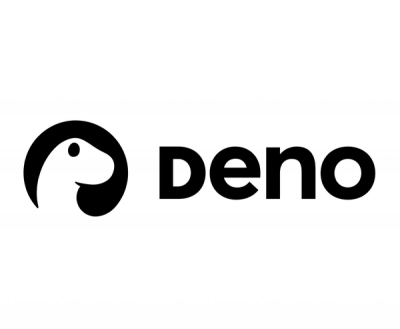
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The 'three' npm package is a JavaScript library that provides a wide array of functionalities for creating and displaying 3D graphics in web browsers. It uses WebGL under the hood and provides an easy-to-use API to create 3D scenes, render them, and add various elements like shapes, materials, lights, cameras, and more.
Creating a Scene
This code sample demonstrates how to create a new 3D scene using Three.js. A scene is a container that holds all your objects, cameras, and lights.
const scene = new THREE.Scene();
Adding a Mesh
This code sample shows how to create a simple cube mesh with a green color and add it to the scene. A mesh consists of a geometry and a material.
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
Setting up a Camera
This code sets up a perspective camera with a certain field of view, aspect ratio, and near and far clipping planes. The camera is positioned 5 units away from the origin along the z-axis.
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
Rendering the Scene
This code sample initializes a WebGL renderer, sets its size, and appends its DOM element to the body of the document. It also defines an animate function that continuously renders the scene using the camera.
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
animate();
Adding Lights
This code sample adds a point light to the scene, which emits light in all directions. The light's color, intensity, and distance are set, and its position is placed at coordinates (10, 10, 10).
const light = new THREE.PointLight(0xffffff, 1, 100);
light.position.set(10, 10, 10);
scene.add(light);
Babylon.js is a powerful, beautiful, simple, and open game and rendering engine packed into a friendly JavaScript framework. Similar to Three.js, it allows developers to create 3D content for the web. Babylon.js has a different API and additional features like a physics engine and advanced particle systems.
PlayCanvas is an open-source 3D engine/IDE that is specifically designed for the web. It offers real-time collaboration features and is more focused on game development. It provides an editor for building 3D applications and has a different approach to workflow compared to Three.js.
A-Frame is a web framework for building virtual reality (VR) experiences. It is built on top of Three.js and abstracts away the complexity of Three.js with a declarative HTML-like syntax. A-Frame is designed for creating VR experiences and is more accessible for web developers who are familiar with HTML and JavaScript.
The aim of the project is to create a lightweight 3D library with a very low level of complexity — in other words, for dummies. The library provides <canvas>, <svg>, CSS3D and WebGL renderers.
Examples — Documentation — Migrating — Help
Download the minified library and include it in your html. Alternatively see how to build the library yourself.
<script src="js/three.min.js"></script>
This code creates a scene, then creates a camera, adds the camera and cube to the scene, creates a <canvas> renderer and adds its viewport in the document.body element.
<script>
var camera, scene, renderer;
var geometry, material, mesh;
init();
animate();
function init() {
camera = new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 1, 10000 );
camera.position.z = 1000;
scene = new THREE.Scene();
geometry = new THREE.CubeGeometry( 200, 200, 200 );
material = new THREE.MeshBasicMaterial( { color: 0xff0000, wireframe: true } );
mesh = new THREE.Mesh( geometry, material );
scene.add( mesh );
renderer = new THREE.CanvasRenderer();
renderer.setSize( window.innerWidth, window.innerHeight );
document.body.appendChild( renderer.domElement );
}
function animate() {
// note: three.js includes requestAnimationFrame shim
requestAnimationFrame( animate );
mesh.rotation.x += 0.01;
mesh.rotation.y += 0.02;
renderer.render( scene, camera );
}
</script>
2013 02 15 - r56 (407,609 KB, gzip: 96,870 KB)
LineDashedMaterial
support to CanvasRenderer
. (sole)Matrix3.getNormalMatrix()
and Vector3.transformDirection()
. (WestLangley)Matrix4
's getPosition()
and getColumn*()
. (mrdoob and bhouston)Vector*.toArray()
. (mrdoob)FlyControls
. (WestLangley)OBJLoader
and OBJMTLLoader
. (edwardsp and Fktrcfylh).reflect()
, projectOnVector()
and projectOnPlane()
to Vector3
. (bhouston)OculusRiftEffect
. (troffmo5)setHSL()
and getHSL()
to Color
. (pksunkara and mrdoob)STLLoader
. (aleeper)setHSV()
, getHSV()
from Color
. (mrdoob)ColorUtils.adjustHSV()
with Color
's .offsetHSL()
. (mrdoob)Line3
. (bhouston)linewidth
support to BufferGeometry
lines. (arodic)Box3
/Line3
/Plane
/Ray
/Sphere
's .transform()
to applyMatrix4()
. (bhouston)smoothstep
and smootherstep
to Math
. (bhouston).reset()
to TrackballControls
. (WestLangley)ColladaLoader
. (jihoonl)2013 01 15 - r55 (406,462 KB, gzip: 96,542 KB)
set()
, identity()
, copy()
, multiplyScalar()
, determinant()
, getInverse()
and clone()
to Matrix3
. (bhouston)WebGLRenderer
under the WebGLRenderer2
name (may become WebGLRenderer
next release). (gero3)Matrix4
's determinant()
. (bhouston)negate()
to Plane
. (bhouston)containsPoint()
and intersectsObject()
to Frustum
. (bhouston)MeshNormalMaterial
rendering in CanvasRenderer
and SVGRenderer
. (mrdoob)Matrix*/Quaternion
's multiply*
to Vector*
's .apply*
. (mrdoob)MeshNormalMaterial
with SmoothShading
support to CanvasRenderer
. (mrdoob)Edit / Clone
to the editor. (mrdoob)ArrowHelper
. (bhouston and WestLangley)Geometry
's mergeVertices()
. (bhouston)LatheGeometry
. (bhouston and WestLangley)insertPass
to EffectComposer
. (alteredq)BufferGeometry
support to Line
. (arodic)intersectsSphere
to Sphere
. (Fox32)WebGLDeferredRenderer
. (alteredq)updateMorphTargets
a public method of Mesh
. (jonobr1)add()
, addColors()
, addScalar()
, multiply()
and multiplyScalar()
to Color
. (mrdoob)Vector*/Matrix*/Quaternion
's add()/sub()/cross()...
to addVectors()/subVectors()/crossVectors()...
. (mrdoob)Vector*/Matrix*/Quaternion
's addSelf()/subSelf()/crossSelf()...
to add()/sub()/cross()...
. (mrdoob)GeometryUtils
's explode()
and tessellate()
to ExplodeModifier
and TessellateModifier
. (mrdoob)BinaryLoader
out of the lib to examples folder. (mrdoob)OBJExporter
. (mrdoob)TrackballControls
. (ericnoble and mrdoob)OrbitControls
.. (mrdoob)SoftwareRenderer
and started adding material handling. (rygorous and mrdoob)CanvasRenderer/SVGRenderer
x/y clipping to Projector
. (mrdoob)applyEuler()
and applyAxisAngle()
in Vector3
. (WestLangley)FirstPersonControls
. (RommelVR)2012 12 25 - r54 (411,352 KB, gzip: 98,639 KB)
Sprite
. (alteredq)WebGLDeferredRenderer
with all sorts of goodness. (mpanknin and alteredq)Sprite
to use SpriteMaterial
instead of custom object. (alteredq)sourcemap
option to python and nodejs build systems. (zz85 and gero3)BufferGeometry
. (benaadams)Texture
and DataTexture
. (benaadams and alteredq)Color
. (mrdoob)SceneLoader
. (RommelVR and alteredq)Plane
class and implemented in Frustum
. (bhouston)Box3
, Sphere
math classes and implemented in Geometry
. (bhouston)UV
with Vector2
. (mrdoob)Ray
to Raycaster
and added new Ray
math class. (bhouston)CSS3DRenderer
. (mrdoob, benaadams and zz85).dispose()
to Geometry
, BufferGeometry
, Texture
, Material
and WebGLRenderTarget
for deallocating from GPU. (mrdoob and alteredq)SubdivisionModifier
out of the build to examples/js/modifiers
. (mrdoob)ColladaLoader
. (dgossow).setMaterialIndex()
to GeometryUtils
. (gero3)devicePixelRatio
support to CanvasRenderer
and WebGLRenderer
. (mrdoob)2012 11 15 - r53 (392,799 KB, gzip: 96,044 KB)
Sprite
no longer gets its size from the texture. (alteredq and mrdoob)CSS3DRenderer
. (mrdoob and alteredq)Ribbon
. (alteredq)Object3D
's .clone()
is now recursive. (mrdoob)Sprite
. (alteredq)Object3D
's .lookAt()
now working when using quaternions. (motin)TrackballControls
. (mrdoob)WebGLRenderer
is now stable, regardless of browser implementation. (alteredq)MeshPhongMaterial
's perPixel
is not true
by default. (alteredq)LineDashedMaterial
. (alteredq).setContextStyle
to Color
. (greyscales)KaleidoShader
, MirrorShader
and RGBShiftShader
. (felixturner)Geometry
. (alteredq)MeshFaceMaterial
. (gero3, alteredq and mrdoob)materials
and sides
from CubeGeometry
. (mrdoob)GeometryUtils
's .clone()
to Geometry
. (mrdoob)2012 10 15 - r52 (379,442 KB, gzip: 94,126 KB)
SubdivisionModifier
. (zz85)defines
parameter for adding preprocessor definitions to ShaderMaterial
. (alteredq)ShaderExtras
into single files (BasicShader
, BlendShader
, ConvolutionShader
, ... ). (mrdoob)HueSaturationShader
and BrightnessContrastShader
. (tapio)ColladaLoader
not loading sometimes. (tapio)material.vertexColors = THREE.FaceColor
support to CanvasRenderer
and SVGRenderer
. (mrdoob)Object3D.defaultEulerOrder
. (mrdoob)SceneUtils.traverseHierarchy
and SceneUtils.showHierarchy
with object.traverse
. (mrdoob)PointerLockControls
. (mrdoob)SceneUtils.cloneObject
into *.clone()
. (mrdoob)AxisHelper
. (mrdoob)GeometryExporter
. (mrdoob)OrbitControls
. (WestLangley)GeometryLibrary
, MaterialLibrary
, TextureLibrary
and ObjectLibrary
(bear in mind that you can't rely on the GC now. Call *.deallocate()
for removing). (mrdoob)*Controls
out of the lib. (mrdoob)Manual
section to the documentation pages. (oal).angleTo()
to Vector3
. (Wilt)2012 09 15 - r51 (405,491 KB, gzip: 99,389 KB)
STLLoader
. (aleeper and mrdoob)Ray
(2x faster). (gero3).getDescendants
method to Object3D
. (gero3 and mrdoob)SkinnedMesh
can now work with MorphAnimMesh
. (apendua)CameraHelper
. Now it matches the camera independently of where it's in the scene graph. (mrdoob)ShaderMaterial
. (alteredq)HemisphereLight
. (alteredq)WebGLRenderer
handling of flip sided materials. (WestLangley and alteredq)MeshPhongMaterial
. (crobi and alteredq)BufferGeometry
for ParticleSystems
. (alteredq)WebGLRenderer
. (alteredq)CanvasRenderer
and SVGRenderer
. (mrdoob)Loader
and SceneLoader
. (alteredq)TrackballControls
. (jherrm)MTLLoader
and OBJMTLLoader
. (angelxuanchang)UTF8Loader
to latest version. (angelxuanchang and alteredq)SceneLoader
. (alteredq)object.renderDepth
in Projector
. (mrdoob)SceneLoader
. (alteredq)CSS3DRenderer
. (mrdoob)ShapeGeometry
. (jonobr1)Vector3
's .setEulerFromRotationMatrix
method.(WestLangley)2012 08 15 - r50 (391,250 KB, gzip: 96,143 KB)
DOMRenderer
and SVGRenderer
out of common build. (mrdoob).deallocateMaterial
method to WebGLRenderer
. (alteredq).worldToLocal
and .localToWorld
methods to Object3D
. (zz85 and WestLangley)ConvexGeometry
. (qiao)ImmediateRenderObjects
. (alteredq)LoadingMonitor
and EventTarget
in loaders. (mrdoob)Path.ellipse
. (linzhp)near
and far
properties to Ray
. (niklassa)OrbitControls
. (qiao, mrdoob and alteredq)VTKLoader
parsing more robust. (mrdoob)recursive
flag to Ray
. (mrdoob)BufferGeometry
. (alteredq)WebGLRenderer
. (alteredq)SkinnedMesh
. (n3tfr34k and alteredq)OBJLoader
parsing more robust. (Dahie)WebGLRenderer
. (alteredq)ParticleBasicMaterial
without map
in CanvasRenderer
. (mrdoob)SceneLoader
now supports nested scene graphs and per object custom properties. (skfcz)Camera
doesn't need to be added to the scene anymore. (mrdoob)Object3D
's flipSided
and doubleSided
properties are now Material
's side
property. (alteredq and mrdoob).clone
method to *Material
. (gero3, mrdoob and alteredq)CircleGeometry
. (hughes)bumpMap
to MeshPhongMaterial
. (alteredq)specularMap
to MeshBasicMaterial
, MeshLambertMaterial
and MeshPhongMaterial
. (alteredq)2012 04 22 - r49 (364,242 KB, gzip: 89,057 KB)
ColladaLoader
improvements. (ekitson, AddictArts and pblasco)MorphBlendMesh
. (alteredq)emissive
component to WebGL Materials. (alteredq)DepthPassPlugin
. (alteredq)Path
. (asutherland)Curve
. (zz85)ArrowHelper
. (zz85 and WestLangley)WebGLRenderer
to use world positions. (alteredq)WebGLRenderer
. (WestLangley)Projector
to use world positions. (mrdoob)TubeGeometry
. (zz85 and WestLangley)needsUpdate
flag to Material
. (alteredq)GeometryUtils.triangulateQuads
. (alteredq)GeometryUtils.tessellate
. (alteredq)PlaneGeometry
from XY to XZ. (mrdoob)WebGLRenderer
back to highp
shader precision. (mrdoob)deallocateRenderTarget
to `WebGLRenderer. (kovleouf)DOMRenderer
. (ajorkowski)CameraHelper
. (zz85)ExtrudeGeometry
. (zz85)MarchingCubes
moved out of the lib into /examples/js
folder. (alteredq)ImmediateRenderObject
. (alteredq)__dirty*
to *NeedUpdate
. (valette and mrdoob)CustomBlending
to Material
and premultiplyAlpha
to Texture
. (alteredq)CubeCamera
. (alteredq and mrdoob)CanvasRenderer.setClearColor()
and .setClearColorHex()
now sets opacity
to 1 when null. (mrdoob)SubdivisionModifier
. (zz85)Matrix4
's setTranslation
, setRotationX
, setRotationY
, setRotationZ
, setRotationAxis
and setScale
to makeTranslation
, makeRotationX
, makeRotationY
, makeRotationZ
, makeRotationAxis
and makeScale
. (mrdoob)Matrix4
static methods makeFrustum
, makePerspective
, makeOrtho
to non-static methods. (mrdoob)Matrix4
to Matrix3
inversion. (alteredq)GodRays
postprocessing. (huwb)LinePieces
support to Projector
. (mrdoob)GeometryUtils.tessellate
. (alteredq)Matrix4
, Matrix3
and Frustum
. (gero3)LatheGeometry
. (zz85)Vertex
. Use Vector3
instead. (mrdoob)Spotlight
s. (alteredq)ParametricGeometry
. (zz85)OBJLoader
in /examples/js/loaders
folder. (mrdoob)ColladaLoader
and UTF8Loader
to /examples/js/loaders
folder. (mrdoob)VTKLoader
to /examples/js/loaders
folder. (valette and mrdoob)visible
property to Material
. (mrdoob)CanvasRenderer
. (mrdoob)CylinderGeometry
. (qiao)WebGLRenderer
. (mrdoob)AnaglyphWebGLRenderer
and co. to AnaglyphEffect
& co. and moved to /examples/js/effects
. (mrdoob)AsciiEffect
. (zz85)2012 03 04 - r48 (393,626 KB, gzip: 99,395 KB)
ColladaLoader
. (jbaicoianu)ColladaLoader
improvements. (mrdoob, AddictArts, kduong)IcosahedronGeometry
and OctahedronGeometry
with timothypratley's PolyhedronGeometry
code which also brings TetrahedronGeometry
. (mrdoob)LOD
should now behave as expected from anywhere in the scene graph. (mrdoob)THREE.REVISION
. (mrdoob)Geometry
's .computeBoundingBox
and .computeBoundingSphere
. (alteredq)SceneLoader
. (alteredq)Material
's default ambient color to 0xffffff. (alteredq)MorphTarget
. (alteredq).setFrameRange
and .setAnimationLabel
to MorphAnimMesh
. (alteredq)MorphAnimMesh
. (alteredq)MorphAnimMesh
to be able to play animations backwards. (alteredq).generateDataTexture
to ImageUtils
. (alteredq).intersectScene()
from Ray
. (mrdoob).triangulateQuads
to GeometryUtils
. (alteredq)Projector
and WebGLRenderer
now handles doubleSided lighting properly. (mrdoob and alteredq)MorphAnimMesh
playback bug where the last frame didn't display. (alteredq)TrackballControls
implements EventTarget
. (mrdoob).clone
to Vertex
, Face3
and Face4
. (alteredq).explode
and .tessellate
to GeometryUtils
. (alteredq).lerpSelf
to Vector2
, Vector3
and UV
. (alteredq)DOMRenderer
by using single-materials. (ajorkowski ).setPrecision
to Ray
. (mrdoob)NoBlending
to Material
and WebGLRenderer
. (kovleouf).applyMatrix
to Object3D
. (mrdoob and alteredq).attach
and .detach
to SceneUtils
to retain position in space. (alteredq).sign
to Math
. (alteredq)Mesh
, Line
and ParticleSystem
. (mrdoob)2012 01 14 - r47 (378,169 KB, gzip: 96,015 KB)
WebGLRenderer
plugin. (alteredq)Matrix4
's transpose()
and getInverse()
. (ekitson)Frustum
class. (alteredq)ColladaLoader
improvements. (ekitson, jterrace, mrdoob and alteredq)WebGLRenderer
. (alteredq)/doc
(using sphinx). (jterrace)mediump
. (mrdoob)BufferGeometry
for direct rendering from typed arrays. (alteredq)Texture
class with format
and type
parameters. (alteredq)WebGLRenderer
's Shadow Map code. (alteredq)xhr.overrideMimeType
before using it (fixing IE support). (mrdoob and alteredq)WebGLRenderer
shaders. (alteredq)generateMipmaps
property to Texture
and RenderTarget
. (alteredq)Frustum
properly handling children with scaled parents. (avinoamr)Ray
when dealing with big polygons. (WestLangley)WebGLRenderer
bug where depth buffer was not cleared. (ekitson)CameraHelper
objects for visualising both Perspective and Orthographic cameras. (alteredq)Path
. (zz85)DirectionalLight
s. (alteredq)Gyroscope
object. (alteredq)alpha
and premultipliedAlpha
parameters to WebGLRenderer
. (mrdoob)Ray
properly handling children with scaled parents. (mrdoob)Axes
object to AxisHelper
. (mrdoob)2011 11 17 - r46 (343.383 KB, gzip: 87.468 KB)
Ray
now checks also object children. (mrdoob)*Loader.load( parameters )
to *Loader( url, callback, texturePath )
. (mrdoob and alteredq)CanvasRenderer
's SphericalReflectionMapping
rendering. (mrdoob)SubdivisionModifier
. (zz85)*Controls
to use externally supplied time delta. (alteredq)CombinedCamera
. (zz85)ColladaLoader
doesn't create extra Object3D
. (mrdoob)Ray
not considering edges. (mrdoob)WebGLRenderer
. (alteredq)Ray
optimisations. (mrdoob and alteredq)CubeGeometry
, PlaneGeometry
, IcosahedronGeometry
and SphereGeometry
. (mrdoob)Curve
. (zz85)Collisions
code and focusing on Ray
. (mrdoob)cloneObject()
method to SceneUtils
. (alteredq)2011 10 06 - r45 (340.863 KB, gzip: 86.568 KB)
Object/Scene.add*()
and Object/Scene.remove*()
are now Object/Scene.add()
and Object/Scene.remove()
. (mrdoob)LOD.add()
is now LOD.addLevel()
. (mrdoob)CylinderGeometry
. (mrdoob).depthWrite
and .fog
to Material
. (alteredq).applyMatrix
to Geometry
. (mrdoob)/examples/js/postprocessing
. (alteredq).center()
to GeometryUtils
. (alteredq)MeshShaderMaterial
to ShaderMaterial
. (alteredq)CanvasRenderer
ignoring color of SmoothShading
ed MeshLambertMaterial
. (mrdoob)renderer.data
to renderer.info
. (mrdoob)Loader
's extractUrlbase()
incorrect output for short urls. (rectalogic and alteredq).color
and .visible
support to Sprite
. (alteredq)Face4
, Vertex Colors and Maya support to ColladaLoader
. (mrdoob)WebGLRenderer
performance improvements. (alteredq)WebGLRenderer
. (NINE78 and alteredq)CubeCamera
for rendering cubemaps. (alteredq)GeometryUtils
's .mergeVertices()
performance. (zz85)Camera
's .target
. (mrdoob)WebGLRenderer
's .clear()
is now .clear( color, depth, stencil )
. (mrdoob).autoClearColor
, .autoClearDepth
and .autoClearStencil
to WebGLRenderer
. (mrdoob and alteredq)OctahedronGeometry
. (clockworkgeek)Camera
into PerspectiveCamera
and OrthographicCamera
. (mrdoob and alteredq)*Controls
. (alteredq and mrdoob)SubdivisionModifier
. (zz85)Projector
's unprojectVector()
now also works with OrthographicCamera
. (jsermeno).setLens()
method to PerspectiveCamera
. (zz85)Texture
's .offset
and .repeat
and reflections support to Normal Map shader. (alteredq)2011 09 04 - r44 (330.356 KB, gzip: 84.039 KB)
ColladaLoader
. (timknip2)ExtrudeGeometry
. (zz85)CylinderGeometry
normals. (alteredq)WebGLRenderer.setTexture
(rectalogic)TorusGeometry
normals. (mrdoob)Ray
behind-ray intersects. (mrdoob)OrthoCamera
. (alteredq).deallocateObject()
and .deallocateTexture()
methods to WebGLRenderer
. (mrdoob).frustumCulled
property to Object3D
. (alteredq and mrdoob)2011 08 14 - r43 (298.199 KB, gzip: 74.805 KB)
ImageUtils
. (mrdoob)TextGeometry
and added Shape
, Curve
, Path
, ExtrudeGeometry
, TextPath
. (zz85 and alteredq)ParticleSystems
. (alteredq)CanvasRenderer.setClearColor
. (mrdoob, StephenHopkins and sebleedelisle)WebGLRenderer
. (alteredq)WebGLRenderer
. (alteredq)Spotlight
light type. (alteredq)DataTexture
. (alteredq)WebGLRenderer
opaque pass now renders from front to back. (alteredq)Color
. (mrdoob)preserveDrawingBuffer
option to WebGLRenderer
. (jeromeetienne)UTF8Loader
for loading the new, uber compressed, UTF8 format. (alteredq)CanvasRenderer
now supports RepeatWrapping
, texture.offset
and texture.repeat
. (mrdoob)2011 07 06 - r42 (277.852 KB, gzip: 69.469 KB)
AnaglypWebGLRenderer
and CrosseyedWebGLRenderer
. (mrdoob, alteredq and marklundin)TextGeometry
. (zz85 and alteredq)setViewOffset
method to Camera
. (greggman)*Geometry
. (mrdoob)Matrix4.multiply()
. (thanks lukem1)Matrix4
. (rectalogic)QuakeCamera
to FirstPersonCamera
. (chriskillpack)Collision
now supports Object3D.flipSided
and Object3D.doubleSided
. (NINE78)SceneUtils
methods. (mrdoob)Sound
object and SoundRenderer
. (mrdoob)2011 05 31 - r41/ROME (265.317 KB, gzip: 64.849 KB)
(Up to this point, some RO.ME specific features managed to get in the lib. The aim is to clean this up in next revisions.)
Face4
support to CollisionSystem
. (NINE78)Particle
support to Ray
. (mrdoob and jaycrossler)Ray.intersectObject
performance by checking boundingSphere first. (mrdoob)TrackballCamera
. (egraether)repeat
and offset
properties to Texture
. (mrdoob and alteredq)Vector2
, Vector3
and Vector4
. (egraether)2011 04 24 - r40 (263.774 KB, gzip: 64.320 KB)
Object3D.lookAt
. (mrdoob)CollisionSystem
. (drojdjou and alteredq)Trident
object. (sroucheray)data
object to Renderers for getting number of vertices/faces/callDraws from last render. (mrdoob)Projector
handling Particles with hierarchies. (mrdoob)2011 04 09 - r39 (249.048 KB, gzip: 61.020 KB)
Collisions
classes. (drojdjou)Sprite
object. (empaempa)*Loader
issue where Workers were kept alive and next loads were delayed. (alteredq)THREE
namespace to all the classes that missed it. (mrdoob)2011 03 31 - r38 (225.442 KB, gzip: 55.908 KB)
LensFlare
light. (empaempa)ShadowVolume
object (stencil shadows). (empaempa)Loader
(mrdoob)material.transparent
define whether material is transparent or not (before we were guessing). (mrdoob)2011 03 22 - r37 (208.495 KB, gzip: 51.376 KB)
Geometry.uvs
is now a multidimensional array (allowing infinite uv sets) (alteredq)CanvasRenderer
renders Face4
again (without spliting to 2 Face3
) (mrdoob)ParticleCircleMaterial
> ParticleCanvasMaterial
. Allowing injecting any canvas.context
code! (mrdoob)2011 03 14 - r36 (194.547 KB, gzip: 48.608 KB)
WebGLRenderer
aspect ratio bug when scene had only one material. (mrdoob)sizeAttenuation
property to ParticleBasicMaterial
. (mrdoob)PathCamera
. (alteredq)WebGLRenderer
bug when Camera has a parent. CameraCamera.updateMatrix
method. (empaempa)Camera.updateMatrix
method and Object3D.updateMatrix
. (mrdoob)2011 03 06 - r35 (187.875 KB, gzip: 46.433 KB)
translate
, translateX
, translateY
, translateZ
and lookAt
methods to Object3D
. (mrdoob)setViewport
and setScissor
to WebGLRenderer
. (alteredq)2011 03 02 - r34 (186.045 KB, gzip: 45.953 KB)
QuakeCamera
for easy fly-bys (alteredq)LOD
example (alteredq)2011 02 26 - r33 (184.483 KB, gzip: 45.580 KB)
ParticleSystem
object to WebGLRenderer
(alteredq)Line
support to WebGLRenderer
(alteredq)WebGLRenderer
(alteredq)Ribbon
object. (alteredq)WebGLRenderer
(alteredq)Sound
object and SoundRenderer
. (empaempa)LOD
, Bone
, SkinnedMesh
objects and hierarchy being developed. (empaempa)2010 12 31 - r32 (89.301 KB, gzip: 21.351 KB)
Scene
now supports Fog
and FogExp2
. WebGLRenderer
only right now. (alteredq)setClearColor( hex, opacity )
to WebGLRenderer
and CanvasRenderer
(alteredq & mrdoob)WebGLRenderer
shader system refactored improving performance. (alteredq)Projector
now does frustum culling of all the objects using their sphereBoundingBox. (thx errynp)material
property changed to materials
globaly.2010 12 06 - r31 (79.479 KB, gzip: 18.788 KB)
WebGLRenderer
python build.py --includes
generates includes string2010 11 30 - r30 (77.809 KB, gzip: 18.336 KB)
WebGLRenderer
(alteredq)SmoothShading
support on CanvasRenderer
/MeshLambertMaterial
MeshShaderMaterial
for WebGLRenderer
(alteredq)RenderableFace4
from Projector
/CanvasRenderer
(maybe just temporary).GeometryUtils
, ImageUtils
, SceneUtils
and ShaderUtils
(alteredq & mrdoob)2010 11 17 - r29 (69.563 KB)
CanvasRenderer
(julianwa)CanvasRenderer
and SVGRenderer
. (mrdoob)2010 11 04 - r28 (62.802 KB)
Loader
class allows load geometry asynchronously at runtime. (alteredq)MeshPhongMaterial
working with WebGLRenderer
. (alteredq)Projector.unprojectVector
and Ray
class to check intersections with faces (based on mindlapse work)Projector
z-sorting (not as jumpy anymore).2010 10 28 - r25 (54.480 KB)
WebGLRenderer
now up to date with other renderers! (alteredq)MeshFaceMaterial
(multipass per face)CanvasRenderer
and SVGRenderer
material handling2010 10 06 - r18 (44.420 KB)
PointLight
CanvasRenderer
and SVGRenderer
basic lighting support (ColorStroke/ColorFill only)Renderer
> Projector
. CanvasRenderer
, SVGRenderer
and DOMRenderer
do not extend anymorecomputeCentroids
method to Geometry
2010 09 17 - r17 (39.487 KB)
Light
, AmbientLight
and DirectionalLight
(philogb)WebGLRenderer
basic lighting support (philogb)2010 08 21 - r16 (35.592 KB)
Matrix4
and Vector3
methods2010 07 23 - r15 (32.440 KB)
UV
instead of Vector2
where it should be usedMesh.flipSided
boolean (false by default)CanvasRenderer
was handling UVs at 1,1 as bitmapWidth, bitmapHeight (instead of bitmapWidth - 1, bitmapHeight - 1)ParticleBitmapMaterial.offset
addedFace4
with MeshBitmapUVMappingMaterial
2010 07 17 - r14 (32.144 KB)
CanvasRenderer
(more duplicated code, but easier to handle)Face4
now supports MeshBitmapUVMappingMaterial
*StrokeMaterial
parameters. Now it's color
, opacity
, lineWidth
.BitmapUVMappingMaterial
> MeshBitmapUVMappingMaterial
ColorFillMaterial
> MeshColorFillMaterial
ColorStrokeMaterial
> MeshColorStrokeMaterial
FaceColorFillMaterial
> MeshFaceColorFillMaterial
FaceColorStrokeMaterial
> MeshFaceColorStrokeMaterial
ColorStrokeMaterial
> LineColorMaterial
Rectangle.instersects
returned false with rectangles with 0px witdh or height2010 07 12 - r13 (29.492 KB)
ParticleCircleMaterial
and ParticleBitmapMaterial
Particle
now use ParticleCircleMaterial
instead of ColorFillMaterial
Particle.size
> Particle.scale.x
and Particle.scale.y
Particle.rotation.z
for rotating the particleSVGRenderer
currently out of sync2010 07 07 - r12 (28.494 KB)
WebGLRenderer
(ColorFillMaterial
and FaceColorFillMaterial
by now)Matrix4.lookAt
fix (CanvasRenderer
and SVGRenderer
now handle the -Y)Color
now using 0-1 floats instead of 0-255 integers2010 07 03 - r11 (23.541 KB)
Scene.add
> Scene.addObject
Scene.removeObject
2010 06 22 - r10 (23.959 KB)
Object3D.overdraw = true
to enable CanvasRenderer screen space point expansion hack.2010 06 20 - r9 (23.753 KB)
autoClear
property for renderers.2010 06 06 - r8 (23.496 KB)
Geometry
.CanvasRenderer
expands screen space points (workaround for antialias gaps).CanvasRenderer
supports BitmapUVMappingMaterial
.2010 06 05 - r7 (22.387 KB)
2010 05 17 - r6 (21.003 KB)
CanvasRenderer
and SVGRenderer
clearRect
optimisations on CanvasRenderer
2010 05 16 - r5 (19.026 KB)
THREE
namespaceCamera.x
-> Camera.position.x
Camera.target.x
> Camera.target.position.x
ColorMaterial
> ColorFillMaterial
FaceColorMaterial
> FaceColorFillMaterial
ColorStrokeMaterial
and FaceColorStrokeMaterial
geometry.faces.a
are now indexes instead of references2010 04 26 - r4 (16.274 KB)
SVGRenderer
Particle renderingCanvasRenderer
uses context.setTransform
to avoid extra calculations2010 04 24 - r3 (16.392 KB)
Plane
and Cube
primitives2010 04 24 - r2 (15.724 KB)
Color
handling2010 04 24 - r1 (15.25 KB)
FAQs
JavaScript 3D library
The npm package three receives a total of 1,401,970 weekly downloads. As such, three popularity was classified as popular.
We found that three demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.