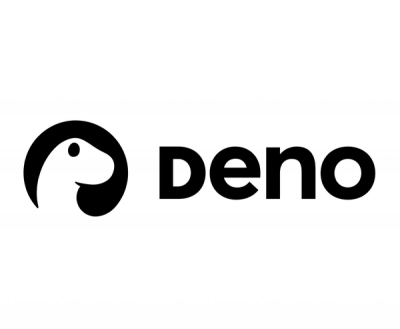
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
templates
Advanced tools
System for creating and managing template collections, and rendering templates with any node.js template engine. Can be used as the basis for creating a static site generator or blog framework.
System for creating and managing template collections, and rendering templates with any node.js template engine. Can be used as the basis for creating a static site generator or blog framework.
Install with npm
$ npm i templates --save
var templates = require('templates');
This function is the main export of the templates module. Initialize an instance of templates
to create your application.
Params
options
{Object}Example
var templates = require('templates');
var app = templates();
Create a view collection. View collections are stored on the app.views
object. For example, if you create a collection named posts
, the all posts
will be stored on app.views.posts
.
Params
name
{String}: The name of the collection. Plural or singular form.opts
{Object}: Collection optionsloaders
{String|Array|Function}: Loaders to use for adding views to the created collection.returns
{Object}: Returns the Template
instance for chaining.Example
app.create('posts');
app.posts({...}); // add an object of views
app.post('foo', {content: '...'}); // add a single view
// collection methods are chainable too
app.post('home.hbs', {content: 'foo <%= title %> bar'})
.render({title: 'Home'}, function(err, res) {
//=> 'foo Home bar'
});
Find a view by name
, optionally passing a collection
to limit the search. If no collection is passed all renderable
collections will be searched.
Params
name
{String}: The name/key of the view to findcolleciton
{String}: Optionally pass a collection name (e.g. pages)returns
{Object|undefined}: Returns the view if found, or undefined
if not.Example
var page = app.find('my-page.hbs');
// optionally pass a collection name as the second argument
var page = app.find('my-page.hbs', 'pages');
Get view key
from the specified collection
.
Params
collection
{String}: Collection name, e.g. pages
key
{String}: Template namefn
{Function}: Optionally pass a renameKey
functionreturns
{Object}Example
var view = app.getView('pages', 'a/b/c.hbs');
// optionally pass a `renameKey` function to modify the lookup
var view = app.getView('pages', 'a/b/c.hbs', function(fp) {
return path.basename(fp);
});
Get all views from a collection
using the collection's singular or plural name.
Params
name
{String}: The collection name, e.g. pages
or page
returns
{Object}Example
var pages = app.getViews('pages');
//=> { pages: {'home.hbs': { ... }}
var posts = app.getViews('posts');
//=> { posts: {'2015-10-10.md': { ... }}
Returns the first view from collection
with a key that matches the given glob pattern.
Params
collection
{String}: Collection name.pattern
{String}: glob patternoptions
{Object}: options to pass to micromatchreturns
{Object}Example
var pages = app.matchView('pages', 'home.*');
//=> {'home.hbs': { ... }, ...}
var posts = app.matchView('posts', '2010-*');
//=> {'2015-10-10.md': { ... }, ...}
Returns any views from the specified collection with keys that match the given glob pattern.
Params
collection
{String}: Collection name.pattern
{String}: glob patternoptions
{Object}: options to pass to micromatchreturns
{Object}Example
var pages = app.matchViews('pages', 'home.*');
//=> {'home.hbs': { ... }, ...}
var posts = app.matchViews('posts', '2010-*');
//=> {'2015-10-10.md': { ... }, ...}
Handle a middleware method
for view
.
Params
method
{String}: Name of the router method to handle. See router methodsview
{Object}: View objectcallback
{Function}: Callback functionreturns
{Object}Example
app.handle('customMethod', view, callback);
See the [route API documentation][route-api] for details on adding handlers and middleware to routes.
Params
path
{String}returns
{Object} Route
: for chainingExample
app.create('posts');
app.route(/blog/)
.all(function(view, next) {
// do something with view
next();
});
app.post('whatever', {path: 'blog/foo.bar', content: 'bar baz'});
Special route method that works just like the router.METHOD()
methods, except that it matches all verbs.
Params
path
{String}callback
{Function}returns
{Object} this
: for chainingExample
app.all(/\.hbs$/, function(view, next) {
// do stuff to view
next();
});
Add callback triggers to route parameters, where name
is the name of the parameter and fn
is the callback function.
Params
name
{String}fn
{Function}returns
{Object}: Returns the instance of Templates
for chaining.Example
app.param('title', function (view, next, title) {
//=> title === 'foo.js'
next();
});
app.onLoad('/blog/:title', function (view, next) {
//=> view.path === '/blog/foo.js'
next();
});
Params
exts
{String|Array}: String or array of file extensions.fn
{Function|Object}: or settings
settings
{Object}: Optionally pass engine options as the last argument.Example
app.engine('hbs', require('engine-handlebars'));
// using consolidate.js
var engine = require('consolidate');
app.engine('jade', engine.jade);
app.engine('swig', engine.swig);
// get a registered engine
var swig = app.engine('swig');
Compile content
with the given locals
.
Params
view
{Object|String}: View object.locals
{Object}isAsync
{Boolean}: Load async helpersreturns
{Object}: View object with fn
property with the compiled function.Example
var indexPage = app.page('some-index-page.hbs');
var view = app.compile(indexPage);
// view.fn => [function]
// you can call the compiled function more than once
// to render the view with different data
view.fn({title: 'Foo'});
view.fn({title: 'Bar'});
view.fn({title: 'Baz'});
Render a view with the given locals
and callback
.
Params
view
{Object|String}: Instance of View
locals
{Object}: Locals to pass to template engine.callback
{Function}Example
var blogPost = app.post.getView('2015-09-01-foo-bar');
app.render(blogPost, {title: 'Foo'}, function(err, view) {
// `view` is an object with a rendered `content` property
});
Install dev dependencies:
$ npm i -d && npm test
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Jon Schlinkert
Copyright © 2015 Jon Schlinkert Released under the MIT license.
This file was generated by verb-cli on September 09, 2015.
FAQs
System for creating and managing template collections, and rendering templates with any node.js template engine. Can be used as the basis for creating a static site generator or blog framework.
The npm package templates receives a total of 26,482 weekly downloads. As such, templates popularity was classified as popular.
We found that templates demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.