What is terminal-kit?
The terminal-kit npm package provides a comprehensive set of tools for creating rich terminal applications. It offers functionalities for handling terminal input and output, creating interactive user interfaces, and managing terminal capabilities.
What are terminal-kit's main functionalities?
Terminal Output
This feature allows you to output text to the terminal. The code sample demonstrates how to print 'Hello, world!' to the terminal using terminal-kit.
const term = require('terminal-kit').terminal;
term('Hello, world!\n');
Interactive Menus
This feature allows you to create interactive menus. The code sample shows how to create a single-column menu with three options and handle the user's selection.
const term = require('terminal-kit').terminal;
term.singleColumnMenu(['Option 1', 'Option 2', 'Option 3'], function(error, response) {
term('
').eraseLineAfter.green('You selected: %s
', response.selectedText);
});
Text Input
This feature allows you to capture text input from the user. The code sample demonstrates how to prompt the user to enter their name and then display it.
const term = require('terminal-kit').terminal;
term('Enter your name: ');
term.inputField(function(error, input) {
term.green('
Your name is: %s
', input);
});
Progress Bars
This feature allows you to create and manage progress bars. The code sample shows how to create a progress bar that updates every 100 milliseconds until it reaches 100%.
const term = require('terminal-kit').terminal;
const ProgressBar = require('terminal-kit').ProgressBar;
const progressBar = new ProgressBar({
width: 80,
title: 'Progress',
eta: true,
percent: true
});
let progress = 0;
const interval = setInterval(() => {
progress += 0.01;
progressBar.update(progress);
if (progress >= 1) {
clearInterval(interval);
term.green('
Done!
');
}
}, 100);
Other packages similar to terminal-kit
blessed
Blessed is a high-level terminal interface library for node.js. It provides a wide range of widgets and supports complex layouts, making it suitable for creating advanced terminal applications. Compared to terminal-kit, blessed offers more built-in widgets and a more extensive API for layout management.
ink
Ink is a React-like library for building command-line interfaces using React components. It allows you to use the familiar React paradigm to create terminal applications. Compared to terminal-kit, ink leverages the React ecosystem, making it a good choice for developers who are already comfortable with React.
vorpal
Vorpal is a framework for building interactive CLI applications. It provides a command-line interface with built-in support for commands, arguments, and options. Compared to terminal-kit, vorpal focuses more on creating command-line tools rather than rich terminal UIs.


Terminal Kit
A full-blown terminal lib featuring: 256 colors, styles, keys & mouse handling, input field, progress bars,
screen buffer (including 32-bit composition and image loading), text buffer, and many more...
Whether you just need colors & styles, build a simple interactive command line tool or a complexe terminal application:
this is the absolute terminal lib for Node.js!
It does NOT depend on ncurses.
Some tutorials are available at blog.soulserv.net/tag/terminal.
Screenshot, PleaZe!
This is a fraction of what Terminal-Kit can do, with only few lines of code.
Click any image to see the documentation related to the feature!

← Word-wrapping
← Table with automatic column computing, cell fitting and word-wrapping




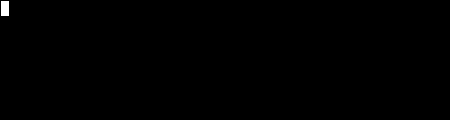
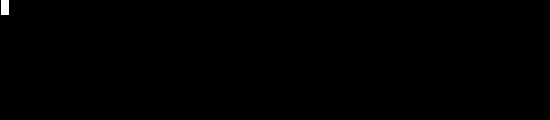




← Surfaces and Sprites
← Load and draw an image inside the terminal
Key features
New: Document model for building rich app GUI
New: Spinner
New: Table with automatic column computing, cell fitting and word-wrapping
New: Promises can be used instead of callback everywhere
New: Word-wrapping along full terminal width or a pre-defined column-width
New: ScreenBuffer HD 32-bit (RGBA) surfaces with composition and image loading
Quick examples
var term = require( 'terminal-kit' ).terminal ;
term( 'Hello world!\n' ) ;
term.red( 'red' ) ;
term.bold( 'bold' ) ;
term.bold.underline.red( 'mixed' ) ;
term.green( "My name is %s, I'm %d.\n" , 'Jack' , 32 ) ;
term( "My name is " ).red( "Jack" )( " and I'm " ).green( "32\n" ) ;
term( "My name is ^rJack^ and I'm ^g32\n" ) ;
term( 'The terminal size is %dx%d' , term.width , term.height ) ;
term.moveTo( 1 , 1 ) ;
term.moveTo( 1 , 1 , 'Upper-left corner' ) ;
term.moveTo( 1 , 1 , "My name is %s, I'm %d.\n" , 'Jack' , 32 ) ;
term.moveTo.cyan( 1 , 1 , "My name is %s, I'm %d.\n" , 'Jack' , 32 ) ;
term.magenta( "Enter your name: " ) ;
term.inputField(
function( error , input ) {
term.green( "\nYour name is '%s'\n" , input ) ;
}
) ;
License: MIT