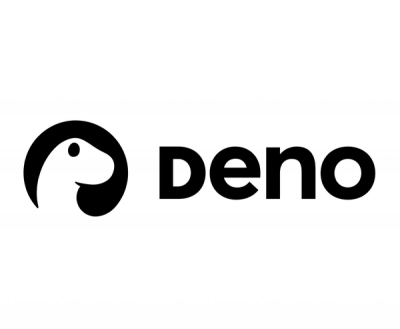
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Tesla.js is a boilerplate node.js framework, with some basic MVC features. It's still a work in progress and will be at least few more weeks before it's feature complete.
#####Prerequisites
#####Included Packages
#####Installation
#####Quick Start
#####Configuration
#####Models, Controllers & Views, Oh My!
#####Troubleshooting
$ npm install -g tesla-cli
Once Tesla is installed, simply run the following command anytime you want to create a new app:
$ tesla app-name
This will create a new app with the name "app-name". Next, switch into your new apps directory:
$ cd app-name
Than install dependencies:
$ npm install
And finally start the server:
$ grunt
Once the server has started, simply point your browser to:
http://localhost:3000
Usage: tesla [options]
Options:
-V, --version output the version number
-e, --ejs add ejs engine support (defaults to jade)
-J, --jshtml add jshtml engine support (defaults to jade)
-H, --hogan add hogan.js engine support (defaults to jade)
-c, --css add stylesheet support (less|sass|stylus) (defaults to plain css)
-f, --force force on non-empty directory
For example, if you want to generate an application called "foobar" with Jade & Stylus support you would simply execute:
$ tesla --css stylus foobar
Or to generate an application with EJS & SASS support:
$ tesla --css sass --ejs foobar
The second option is to simply clone the repo and use it as a barebones boilerplate to start your next project.
$ git clone git@github.com:teslajs/tesla.js.git
This is the most light-weight option and gives you the most control over how you set your project up.
However, this step requires a few additional steps to configure correctly:
Open app/config/config.js and set the "app.site.name" to your app name. Open package.json and set the "name" to your app name.
Open app/config/config.js and set your engines:
app.config = {
engines : {
html: "jade", // options: [jade|ejs|haml|hjs|jshtml]
css: "stylus", // options: [stylus|sass|less]
},
}
Install NPM modules
// Install your selected HTML engine
$ npm install jade // for Jade
$ npm install ejs // for EJS
$ npm install jshtml // for JSHTML
$ npm install express-hogan // for HJS (Hogan)
// Install your selected CSS engine
$ npm install less // for LESS
$ npm install sass // for SASS
$ npm install stylus // for STYLUS
Next, go into the app folder and rename the view folder you want to use to "views". For example if you're using Jade, rename "views.jade" to "views". Delete the rest.
Finally, do the same for the css directory. Go into the public folder and rename the css directory you want to use. To use Stylus, rename "stylus.css" to "css" and delete the rest of the folders. If you don't want to use a css processor, keep the current "css" folder and delete the rest.
Now that everything is configured, cd into your app directory and run the following command:
$ npm install
We recommend using Grunt to start the server:
$ grunt
This will watch for changes to any of your files and automatically restart then server when necesary. If you choose not not using Grunt, you can run the app like so:
$ node server
With this method you have to manually stop and start the server any time you make changes.
Once the server has started, simply point your browser to:
http://localhost:3000
All configuration is specified in the config folder, particularly the config.js file and the env files. Here you will need to specify your application name, database name, and any other settings you would like to customize.
Most default settings can be set & updated here:
app.site = {
name : "Tesla.js", // the name of you app
}
app.config = {
port : 3000, // port to run the server on
prettify : {
html : true, // whether to pretify html
},
engines : {
html: "jade", // specify view engine - options: jade, ejs, haml, hjs (hogan)
css: "stylus", // specify css processor - options: stylus, sass, less
},
root : rootPath,
db : {
url : "mongodb://localhost/db-name" // url to database
},
jsonp : true, // allow jsonp requests
secret : 'MYAPPSECRET',
protocol : 'http://',
autoLoad : false, // whether to autoload controllers & models
}
// some default meta setting for head
app.site.meta = {
description : '',
keywords : '',
viewport : 'width=device-width, user-scalable=yes, initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0',
encoding : "utf-8"
}
To run with a different environment, just specify NODE_ENV as you call grunt:
$ NODE_ENV=test grunt
If you are using node instead of grunt, it is very similar:
$ NODE_ENV=test node server
// global settings
app.site.domain = "localhost"; // domain the site is running on
app.site.environment = "Development"; // name of environment
app.site.url = app.config.protocol + app.site.domain + ':' + app.config.port + '/'; // base url
// directories location to use for dynamic file linking
app.site.dir = {
css : app.site.url + "css/",
img : app.site.url + "img/",
lib : app.site.url + "lib/",
js : app.site.url + "js/"
};
NOTE: Running Node.js applications in the production environment enables caching, which is disabled by default in all other environments.
Tesla comes with an automatic routing system which saves you the trouble of manually creating routes for your site. The routing is based on the following URI structure:
http://localhost:3000/controller/action
As an example, http://localhost:3000/home would load the following controller: app/controllers/home.js
Similarly, http://localhost:3000/foo/bar would load this controller: app/controllers/foo/bar.js
The exeption to this rule is if you set "autoLoad: true" in config/config.js. In this case you only need to create a model and a view, as Tesla will attempt to automatically load the model and the view using the app/controllers/auto.js controller.
With autoload, going to http://localhost:3333/hello/world will attempt to load the following files:
*Controller: app/controllers/auto.js *Model: app/models/hello.js *View: app/views/hello/world.jade
Autoload assumes a model with the name of the controller, and will try to find a record whose "name" field matches the action. Using http://localhost:3000/articles/super-awesome-fun-time as an example, autoload load use a model called "articles" and try to find a record with the name "super-awesome-fun-time":
articles.findOne({name: "super-awesome-fun-time"})
With autoloading, any data returned from the model will be sent to the view via the "data" variable. If no data is returned we assume the page does not exist and will throw a 404 error. Also worth noting, autoload expects to find a model to provide data to the view. If you forget to create a model with the correct name, you will get a 404 error when loading the page. If you don't want or need a model to provide data to your controller, or want to use a different URI structure, don't use autoload.
If you are not using autoload, you will need to create your own controllers, which couldn't be easier! For example, if you want to create the page http://localhost:3000/help, simply create a controller with the same name: app/controllers/help.
Then, add the following code:
exports.render = function(app) {
app.res.render('help', {
site: app.site
});
};
The above code is pretty simple, it simply loads the view "app/views/help.jade" and passes it a "site" variable.
For a slightly for complex example, lets say you want to create a blog with the following uri structure: http://localhost:3000/article/read?id=12345
Let's assume also you also want use an "article" model to load an article with the id "12345". Create the file "app/controllers/article/read.js" with the following code:
var mongoose = require('mongoose'),
Articles = mongoose.model( 'Article' ),
exports.render = function(app) {
var id = req.query('id')
Articles.findOne({_id: ud}).exec(function(err, article) {
// IF WE GET AN ERROR
if (err) {
app.res.render('error', {
status: 500
});
// IF NO DATA WAS RETURNED, THROW A 404
} else if ( article === null) {
app.res.status(404).render('404', {
pageTitle : app.site.name + ' - Not Found',
url: app.req.originalUrl,
error: 'Not found',
site: app.site
});
// IF NO PROBLEMS, RENDER PAGE
} else {
// LOAD THE ARTICLE/READ VIEW & PASS DATA FROM THE MODEL
app.res.render('article/read', {
article : article,
site: app.site
});
}
}
});
};
Views can use Jade (default), Haml, Handlebars or EJS. See the appropriate documentation for you chosen templating language for more info on how to use it.
For models, Tesla uses Mongoose to connect to a MongoDB server. Documentation on working with Mongoos can be found here: http://mongoosejs.com/docs/guide.html
During install some of you may encounter some issues, most of this issues can be solved by one of the following tips. If you went through all this and still can't solve the issue, feel free to contact me(Amos), via the repository issue tracker or the links provided below.
Sometimes you may find there is a weird error during install like npm's Error: ENOENT, usually updating those tools to the latest version solves the issue.
Updating NPM:
$ npm update -g npm
Updating Grunt:
$ npm update -g grunt-cli
Updating Bower:
$ npm update -g bower
NPM and Bower has a caching system for holding packages that you already installed. We found that often cleaning the cache solves some troubles this system creates.
NPM Clean Cache:
$ npm cache clean
Bower Clean Cache:
$ bower cache clean
Before you start make sure you have heroku toolbelt installed and an accessible mongo db instance - you can try mongohq which have an easy setup )
git init
git add .
git commit -m "initial version"
heroku apps:create
git push heroku master
Inspired by the MEAN Stack by Amos Haviv and Express by TJ Holowaychuk
FAQs
A modern tesla api client for the tesla api.
The npm package tesla receives a total of 5 weekly downloads. As such, tesla popularity was classified as not popular.
We found that tesla demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.