
// to render the view with different data
view.fn({title: 'Foo'});
view.fn({title: 'Bar'});
view.fn({title: 'Baz'});
Render a view with the given locals
and callback
.
Params
view
{Object|String}: Instance of View
locals
{Object}: Locals to pass to template engine.callback
{Function}Example
var blogPost = app.post.getView('2015-09-01-foo-bar');
app.render(blogPost, {title: 'Foo'}, function(err, view) {
// `view` is an object with a rendered `content` property
});
Returns a new view, using the View
class currently defined on the instance.
Params
key
{String|Object}: View key or objectvalue
{Object}: If key is a string, value is the view object.returns
{Object}: returns the view
objectExample
var view = app.view('foo', {conetent: '...'});
// or
var view = app.view({path: 'foo', conetent: '...'});
Register a template helper.
Params
name
{String}: Helper namefn
{Function}: Helper function.Example
app.helper('upper', function(str) {
return str.toUpperCase();
});
Register multiple template helpers.
Params
helpers
{Object|Array}: Object, array of objects, or glob patterns.Example
app.helpers({
foo: function() {},
bar: function() {},
baz: function() {}
});
Get or set an async helper. If only the name is passed, the helper is returned.
Params
name
{String}: Helper name.fn
{Function}: Helper functionExample
app.asyncHelper('upper', function(str, next) {
next(null, str.toUpperCase());
});
Register multiple async template helpers.
Params
helpers
{Object|Array}: Object, array of objects, or glob patterns.Example
app.asyncHelpers({
foo: function() {},
bar: function() {},
baz: function() {}
});
Create an instance of Views
with the given options
.
Params
options
{Object}Example
var collection = new Views();
collection.addView('foo', {content: 'bar'});
Run a plugin on the collection instance. Plugins are invoked immediately upon creating the collection in the order in which they were defined.
Params
fn
{Function}: Plugin function. If the plugin returns a function it will be passed to the use
method of each view created on the instance.returns
{Object}: Returns the instance for chaining.Example
collection.use(function(views) {
// `views` is the instance, as is `this`
// optionally return a function to be passed to
// the `.use` method of each view created on the
// instance
return function(view) {
// do stuff to each `view`
};
});
Returns a new view, using the View
class currently defined on the instance.
Params
key
{String|Object}: View key or objectvalue
{Object}: If key is a string, value is the view object.returns
{Object}: returns the view
objectExample
var view = app.view('foo', {conetent: '...'});
// or
var view = app.view({path: 'foo', conetent: '...'});
Set a view on the collection. This is identical to addView except setView
does not emit an event for each view.
Params
key
{String|Object}: View key or objectvalue
{Object}: If key is a string, value is the view object.returns
{Object}: returns the view
instance.Example
collection.setView('foo', {content: 'bar'});
Adds event emitting and custom loading to setView.
Params
key
{String}value
{Object}Load multiple views onto the collection.
Params
views
{Object|Array}returns
{Object}: returns the collection
objectExample
collection.addViews({
'a.html': {content: '...'},
'b.html': {content: '...'},
'c.html': {content: '...'}
});
Load an array of views onto the collection.
Params
views
{Object|Array}returns
{Object}: returns the collection
objectExample
collection.addViews([
{path: 'a.html', content: '...'},
{path: 'b.html', content: '...'},
{path: 'c.html', content: '...'}
]);
Get a view from the collection.
Params
key
{String}: Key of the view to get.returns
{Object}Example
collection.getView('a.html');
Create an instance of View
. Optionally pass a default object to use.
Params
view
{Object}Example
var view = new View({
path: 'foo.html',
content: '...'
});
Run a plugin on the view
instance.
Params
fn
{Function}returns
{Object}Example
var view = new View({path: 'abc', contents: '...'})
.use(require('foo'))
.use(require('bar'))
.use(require('baz'))
Synchronously compile a view.
Params
locals
{Object}: Optionally pass locals to the engine.returns
{Object} View
: instance, for chaining.Example
var view = page.compile();
view.fn({title: 'A'});
view.fn({title: 'B'});
view.fn({title: 'C'});
Asynchronously render a view.
Params
locals
{Object}: Optionally pass locals to the engine.returns
{Object} View
: instance, for chaining.Example
view.render({title: 'Home'}, function(err, res) {
//=> view object with rendered `content`
});
Re-decorate View methods after calling vinyl's .clone()
method.
Params
options
{Object}returns
{Object} view
: Cloned instanceExample
view.clone({deep: true}); // false by default
Run a plugin on the list instance. Plugins are invoked immediately upon creating the list in the order in which they were defined.
Params
fn
{Function}: Plugin function. If the plugin returns a function it will be passed to the use
method of each view created on the instance.returns
{Object}: Returns the instance for chaining.Example
list.use(function(views) {
// `views` is the instance, as is `this`
// optionally return a function to be passed to
// the `.use` method of each view created on the
// instance
return function(view) {
// do stuff to each `view`
};
});
Returns a new item, using the Item
class currently defined on the instance.
Params
key
{String|Object}: Item key or objectvalue
{Object}: If key is a string, value is the item object.returns
{Object}: returns the item
objectExample
var item = app.item('foo', {conetent: '...'});
// or
var item = app.item({path: 'foo', conetent: '...'});
Add an item to the list. An item may be an instance of Item
, and if not the item is converted to an instance of Item
.
Params
items
{Object}: Object of viewsExample
var list = new List(...);
list.addItem('a.html', {path: 'a.html', contents: '...'});
Add an object of views
to the list.
Params
items
{Object}: Object of viewsExample
var list = new List(...);
list.addItems({
'a.html': {path: 'a.html', contents: '...'}
});
Add the items from another instance of List
.
Params
list
{Array}: Instance of List
fn
{Function}: Optional sync callback function that is called on each item.Example
var foo = new List(...);
var bar = new List(...);
bar.addList(foo);
Get a the index of a specific item from the list by key
.
Params
key
{String}returns
{Object}Example
list.getIndex('foo.html');
//=> 1
Get a specific item from the list by key
.
Params
key
{String}returns
{Object}Example
list.getItem('foo.html');
//=> '<View <foo.html>>'
Group all list items
using the given property, properties or compare functions. See group-array for the full range of available features and options.
returns
{Object}: Returns the grouped items.Example
var list = new List();
list.addItems(...);
var groups = list.groupBy('data.date', 'data.slug');
Sort all list items
using the given property, properties or compare functions. See array-sort for the full range of available features and options.
returns
{Object}: Returns a new List
instance with sorted items.Example
var list = new List();
list.addItems(...);
var result = list.sortBy('data.date');
//=> new sorted list
Paginate all items
in the list with the given options, See paginationator for the full range of available features and options.
returns
{Object}: Returns the paginated items.Example
var list = new List(items);
var pages = list.paginate({limit: 5});
Create an instance of Group
with the given options
.
Params
options
{Object}Example
var group = new Group({
'foo': {
items: [1,2,3]
}
});
Run a plugin on the group instance. Plugins are invoked immediately upon creating the group in the order in which they were defined.
Params
fn
{Function}: Plugin function. If the plugin returns a function it will be passed to the use
method of each view created on the instance.returns
{Object}: Returns the instance for chaining.Example
group.use(function(group) {
// `group` is the instance, as is `this`
});
Install dev dependencies:
$ npm i -d && npm test
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Jon Schlinkert
Copyright © 2015 Jon Schlinkert Released under the MIT license.
This file was generated by verb-cli on September 17, 2015.
FAQs
System for creating and managing template collections, and rendering templates with any node.js template engine. Can be used as the basis for creating a static site generator or blog framework.
The npm package templates receives a total of 26,482 weekly downloads. As such, templates popularity was classified as popular.
We found that templates demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.