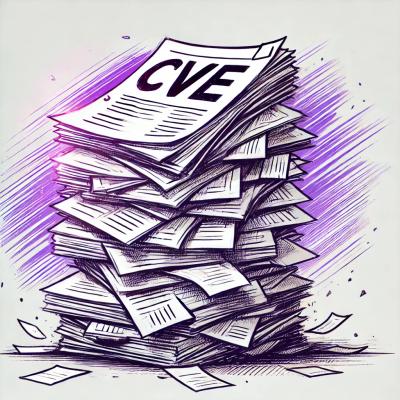
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
tonal-key
is a collection of functions to query about tonal keys.
This is part of tonal music theory library.
Example
// es6
import * as Key from "tonal-key"
// es5
const Key = require("tonal-key")
Example
Key.scale("E mixolydian") // => [ "E", "F#", "G#", "A", "B", "C#", "D" ]
Key.relative("minor", "C major") // => "A minor"
.degrees
⇒ Array
.modeNames(alias)
⇒ Array
.fromAlter(alt)
⇒ Key
.props(name)
⇒ Object
.scale(key)
⇒ Array
.alteredNotes(key)
⇒ Array
.leadsheetSymbols(symbols, keyName, [degrees])
⇒ function
.chords(name, [degrees])
⇒ Array.<string>
.triads(name, [degrees])
⇒ Array.<string>
.secDomChords(name)
⇒ Array
.relative(mode, key)
.tokenize(name)
⇒ Array
Key.degrees
⇒ Array
Get a list of key scale degrees in roman numerals
Kind: static constant of Key
Param | Type |
---|---|
keyName | string |
Example
Key.degrees("C major") => ["I", "ii", "iii", "IV", "V", "vi", "vii"]
Key.modeNames(alias)
⇒ Array
Get a list of valid mode names. The list of modes will be always in increasing order (ionian to locrian)
Kind: static method of Key
Returns: Array
- an array of strings
Param | Type | Description |
---|---|---|
alias | Boolean | true to get aliases names |
Example
Key.modes() // => [ "ionian", "dorian", "phrygian", "lydian",
// "mixolydian", "aeolian", "locrian" ]
Key.modes(true) // => [ "ionian", "dorian", "phrygian", "lydian",
// "mixolydian", "aeolian", "locrian", "major", "minor" ]
Key.fromAlter(alt)
⇒ Key
Create a major key from alterations
Kind: static method of Key
Returns: Key
- the key object
Param | Type | Description |
---|---|---|
alt | Integer | the alteration number (positive sharps, negative flats) |
Example
Key.fromAlter(2) // => "D major"
Key.props(name)
⇒ Object
Return the a key properties object with the following information:
Kind: static method of Key
Returns: Object
- the key properties object or null if not a valid key
Param | Type | Description |
---|---|---|
name | string | the key name |
Example
Key.props("C3 dorian") // => { tonic: "C", mode: "dorian", ... }
Key.scale(key)
⇒ Array
Get scale of a key
Kind: static method of Key
Returns: Array
- the key scale
Param | Type |
---|---|
key | string | Object |
Example
Key.scale("A major") // => [ "A", "B", "C#", "D", "E", "F#", "G#" ]
Key.scale("Bb minor") // => [ "Bb", "C", "Db", "Eb", "F", "Gb", "Ab" ]
Key.scale("C dorian") // => [ "C", "D", "Eb", "F", "G", "A", "Bb" ]
Key.scale("E mixolydian") // => [ "E", "F#", "G#", "A", "B", "C#", "D" ]
Key.alteredNotes(key)
⇒ Array
Get a list of the altered notes of a given Key. The notes will be in the same order than in the key signature.
Kind: static method of Key
Param | Type | Description |
---|---|---|
key | string | the key name |
Example
Key.alteredNotes("Eb major") // => [ "Bb", "Eb", "Ab" ]
Key.leadsheetSymbols(symbols, keyName, [degrees])
⇒ function
Get a lead-sheet symbols for a given key name
This function is currified (so can be partially applied)
From http://openmusictheory.com/triads.html
A lead-sheet symbol begins with a capital letter (and, if necessary, an accidental) denoting the root of the chord. That letter is followed by information about a chord’s quality:
Kind: static method of Key
See
Param | Type | Description |
---|---|---|
symbols | Array.<string> | an array of symbols in major scale order |
keyName | string | the name of the key you want the symbols for |
[degrees] | Array.<string> | the list of degrees. By default from 1 to 7 in ascending order |
Example
const chords = Key.leadsheetSymbols(["M", "m", "m", "M", "7", "m", "dim"])
chords("D dorian") //=> ["Dm", "Em", "FM", "G7", "Am", "Bdim", "CM"]
chords("D dorian", ['ii', 'V']) //=> [Em", "G7"]
Key.chords(name, [degrees])
⇒ Array.<string>
Get key seventh chords
Kind: static method of Key
Returns: Array.<string>
- seventh chord names
Param | Type | Description |
---|---|---|
name | string | the key name |
[degrees] | Array.<(number|string)> | can be numbers or roman numerals |
Example
Key.chords("A major") // => ["AMaj7", "Bm7", "C#m7", "DMaj7", ..,]
Key.chords("A major", ['I', 'IV', 'V']) // => ["AMaj7", "DMaj7", "E7"]
Key.chords("A major", [5, 4, 1]) // => ["E7", "DMaj7", AMaj7"]
Key.triads(name, [degrees])
⇒ Array.<string>
Get key triads
Kind: static method of Key
Returns: Array.<string>
- triad names
Param | Type | Description |
---|---|---|
name | string | the key name |
[degrees] | Array.<(string|number)> |
Example
Key.triads("A major") // => ["AM", "Bm", "C#m", "DM", "E7", "F#m", "G#mb5"]
Key.triads("A major", ['I', 'IV', 'V']) // => ["AMaj7", "DMaj7", "E7"]
Key.triads("A major", [1, 4, 5]) // => ["AMaj7", "DMaj7", "E7"]
Key.secDomChords(name)
⇒ Array
Get secondary dominant key chords
Kind: static method of Key
Param | Type | Description |
---|---|---|
name | string | the key name |
Example
Key.secDomChords("A major") // => ["E7", "F#7", ...]
Key.relative(mode, key)
Get relative of a key. Two keys are relative when the have the same key signature (for example C major and A minor)
It can be partially applied.
Kind: static method of Key
Param | Type | Description |
---|---|---|
mode | string | the relative destination |
key | string | the key source |
Example
Key.relative("dorian", "B major") // => "C# dorian"
// partial application
var minor = Key.relative("minor")
minor("C major") // => "A minor"
minor("E major") // => "C# minor"
Key.tokenize(name)
⇒ Array
Split the key name into its components (pitch class tonic and mode name)
Kind: static method of Key
Returns: Array
- an array in the form [tonic, key]
Param | Type |
---|---|
name | string |
Example
Key.tokenize("C major") // => ["C", "major"]
FAQs
Conversion between key numbers and note names
The npm package tonal-key receives a total of 735 weekly downloads. As such, tonal-key popularity was classified as not popular.
We found that tonal-key demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.