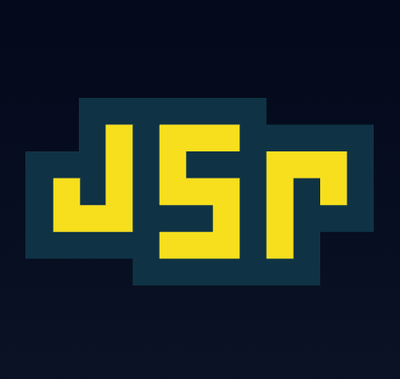
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The tmp npm package is used for creating temporary files and directories in a Node.js environment. It helps manage and clean up temporary files automatically.
Temporary File Creation
This feature allows you to create a temporary file. The library provides a callback with the path and file descriptor, and a cleanup callback to remove the file when it's no longer needed.
const tmp = require('tmp');
tmp.file(function _tempFileCreated(err, path, fd, cleanupCallback) {
if (err) throw err;
console.log('File: ', path);
console.log('Filedescriptor: ', fd);
// If we don't need the file anymore we could manually call the cleanupCallback
// But that is not necessary if we didn't pass the keep option because the library will clean after itself.
cleanupCallback();
});
Temporary Directory Creation
This feature allows you to create a temporary directory. Similar to temporary file creation, it provides a path to the directory and a cleanup callback.
const tmp = require('tmp');
tmp.dir(function _tempDirCreated(err, path, cleanupCallback) {
if (err) throw err;
console.log('Dir: ', path);
// Manual cleanup
cleanupCallback();
});
Synchronous File Creation
This feature allows for synchronous creation of a temporary file name. It returns the name directly without the need for a callback.
const tmp = require('tmp');
const name = tmp.tmpNameSync();
console.log('Temporary filename: ', name);
Synchronous Directory Creation
This feature allows for synchronous creation of a temporary directory. It returns an object with the directory name.
const tmp = require('tmp');
const dir = tmp.dirSync();
console.log('Temporary directory: ', dir.name);
The 'temp' package is similar to 'tmp' and is also used for managing temporary files and directories. It provides automatic cleanup and tracking of temporary files, but it has not been updated as frequently as 'tmp'.
The 'tempfile' package is a simpler alternative to 'tmp' that focuses on generating temporary file paths. It does not handle the creation or cleanup of the files.
The 'temp-dir' package provides the path to the system's default directory for temporary files, rather than creating temporary files or directories itself.
The 'mktemp' package creates temporary files and directories in a way similar to the Unix command of the same name. It offers a lower-level API compared to 'tmp' and requires manual cleanup.
A simple temporary file and directory creator for node.js.
The main difference between bruce's node-temp is that that mine more aggressively checks for the exitence of the newly created temporary file.
The API is slightly different, mine does not yet provide synchronous calls and all the parameters are optional. And you can even set whether you want to remove the temporary file on process exit or not.
npm install tmp
var tmp = require('tmp');
tmp.file(function _tempFileCreated(err, path, fd) {
if (err) throw err;
console.log("File: ", path);
console.log("Filedescriptor: ", fd);
});
var tmp = require('tmp');
tmp.dir(function _tempDirCreated(err, path) {
if (err) throw err;
console.log("Dir: ", path);
});
var tmp = require('tmp');
tmp.file({ mode: 0644, prefix: 'prefix-', postfix: '.txt' }, function _tempFileCreated(err, path, fd) {
if (err) throw err;
console.log("File: ", path);
console.log("Filedescriptor: ", fd);
});
tmp.dir({ mode: 0750, prefix: 'myTmpDir_' }, function _tempDirCreated(err, path) {
if (err) throw err;
console.log("Dir: ", path);
});
tmp.dir({ template: '/tmp/tmp-XXXXXX' }, function _tempDirCreated(err, path) {
iff (err) throw err;
console.log("Dir: ", path);
});
All options are optional :)
mode
: the file mode to create with it fallbacks to 0600
on file creation and 0700
on directory creationprefix
: the optional prefix, fallback to tmp-
if not providedpostfix
: the optional postfix, fallbacks to .tmp
on file creationtemplate
: mkstemps like filename template, no defaultdir
: the optional temporary directory, fallbacks to system default (guesses from environment)tries
: how many times should the function tries to get a unique filename before giving up, default 3
keep
: signals that the temporary file or directory should not be deleted on exit, default is to deleteFAQs
Temporary file and directory creator
The npm package tmp receives a total of 36,543,967 weekly downloads. As such, tmp popularity was classified as popular.
We found that tmp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.