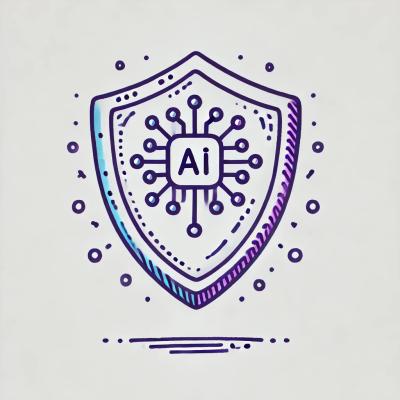
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
tonal-note
Advanced tools
tonal-note
is a collection of functions to manipulate musical notes in scientific notation
This is part of tonal music theory library.
import * as note from 'tonal-note'
// or var note = require('tonal-note')
note.name('bb2') // => 'Bb2'
note.chroma('bb2') // => 10
note.enharmonics('C#6') // => [ 'B##5', 'C#6', 'Db6' ]
note.simplify('B#3') // => 'C4'
// using ES6 import syntax
import { name } from 'tonal-note'
['c', 'db3', '2', 'g+', 'gx4'].map(name)
// => ['C', 'Db3', null, null, 'G##4']
.midi(note)
⇒ Integer
.fromMidi(midi, useSharps)
⇒ String
.freq(note)
⇒ Number
.chroma(note)
⇒ Integer
.name(n)
⇒ String
.note()
.props(note)
⇒ Object
.fromProps(noteProps)
⇒ String
.oct(note)
⇒ Integer
.step(note)
⇒ Integer
.pcFifths(note)
⇒ Integer
.alt(note)
⇒ Integer
.pc(n)
⇒ String
.enharmonics(note)
⇒ Array
.simplify(note)
⇒ String
note.midi(note)
⇒ Integer
Get the note midi number
(an alias of tonal-midi toMidi
function)
Kind: static method of note
Returns: Integer
- the midi number or null if not valid pitch
See: midi.toMidi
Param | Type | Description |
---|---|---|
note | Array | String | Number | the note to get the midi number from |
Example
note.midi('C4') // => 60
note.fromMidi(midi, useSharps)
⇒ String
Get the note name of a given midi note number
(an alias of tonal-midi note
function)
Kind: static method of note
Returns: String
- the note name
See: midi.note
Param | Type | Description |
---|---|---|
midi | Integer | the midi note number |
useSharps | Boolean | (Optional) set to true to use sharps instead of flats |
Example
note.fromMidi(60) // => 'C4'
note.freq(note)
⇒ Number
Get the frequency of a note
(an alias of the tonal-note package toFreq
function)
Kind: static method of note
Returns: Number
- the frequency
See: freq.toFreq
Param | Type | Description |
---|---|---|
note | Array | String | Number | the note to get the frequency |
Example
note.freq('A4') // => 440
note.chroma(note)
⇒ Integer
Return the chroma of a note. The chroma is the numeric equivalent to the pitch class, where 0 is C, 1 is C# or Db, 2 is D... 11 is B
Kind: static method of note
Returns: Integer
- the chroma
Param | Type |
---|---|
note | String | Pitch |
Example
var note = require('tonal-note')
note.chroma('Cb') // => 11
['C', 'D', 'E', 'F'].map(note.chroma) // => [0, 2, 4, 5]
note.name(n)
⇒ String
Given a note (as string or as array notation) returns a string with the note name in scientific notation or null if not valid note
Can be used to test if a string is a valid note name.
Kind: static method of note
Param | Type |
---|---|
n | Pitch | String |
Example
var note = require('tonal-note')
note.name('cb2') // => 'Cb2'
['c', 'db3', '2', 'g+', 'gx4'].map(note.name) // => ['C', 'Db3', null, null, 'G##4']
note.note()
Deprecated
Kind: static method of note
note.props(note)
⇒ Object
Deprecated
Kind: static method of note
Returns: Object
- the object with note properties or null if not valid note
Param | Type | Description |
---|---|---|
note | String | Pitch | the note |
Example
note.props('Db3') // => { step: 1, alt: -1, oct: 3 }
note.props('C#') // => { step: 0, alt: 1, oct: undefined }
note.fromProps(noteProps)
⇒ String
Deprecated
Kind: static method of note
Returns: String
- the note name
Param | Type | Description |
---|---|---|
noteProps | Object | an object with the following attributes: |
Example
note.fromProps({ step: 1, alt: -1, oct: 5 }) // => 'Db5'
note.fromProps({ step: 0, alt: 1 }) // => 'C#'
note.oct(note)
⇒ Integer
Get the octave of the given pitch
Kind: static method of note
Returns: Integer
- the octave, undefined if its a pitch class or null if
not a valid note
Param | Type | Description |
---|---|---|
note | String | Pitch | the note |
Example
note.oct('C#4') // => 4
note.oct('C') // => undefined
note.oct('blah') // => undefined
note.step(note)
⇒ Integer
Get the note step: a number equivalent of the note letter. 0 means C and
6 means B. This is different from chroma
(see example)
Kind: static method of note
Returns: Integer
- a number between 0 and 6 or null if not a note
Param | Type | Description |
---|---|---|
note | String | Pitch | the note |
Example
note.step('C') // => 0
note.step('Cb') // => 0
// usually what you need is chroma
note.chroma('Cb') // => 6
note.pcFifths(note)
⇒ Integer
Deprecated
Kind: static method of note
Returns: Integer
- the number of fifths to reach that pitch class from 'C'
Param | Type | Description |
---|---|---|
note | String | Pitch | the note (can be a pitch class) |
note.alt(note)
⇒ Integer
Get the note alteration: a number equivalent to the accidentals. 0 means no accidentals, negative numbers are for flats, positive for sharps
Kind: static method of note
Returns: Integer
- the alteration
Param | Type | Description |
---|---|---|
note | String | Pitch | the note |
Example
note.alt('C') // => 0
note.alt('C#') // => 1
note.alt('Cb') // => -1
note.pc(n)
⇒ String
Get pitch class of a note. The note can be a string or a pitch array.
Kind: static method of note
Returns: String
- the pitch class
Param | Type |
---|---|
n | String | Pitch |
Example
tonal.pc('Db3') // => 'Db'
tonal.map(tonal.pc, 'db3 bb6 fx2') // => [ 'Db', 'Bb', 'F##']
note.enharmonics(note)
⇒ Array
Get the enharmonics of a note. It returns an array of three elements: the below enharmonic, the note, and the upper enharmonic
Kind: static method of note
Returns: Array
- an array of pitches ordered by distance to the given one
Param | Type | Description |
---|---|---|
note | String | the note to get the enharmonics from |
Example
var note = require('tonal-note')
note.enharmonics('C') // => ['B#', 'C', 'Dbb']
note.enharmonics('A') // => ['G##', 'A', 'Bbb']
note.enharmonics('C#4') // => ['B##3', 'C#4' 'Db4']
note.enharmonics('Db') // => ['C#', 'Db', 'Ebbb'])
note.simplify(note)
⇒ String
Get a simpler enharmonic note name from a note if exists
Kind: static method of note
Returns: String
- the simplfiied note (if not found, return same note)
Param | Type | Description |
---|---|---|
note | String | the note to simplify |
Example
var note = require('tonal-note')
note.simplify('B#3') // => 'C4'
FAQs
Parse and manipulate music notes in scientific notation
The npm package tonal-note receives a total of 140 weekly downloads. As such, tonal-note popularity was classified as not popular.
We found that tonal-note demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.